Given a Scanner reference variable named input that has been associated with an input source consisting of a sequence of lines, write the code necessary to read in every line and print them all out on a single line, separated by a space. Hint: Use input.nextLine() to read a line and use input.hasNextLine() to test if the input has a next line available to read.
Given a Scanner reference variable named input that has been associated with an input source consisting of a sequence of lines, write the code necessary to read in every line and print them all out on a single line, separated by a space. Hint: Use input.nextLine() to read a line and use input.hasNextLine() to test if the input has a next line available to read.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please write in Java code

Transcribed Image Text:### Reading and Printing a Sequence of Lines with a Scanner in Java
**Problem Statement:**
Given a `Scanner` reference variable named `input` that has been associated with an input source consisting of a sequence of lines, write the code necessary to read in every line and print them all out on a single line, separated by a space.
**Hint:**
- Use `input.nextLine()` to read a line.
- Use `input.hasNextLine()` to test if the input has a next line available to read.
### Sample Solution
Here is a sample solution to address the given problem:
```java
StringBuilder result = new StringBuilder();
while (input.hasNextLine()) {
String line = input.nextLine();
if (result.length() == 0) {
result.append(line); // No leading space for the first line
} else {
result.append(" ").append(line); // Space before subsequent lines
}
}
System.out.println(result.toString());
```
### Explanation:
1. **StringBuilder Initialization:**
- We initialize a `StringBuilder` named `result` to efficiently concatenate strings.
2. **While Loop with `input.hasNextLine()`:**
- We use a `while` loop that continues as long as there is another line to read (`input.hasNextLine()` returns `true`).
3. **Reading and Appending Lines:**
- Inside the loop, `input.nextLine()` reads the current line.
- If `result` is empty (for the first iteration), append the line without a leading space.
- For subsequent lines, append a space before appending the line to ensure they are separated by a space.
4. **Printing the Result:**
- Finally, we print the concatenated result using `System.out.println(result.toString())`.
This solution ensures that all the lines are read and printed on a single line, separated by spaces, effectively solving the given task using the Java `Scanner` class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
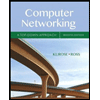
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
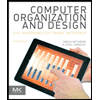
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
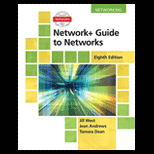
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
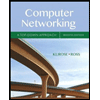
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
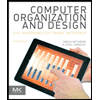
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
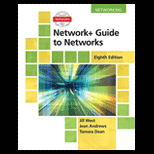
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
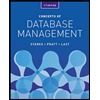
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
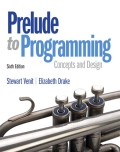
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
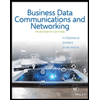
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY