Given main() and the Instrument class, define a derived class, StringInstrument, for string instruments. Ex. If the input is: Drums Zildjian 2015 2500 Guitar Gibson 2002 1200 6 19 the output is: Instrument Information: Name: Drums Manufacturer: Zildjian Year built: 2015 Cost: 2500 Instrument Information: Name: Guitar Manufacturer: Gibson Year built: 2002 Cost: 1200 Number of strings: 6 Number of frets: 19
Given main() and the Instrument class, define a derived class, StringInstrument, for string instruments. Ex. If the input is: Drums Zildjian 2015 2500 Guitar Gibson 2002 1200 6 19 the output is: Instrument Information: Name: Drums Manufacturer: Zildjian Year built: 2015 Cost: 2500 Instrument Information: Name: Guitar Manufacturer: Gibson Year built: 2002 Cost: 1200 Number of strings: 6 Number of frets: 19
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
10.12 LAB: Instrument information
Given main() and the Instrument class, define a derived class, StringInstrument, for string instruments.
Ex. If the input is:
Drums Zildjian 2015 2500 Guitar Gibson 2002 1200 6 19the output is:
Instrument Information: Name: Drums Manufacturer: Zildjian Year built: 2015 Cost: 2500 Instrument Information: Name: Guitar Manufacturer: Gibson Year built: 2002 Cost: 1200 Number of strings: 6 Number of frets: 19
Transcribed Image Text:**Lab Activity: Instrument Information (10.12.1)**
**Current File: StringInstrument.cpp**
```cpp
#include "StringInstrument.h"
// TODO: Define mutator functions -
// SetNumOfStrings(), SetNumOfFrets()
// TODO: Define accessor functions -
// GetNumOfStrings(), GetNumOfFrets()
```
**Overview:**
This code is part of a lab activity focused on developing an understanding of mutator and accessor functions within a C++ class.
**Key Concepts:**
1. **Mutator Functions**: These functions are used to modify private data members of a class. In this example, you are tasked with defining:
- `SetNumOfStrings()`
- `SetNumOfFrets()`
2. **Accessor Functions**: These functions are used to retrieve the values of private data members. You need to define:
- `GetNumOfStrings()`
- `GetNumOfFrets()`
**Objective:**
Complete the TODO sections by implementing the specified functions to manage the properties of a string instrument, such as the number of strings and frets. This exercise will help solidify your grasp on encapsulation and data management within object-oriented programming.

Transcribed Image Text:```cpp
#ifndef STR_INSTRUMENTH
#define STR_INSTRUMENTH
#include "Instrument.h"
class StringInstrument : public Instrument {
// TODO: Declare private data members: numStrings, numFrets
// TODO: Declare mutator functions -
// SetNumOfStrings(), SetNumOfFrets()
// TODO: Declare accessor functions -
// GetNumOfStrings(), GetNumOfFrets()
};
#endif
```
### Explanation
This code snippet is a C++ header file named `StringInstrument.h` which defines a class for string instruments. The `StringInstrument` class publically inherits from the `Instrument` class. It is structured for enhancements, such as adding data members and accessor/mutator functions. Here's a breakdown:
- **Header Guards**:
- `#ifndef` and `#define` are used to prevent multiple inclusions of the same header file, which can cause errors during compilation.
- **Inheritance**:
- `class StringInstrument` inherits from the `Instrument` class, suggesting that it builds on the basic functionality of `Instrument`.
- **Placeholders**:
- Comments indicate placeholders for private data members `numStrings` and `numFrets`.
- Mutator functions `SetNumOfStrings()` and `SetNumOfFrets()` are intended for setting the values of these members.
- Accessor functions `GetNumOfStrings()` and `GetNumOfFrets()` are for retrieving the values.
The file is structured to implement additional functionality for managing characteristics specific to string instruments.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
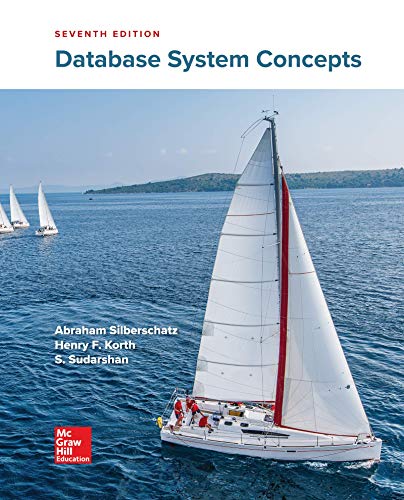
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
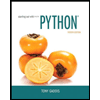
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
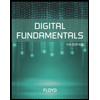
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
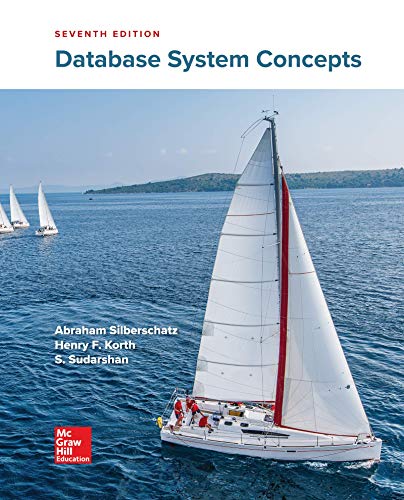
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
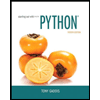
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
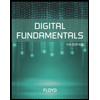
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
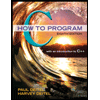
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
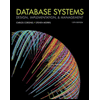
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
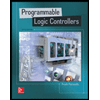
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education