Give a set of nested loops to print a multiplication table in the following format. (Your spacing does not have to be perfect, but you should add some type of blank space between values as shown below).
Give a set of nested loops to print a multiplication table in the following format. (Your spacing does not have to be perfect, but you should add some type of blank space between values as shown below).
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help writing this in Java

Transcribed Image Text:### Creating a Multiplication Table Using Nested Loops
To create a multiplication table like the one shown below, you can use nested loops in your code. Your spacing does not need to be perfectly aligned, but it is essential to add some blank space between the values for clarity.
Here is the multiplication table for numbers 1 through 10:
```
1 2 3 4 5 6 7 8 9 10
2 4 6 8 10 12 14 16 18 20
3 6 9 12 15 18 21 24 27 30
4 8 12 16 20 24 28 32 36 40
5 10 15 20 25 30 35 40 45 50
6 12 18 24 30 36 42 48 54 60
7 14 21 28 35 42 49 56 63 70
8 16 24 32 40 48 56 64 72 80
9 18 27 36 45 54 63 72 81 90
```
### Explanation of the Table
Each number in the table is the product of the row number and the column number. For example, the value at row 3, column 4, is \(3 \times 4 = 12\).
### Example Code Snippet
Here is a sample code snippet in Python that uses nested loops to generate this multiplication table:
```python
# Define the range for the multiplication table
range_limit = 10
for i in range(1, range_limit + 1):
for j in range(1, range_limit + 1):
print(f"{i * j:2}", end=" ")
print()
```
### Detailed Explanation of the Code
1. **Range Definition**: `range_limit` is set to 10 to create a 10x10 multiplication table.
2. **Outer Loop**: The outer loop iterates through each row, starting from 1 to 10.
3. **Inner Loop**: The inner loop iterates through each column, starting from 1 to 10.
4. **Multiplication and Printing
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
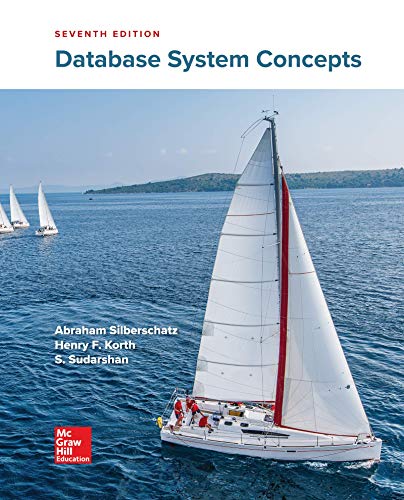
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
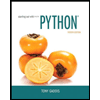
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
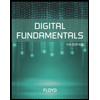
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
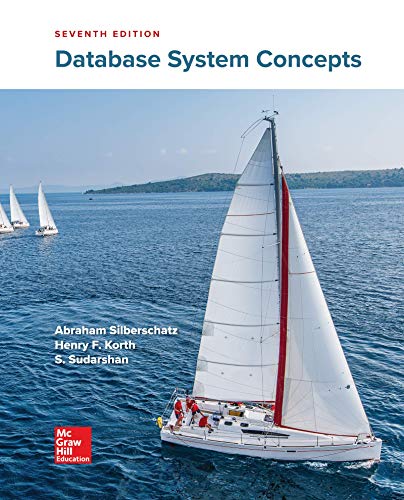
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
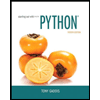
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
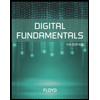
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
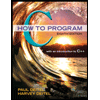
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
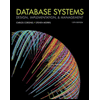
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
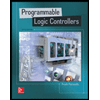
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education