Given a budget (type long) and a list of prices represented as entries of a container of type vector, find out how many items on the price_list you can afford at most. This is the return value of the function. Here is its prototype: int get_most_items(std::vectorprice_list, long budget) To solve this homework, it is a good idea to first sort the price_list in ascending order. For sorting in ascending order, you can use the function std::sort(). It can be called as std::sort(v.begin(), v.end()) .Note that there may be items on the price list whose prices are negative to make the problem a bit more interesting. If the size of price_list is zero, the function returns 0. All necessary libraries have been included. All numbers are expressed in Cents to avoid rounding errors. Here is a simple example: std::vector price_list = { 10, 199, 1}; long budget = 11; int no_items = get_most_items(price_list, budget); The variable no_items will then have the value 2 as two items can be afforded from the price list given the available budget. main.cpp: #include #include "shopping.h" int main(int argc, char **argv) { std::cout << "Please enter positive integer size of vector: "; std::vector price_list; size_t vector_size{ 0 }; std::cin >> vector_size; std::cout << "You entered " << vector_size << " as the size of the vector." << std::endl; std::cout << "\nEnter integer entries followd by Return (vector is called price_list):" << std::endl; long price{ 0 }; for (size_t i = 0; i < vector_size; i++) { std::cout << "price_list[" << i << "]: "; std::cin >> price; price_list.push_back(price); } std::cout << "Please enter positive value for your budget: "; long budget; std::cin >> budget; int no_items = get_most_items(price_list, budget); std::cout << "You were able to afford " << no_items << " items." << std::endl; return 0; }
Given a budget (type long) and a list of prices represented as entries of a container of type
int get_most_items(std::vector<long>price_list, long budget)
To solve this homework, it is a good idea to first sort the price_list in ascending order. For sorting in ascending order, you can use the function std::sort(). It can be called as std::sort(v.begin(), v.end()) .Note that there may be items on the price list whose prices are negative to make the problem a bit more interesting.
If the size of price_list is zero, the function returns 0.
All necessary libraries have been included. All numbers are expressed in Cents to avoid rounding errors.std::vector<long> price_list = { 10, 199, 1};
The variable no_items will then have the value 2 as two items can be afforded from the price list given the available budget.
#include <iostream>
#include "shopping.h"
int main(int argc, char **argv)
{
std::cout << "Please enter positive integer size of vector: ";
std::vector<long> price_list;
size_t vector_size{ 0 };
std::cin >> vector_size;
std::cout << "You entered " << vector_size << " as the size of the vector." << std::endl;
std::cout << "\nEnter integer entries followd by Return (vector is called price_list):" << std::endl;
long price{ 0 };
for (size_t i = 0; i < vector_size; i++)
{
std::cout << "price_list[" << i << "]: ";
std::cin >> price;
price_list.push_back(price);
}
std::cout << "Please enter positive value for your budget: ";
long budget;
std::cin >> budget;
int no_items = get_most_items(price_list, budget);
std::cout << "You were able to afford " << no_items << " items." << std::endl;
return 0;
}

Step by step
Solved in 4 steps with 3 images

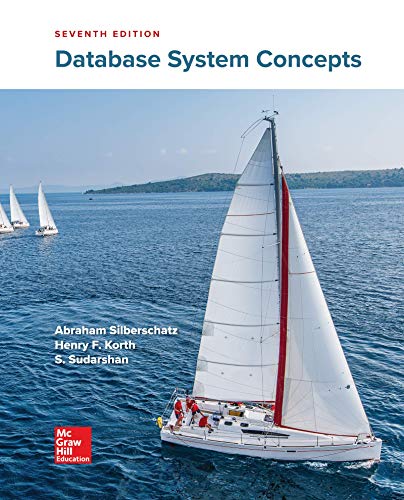
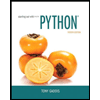
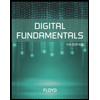
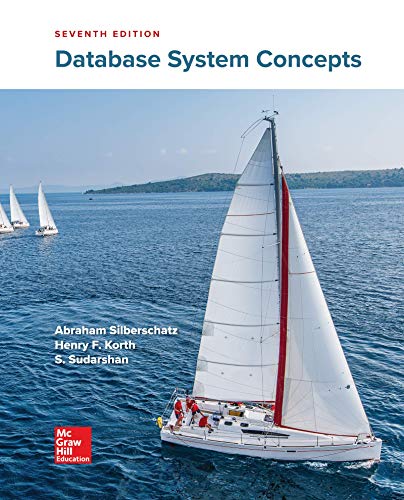
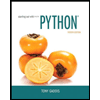
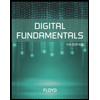
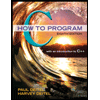
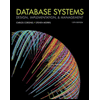
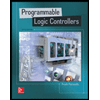