Given a weighted graph class and a PQInt class, please complete the implementation of the Dijkstras algorithm according to the instructions in the screenshot provided. Be done in python 3.10 or later, please class WeightedGraph(Graph) : """Weighted graph represented with adjacency lists.""" def __init__(self, v=10, edges=[], weights=[]) : """Initializes a weighted graph with a specified number of vertexes. Keyword arguments: v - number of vertexes edges - any iterable of ordered pairs indicating the edges weights - list of weights, same length as edges list """ super().__init__(v) for i, (u, v) in enumerate(edges): self.add_edge(u, v, weights[i]) def add_edge(self, a, b, w=1) : """Adds an edge to the graph. Keyword arguments: a - first end point b - second end point """ self._adj[a].add(b, w) self._adj[b].add(a, w) def get_edge_list(self, with_weights=False) : """Returns a list of the edges of the graph as a list of tuples. Default is of the form [ (a, b), (c, d), ... ] where a, b, c, d, etc are vertex ids. If with_weights is True, the generated list includes the weights in the following form [ ((a, b), w1), ((c, d), w2), ... ] where w1, w2, etc are the edge weights. Keyword arguments: with_weights -- True to include weights """ if not with_weights : return super().get_edge_list() else : return [ ((u,v),w) for u, uList in enumerate(self._adj) for v, w in uList.__iter__(True) if v > u] def mst_kruskal(self) : """Returns the set of edges in some minimum spanning tree (MST) of the graph, computed using Kruskal's algorithm. """ A = set() forest = DisjointIntegerSets(self.num_vertexes()) edges = self.get_edge_list(True) edges.sort(key=lambda x : x[1]) for (u,v), w in edges : if forest.findset(u) != forest.findset(v) : A.add((u,v)) forest.union(u,v) return A def mst_prim(self, r=0) : """Returns the set of edges in some minimum spanning tree (MST) of the graph, computed using Prim's algorithm. Keyword arguments: r - vertex id to designate as the root (default is 0). """ parent = [ None for x in range(self.num_vertexes())] Q = PQInts(self.num_vertexes()) Q.insert(r, 0) for u in range(self.num_vertexes()) : if u != r : Q.insert(u, math.inf) while not Q.is_empt
Given a weighted graph class and a PQInt class, please complete the implementation of the Dijkstras
class WeightedGraph(Graph) :
"""Weighted graph represented with adjacency lists."""
def __init__(self, v=10, edges=[], weights=[]) :
"""Initializes a weighted graph with a specified number of vertexes.
Keyword arguments:
v - number of vertexes
edges - any iterable of ordered pairs indicating the edges
weights - list of weights, same length as edges list
"""
super().__init__(v)
for i, (u, v) in enumerate(edges):
self.add_edge(u, v, weights[i])
def add_edge(self, a, b, w=1) :
"""Adds an edge to the graph.
Keyword arguments:
a - first end point
b - second end point
"""
self._adj[a].add(b, w)
self._adj[b].add(a, w)
def get_edge_list(self, with_weights=False) :
"""Returns a list of the edges of the graph
as a list of tuples. Default is of the form
[ (a, b), (c, d), ... ] where a, b, c, d, etc are
vertex ids. If with_weights is True, the generated
list includes the weights in the following form
[ ((a, b), w1), ((c, d), w2), ... ] where w1, w2, etc
are the edge weights.
Keyword arguments:
with_weights -- True to include weights
"""
if not with_weights :
return super().get_edge_list()
else :
return [ ((u,v),w) for u, uList in enumerate(self._adj) for v, w in uList.__iter__(True) if v > u]
def mst_kruskal(self) :
"""Returns the set of edges in some
minimum spanning tree (MST) of the graph,
computed using Kruskal's algorithm.
"""
A = set()
forest = DisjointIntegerSets(self.num_vertexes())
edges = self.get_edge_list(True)
edges.sort(key=lambda x : x[1])
for (u,v), w in edges :
if forest.findset(u) != forest.findset(v) :
A.add((u,v))
forest.union(u,v)
return A
def mst_prim(self, r=0) :
"""Returns the set of edges in some
minimum spanning tree (MST) of the graph,
computed using Prim's algorithm.
