Given a problem and correct (shuffled) program, re-arrange the shuffled code segments in the correct order. ------------------------------------------ ATM machines are nowadays more in use, people are easily able to withdraw money from the ATM, instead of rushing to the bank and waiting in a long queue to get money. But there are people who don't know, how to use ATM machines and they are not aware of the money withdrawal range. Write a c++ program to help them using the Exception handling mechanism. Pin number should be of 4 digits, an exception should be thrown if the user enters more than 4 digits(refer to sample input and output) The maximum amount that can be withdrawn is Rs.20000, an exception should be thrown if the user enters more than Rs.20000(refer to sample input and output.) Consider a class named Atm with the following data members Data type Variable name int pin double amount The class Atm has the following constructor and method Member Function Description Atm(int pin,double amount) Sets the values passed by parameters. void display() This method displays the success message. Input and Output Format: Refer sample input and output for formatting specifications [All text in bold corresponds to input and the rest corresponds to output] Sample Input and Output 1: Welcome to IndusLand Bank ATM Enter your pin number 12345 Exception: Pin number should be of 4 digit Enter your valid pin number 1234 Enter the transaction amount 30000 Exception: Transaction amount exceeded, the limit is Rs.20000 Enter the transaction amount again 20000 Transaction successful, visit again Sample Input and Output 2: Welcome to IndusLand Bank ATM Enter your pin number 4556 Enter the transaction amount 19000 Transaction successful, visit again ------------------------Question ends-------------------------------- the shuffled program given below don't change or add anything in it just rearrange below code . ------------------------------------------- #include #include #include #include using namespace std; class Atm { int pin; double amount; cout<<"Welcome to IndusLand Bank ATM"<>amount; } throw "Exception: Pin number should be of 4 digit"; } } catch(const char* msg) { cerr << msg << endl; cin>>pin; if(!(pin >=1000 && pin<=9999)) { // Main function for the program int main( ) { cout <<"Enter your valid pin number"<>pin; } } Atm(int pin,double amount) { try { cout <<"Enter the transaction amount"<pin = pin; this->amount = amount; } Atm a(pin,amount); a.display(); return 0; cin>>amount; if(amount>20000) {
Given a problem and correct (shuffled) program, re-arrange the shuffled code segments in the correct order.
Pin number should be of 4 digits, an exception should be thrown if the user enters more than 4 digits(refer to sample input and output)
The maximum amount that can be withdrawn is Rs.20000, an exception should be thrown if the user enters more than Rs.20000(refer to sample input and output.)
Consider a class named Atm with the following data members
Data type | Variable name |
int | pin |
double | amount |
The class Atm has the following constructor and method
Member Function | Description |
Atm(int pin,double amount) | Sets the values passed by parameters. |
void display() | This method displays the success message. |
Input and Output Format:
Refer sample input and output for formatting specifications
[All text in bold corresponds to input and the rest corresponds to output]
Sample Input and Output 1:
Welcome to IndusLand Bank ATM
Enter your pin number
12345
Exception: Pin number should be of 4 digit
Enter your valid pin number
1234
Enter the transaction amount
30000
Exception: Transaction amount exceeded, the limit is Rs.20000
Enter the transaction amount again
20000
Transaction successful, visit again
Sample Input and Output 2:
Welcome to IndusLand Bank ATM
Enter your pin number
4556
Enter the transaction amount
19000
Transaction successful, visit again
------------------------Question ends--------------------------------
the shuffled program given below don't change or add anything in it just rearrange below code .
-------------------------------------------
#include<iostream>
#include<cmath> #include <iomanip> #include <cstring>
using namespace std; class Atm { int pin;
double amount; cout<<"Welcome to IndusLand Bank ATM"<<endl; public:
Atm() { public:
int pin; double amount; }
public:
void display() { try
{ cout <<"Enter your pin number"<<endl; throw "Exception: Transaction amount exceeded, the limit is Rs.20000";
} } cout<<"Transaction successful, visit again"<<endl;
} }; cout <<"Enter the transaction amount again"<<endl;
cin>>amount; } throw "Exception: Pin number should be of 4 digit";
} } catch(const char* msg)
{ cerr << msg << endl; cin>>pin;
if(!(pin >=1000 && pin<=9999)) { // Main function for the program
int main( ) { cout <<"Enter your valid pin number"<<endl;
cin>>pin; } }
Atm(int pin,double amount) { try
{ cout <<"Enter the transaction amount"<<endl; catch(const char* msg)
{ cerr << msg << endl; this->pin = pin;
this->amount = amount; } Atm a(pin,amount);
a.display(); return 0; cin>>amount;
if(amount>20000) { |

Step by step
Solved in 2 steps

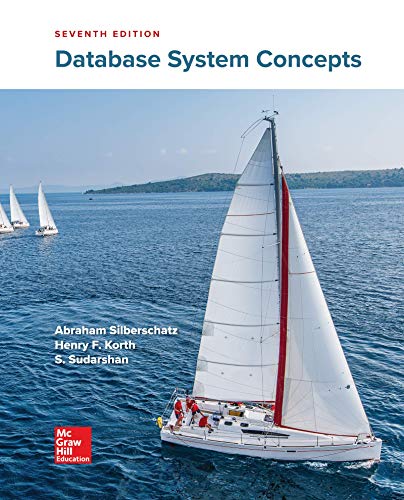
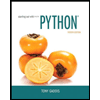
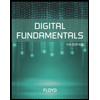
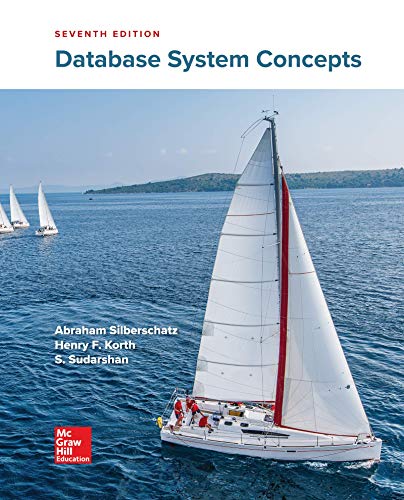
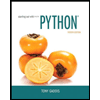
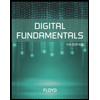
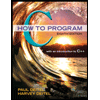
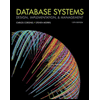
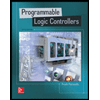