# Guess a number from 1 to 10 # Write the statements requested in Steps 1-6 below # See the examples in the provided code # Use structured programming and indent your code. # Programmer Name: *****add your name here**** import random # uses randrange instead of randint for better results in Python 3.7 # randrange stops just before the upper range, use (1, 11) for 1-10 num = random.randrange(1, 11) # Step 1: Ask the player to enter a name or quit to exit # Step 2: use a while statement to test when the name is not equal to quit # Step 3: input enter a number from 1 to 10 for the variable your_guess # display the number guessed print("Your number is", your_guess) while num != your_guess: # Step 4: Write an if statement for your_guess is less than num print("Your guess is too low.") your_guess = int(input("Guess another number from 1 to 10: ")) elif your_guess > num: print("Your guess is too high") your_guess = int(input("Guess another number from 1 to 10: ")) else: break print("The correct number was", num) # Step 5 display text with your guess and You won, name print("***************************************************************") # Step 6 ask the player to enter a name or quit to exit num = random.randrange(1, 11) print("Thank you for playing!")
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
# Guess a number from 1 to 10
# Write the statements requested in Steps 1-6 below
# See the examples in the provided code
# Use structured
# Programmer Name: *****add your name here**** import random
# uses randrange instead of randint for better results in Python 3.7
# randrange stops just before the upper range, use (1, 11) for 1-10 num = random.randrange(1, 11)
# Step 1: Ask the player to enter a name or quit to exit
# Step 2: use a while statement to test when the name is not equal to quit
# Step 3: input enter a number from 1 to 10 for the variable your_guess
# display the number guessed print("Your number is", your_guess) while num != your_guess:
# Step 4: Write an if statement for your_guess is less than num print("Your guess is too low.")
your_guess = int(input("Guess another number from 1 to 10: "))
elif your_guess > num: print("Your guess is too high")
your_guess = int(input("Guess another number from 1 to 10: "))
else: break print("The correct number was", num)
# Step 5 display text with your guess and You won, name print("***************************************************************")
# Step 6 ask the player to enter a name or quit to exit num = random.randrange(1, 11)
print("Thank you for playing!")

#guess a number from 1 to 10
#by C. calongne, 01/14/2019
#Pseudocode & python with Iteration M3Lab1_student.py
#guess a number from 1 to 10
#write the statements requested in step 1-6 below
#
#see the examples in provided code
#use structured programming and indent your code
#Programmer name ******add your name here********
import random
#uses randrange instead of randint for better results in python 3.7
#rand range stops just before the upper range, use (1, 11) for 1-10
num = random.randrange(1, 11)
#step1: Ask the player to enter a name or quit to exit
name = input("enter your name or quit to exit:\t")
#Step2: Use a while statement to test when the name is not equal to quit
while(name != "quit"):
#step3: input a number from 1 to 10 for the variable your_guess
your_guess = int(input("guess a number from 1 to 10:\t"))
#display the number guessed
print("Your number is :\t",your_guess)
while(num != your_guess):
#write an if statement for your_guess is less than num
if(your_guess < num):
print("Your guess is too low")
your_guess = int(input("guess another number from 1 to 10:\t"))
elif(your_guess > num):
print("your guess is too high")
your_guess = int(input("guess another number from 1 to 10:\t"))
else:
break
print("The correct name was:\t",num)
#step5: Display text with your guess and you won, name
print("Your guess was :\t",your_guess, "You won ",name)
print("**********************************************************")
#step6: ask the player to enter a name or exit
name = input("enter your name or quit to exit:\t")
print("Thank You")
Step by step
Solved in 2 steps with 2 images

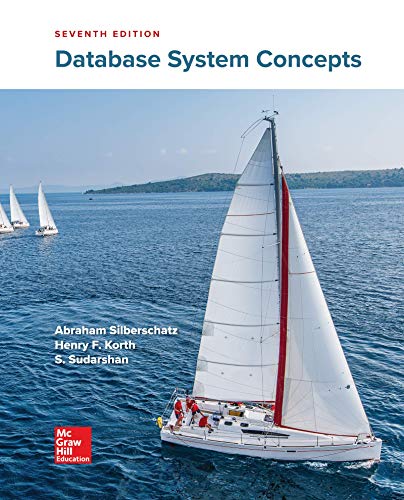
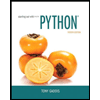
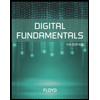
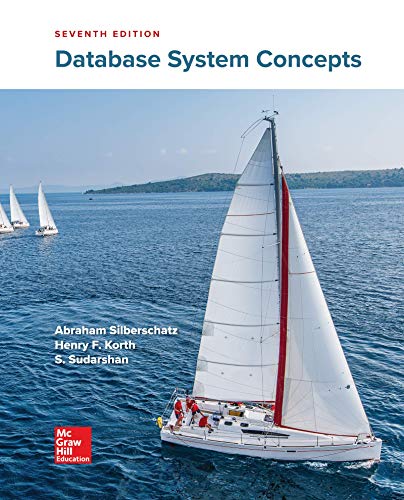
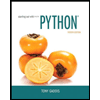
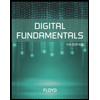
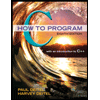
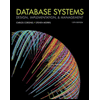
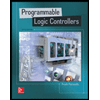