Keyword arguments:
r - vertex id to designate as the root (default is 0).
"""
parent = [ None for x in range(self.num_vertexes())]
Q = PQInts(self.num_vertexes())
Q.insert(r, 0)
for u in range(self.num_vertexes()) :
if u != r :
Q.insert(u, math.inf)
while not Q.is_empty() :
u = Q.extract_min()
for v, w in self._adj[u].__iter__(True) :
if Q.contains(v) and w < Q.get_priority(v) :
parent[v] = u
Q.change_priority(v, w)
return { (u,v) for v, u in enumerate(parent) if u != None}

![class PQInts :
_____slots_____ = ['_minheap', '_index' ]
def _init__(self, n) :
"""Initializes an empty PQ, but configured to support
integers in the interval [0,n) as the elements."""
self._index = [ -1 for x in range (n) ]
self._minheap = []
def size (self):
"""Size of the PQ."ww
return len (self._minheap)
def is empty (self):
"""Returns True if PQ is empty and False otherwise. "ww
return len (self._minheap) == 0
def insert (self, element, value) :
"""Adds an element to the PQ with a specified priority.
Adds the element to the PQ provided PQ doesn't already contain it.
Does nothing if the PQ already contains the element.
Returns True if element added and False if already present.
Keyword arguments:
element - The element to add.
value -- The priority of the element.
111111
if self._index [element] >= 0 :
return False
position = len (self._minheap)
self._minheap.append((element, value))
self._percolate_up (position)
return True
def insert_all (self, pairs) :
"""Adds a list of (element, value) pairs to the PQ.
Adds the (element, value) pairs from the list pairs to the PQ. Only the
pairs for which element is not already in the PQ are added.
Keyword arguments:
pairs -- A list of 2-tuples of the form (element, value) where value is the priority of element.
111111
if len (pairs) >= len (self._minheap) :
for p in pairs :
if self._index [p[0]] < 0:
self._minheap.append (p)
self._heapify()
for el, val in pairs :
self.insert (el, val)
else :](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0d59b49e-3987-421f-888e-fd2b8b6a0cc6%2Fa3c9d892-b1bc-41b4-a8f9-824a1b61a7d5%2F4pprgmu_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

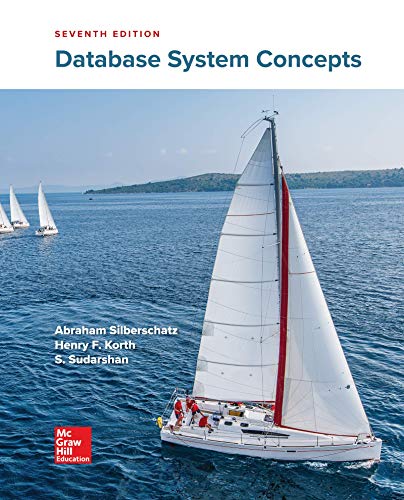
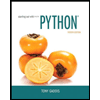
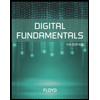
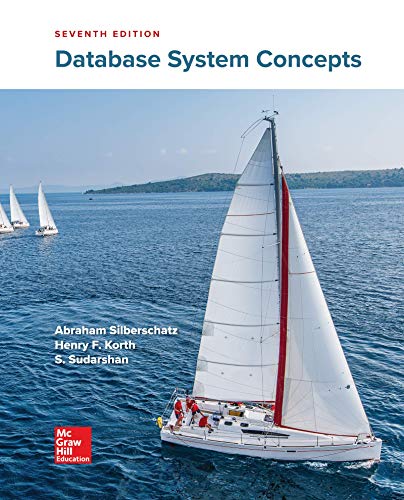
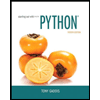
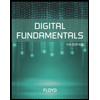
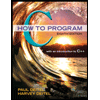
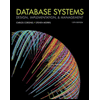
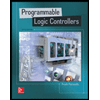