(Haskell) Let us use the list [d1, d2, ..., dn], where each di is between 0 and 9, to represent the (positive) big-integer d1d2...dn. type BigInt = [Int] For example, [9, 9, 9, 9, 9, 9, 9, 9, 9, 8] represents the big-integer 9999999998. Fill out the implementation for bigAdd :: BigInt -> BigInt -> BigInt so that it takes two integer lists, where each integer is between 0 and 9 and returns the list corresponding to the addition of the two big-integers. Again, you have to fill in the implementation to supply the appropriate values to f, base, args. You should get the following behavior: ghci> bigAdd [9, 9] [1, 0, 0, 2] [1, 1, 0, 1] ghci> bigAdd [9, 9, 9, 9] [9, 9, 9] [1, 0, 9, 9, 8] You may find the integer functions div and mod to be helpful here. Your implementation should not be recursive. Note about BigInts: we expect the result of bigAdd to not have any leading zeroes, like [0, 1, 0, 9, 9, 8]. The number zero should be represented as []. Code: import Prelude hiding (replicate, sum) import Data.List (foldl') foldLeft :: (a -> b -> a) -> a -> [b] -> a foldLeft = foldl' -------------------------------------------------------------------------------- -- | `bigAdd n1 n2` returns the `BigInt` representing the sum of `n1` and `n2`. -- -- >>> bigAdd [9, 9] [1, 0, 0, 2] -- [1, 1, 0, 1] -- -- >>> bigAdd [9, 9, 9, 9] [9, 9, 9] -- [1, 0, 9, 9, 8] bigAdd :: BigInt -> BigInt -> BigInt bigAdd l1 l2 = removeZero res where (l1', l2') = padZero l1 l2 res = foldLeft f base args f a x = error "TBD:bigAdd:f" base = error "TBD:bigAdd:base" args = error "TBD:bigAdd:args"
(Haskell) Let us use the list [d1, d2, ..., dn], where each di is between 0 and 9, to represent the (positive) big-integer d1d2...dn.
For example, [9, 9, 9, 9, 9, 9, 9, 9, 9, 8] represents the big-integer 9999999998. Fill out the implementation for
so that it takes two integer lists, where each integer is between 0 and 9 and returns the list corresponding to the addition of the two big-integers. Again, you have to fill in the implementation to supply the appropriate values to f, base, args. You should get the following behavior:
You may find the integer functions div and mod to be helpful here.
Your implementation should not be recursive.
Note about BigInts: we expect the result of bigAdd to not have any leading zeroes, like [0, 1, 0, 9, 9, 8]. The number zero should be represented as [].
Code:
import Prelude hiding (replicate, sum)
import Data.List (foldl')
foldLeft :: (a -> b -> a) -> a -> [b] -> a
foldLeft = foldl'
--------------------------------------------------------------------------------
-- | `bigAdd n1 n2` returns the `BigInt` representing the sum of `n1` and `n2`.
--
-- >>> bigAdd [9, 9] [1, 0, 0, 2]
-- [1, 1, 0, 1]
--
-- >>> bigAdd [9, 9, 9, 9] [9, 9, 9]
-- [1, 0, 9, 9, 8]
bigAdd :: BigInt -> BigInt -> BigInt
bigAdd l1 l2 = removeZero res
where
(l1', l2') = padZero l1 l2
res = foldLeft f base args
f a x = error "TBD:bigAdd:f"
base = error "TBD:bigAdd:base"
args = error "TBD:bigAdd:args"
--------------------------------------------------------------------------------

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

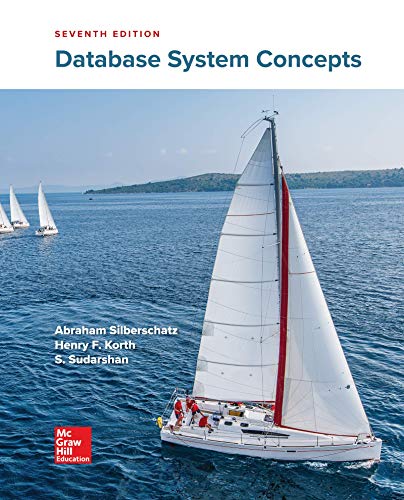
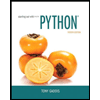
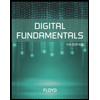
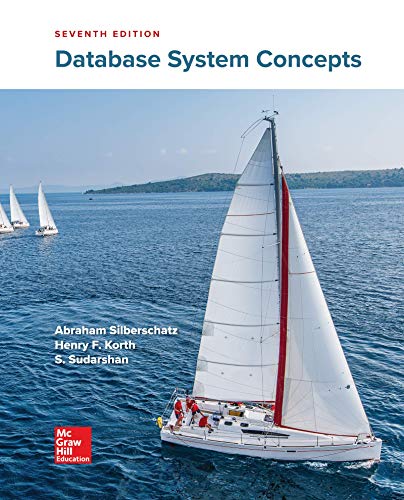
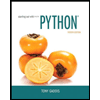
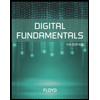
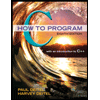
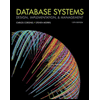
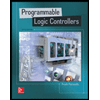