Given main() and an IntNode class, complete the IntList class by writing the append() and search() methods. The search() method should return the IntNode whose data value matches a given key, and null if the key is not found. The search() method should also set the position of each IntNode searched in the IntList, starting with 1. Ex: If the input is: 12 23 59 37 923 2 -1 12 the output is: 12 found in list at position 1. If the input is: 12 23 59 37 923 2 -1 68 the output is: 68 not found in list. ______________________________________ import java.util.Scanner; public class SearchList { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); IntList intList = new IntList(); IntNode curNode, foundNode; int num, searchNum; num = scnr.nextInt(); while (num != -1) { // Insert into linked list curNode = new IntNode(num); intList.append(curNode); num = scnr.nextInt(); } searchNum = scnr.nextInt(); foundNode = intList.search(searchNum); if (foundNode != null) { System.out.println(searchNum + " found in list at position " + foundNode.nodePos + "."); } else { System.out.println(searchNum + " not found in list."); } } } ________________________________________ public class IntList { // Linked list nodes public IntNode headNode; public IntNode tailNode; public IntList() { // Front of nodes list headNode = null; tailNode = null; } // TODO: Write append method // prepend public void prepend(IntNode newNode) { if (headNode == null) { // list empty headNode = newNode; tailNode = newNode; } else { newNode.nextNode = headNode; headNode = newNode; } } // insertAfter public void insertAfter(IntNode curNode, IntNode newNode) { if (headNode == null) { // List empty headNode = newNode; tailNode = newNode; } else if (curNode == tailNode) { // Insert after tail tailNode.nextNode = newNode; tailNode = newNode; } else { newNode.nextNode = curNode.nextNode; curNode.nextNode = newNode; } } // TODO: Write search method that locates node with the same data value (dataVal) // as key and sets each node's position (nodePos) public void printIntList() { IntNode curNode; curNode = headNode; while (curNode != null) { curNode.printNodeData(); System.out.print(" "); curNode = curNode.nextNode; } } } ______________________________ public class IntNode { public int dataVal; public IntNode nextNode; // Reference to the next node public int nodePos; public IntNode() { dataVal = 0; nextNode = null; } // Constructor public IntNode(int dataInit) { this.dataVal = dataInit; this.nextNode = null; } // Constructor public IntNode(int dataInit, IntNode newNextNode) { this.dataVal = dataInit; this.nextNode = newNextNode; } public void printNodeData() { System.out.print(this.dataVal); } }
LAB: Finding an integer in a list (singly-linked list)
Given main() and an IntNode class, complete the IntList class by writing the append() and search() methods. The search() method should return the IntNode whose data value matches a given key, and null if the key is not found. The search() method should also set the position of each IntNode searched in the IntList, starting with 1.
Ex: If the input is:
12 23 59 37 923 2 -1 12the output is:
12 found in list at position 1.If the input is:
12 23 59 37 923 2 -1 68the output is:
68 not found in list.import java.util.Scanner;
public class SearchList {
public static void main (String[] args) {
Scanner scnr = new Scanner(System.in);
IntList intList = new IntList();
IntNode curNode, foundNode;
int num, searchNum;
num = scnr.nextInt();
while (num != -1) {
// Insert into linked list
curNode = new IntNode(num);
intList.append(curNode);
num = scnr.nextInt();
}
searchNum = scnr.nextInt();
foundNode = intList.search(searchNum);
if (foundNode != null) {
System.out.println(searchNum + " found in list at position " +
foundNode.nodePos + ".");
}
else {
System.out.println(searchNum + " not found in list.");
}
}
}
________________________________________
public class IntList {
// Linked list nodes
public IntNode headNode;
public IntNode tailNode;
public IntList() {
// Front of nodes list
headNode = null;
tailNode = null;
}
// TODO: Write append method
// prepend
public void prepend(IntNode newNode) {
if (headNode == null) { // list empty
headNode = newNode;
tailNode = newNode;
}
else {
newNode.nextNode = headNode;
headNode = newNode;
}
}
// insertAfter
public void insertAfter(IntNode curNode, IntNode newNode) {
if (headNode == null) { // List empty
headNode = newNode;
tailNode = newNode;
}
else if (curNode == tailNode) { // Insert after tail
tailNode.nextNode = newNode;
tailNode = newNode;
}
else {
newNode.nextNode = curNode.nextNode;
curNode.nextNode = newNode;
}
}
// TODO: Write search method that locates node with the same data value (dataVal)
// as key and sets each node's position (nodePos)
public void printIntList() {
IntNode curNode;
curNode = headNode;
while (curNode != null) {
curNode.printNodeData();
System.out.print(" ");
curNode = curNode.nextNode;
}
}
}
______________________________
public class IntNode {
public int dataVal;
public IntNode nextNode; // Reference to the next node
public int nodePos;
public IntNode() {
dataVal = 0;
nextNode = null;
}
// Constructor
public IntNode(int dataInit) {
this.dataVal = dataInit;
this.nextNode = null;
}
// Constructor
public IntNode(int dataInit, IntNode newNextNode) {
this.dataVal = dataInit;
this.nextNode = newNextNode;
}
public void printNodeData() {
System.out.print(this.dataVal);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

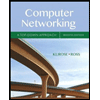
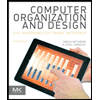
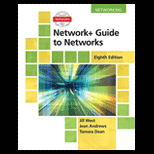
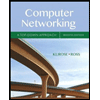
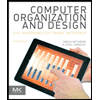
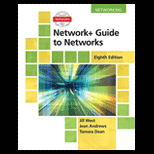
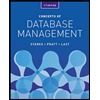
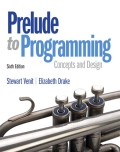
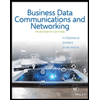