generate
Chapter8: Arrays
Section: Chapter Questions
Problem 9PE
Related questions
Question
Complete the following functions to generate different sequences of numbers:
![Complete the following functions to generate different sequences of numbers:
int* Fibonacci(int n)
• Create an array of integers and size n
• Fill in the array with the first n Fibonacci numbers. The Fibonacci sequence begins with O and then 1 follows. All subsequent
values are the sum of the previous two, ex: 0, 1, 1, 2, 3, 5, 8, 13.
• Return the array
int" Squares (int n)
• Create an array of integers and size n
• Fill in the array with the squares of 1 to n (inclusive). Ex: 1, 4, 9, ..., n²
• Return the array
int* Concatenate (int* array¹, int sizel, int* array2, int size2)
• Create an array of integers and size = size1 + size2
• Fill in the array with the elements of array1 followed by the elements of array2
• Return the array
main() reads the size of the Fibonacci and the squares sequences and calls the three functions above to create the arrays. Then main()
calls PrintArray() provided in the template to print the arrays.
Ex: If the input is:
64
the output is:
0 1 1 2 3 5
1 4 9 16
0 1 1 2 3 5 1 4 9 16
1 #include <stdio.h>
2 #include <stdlib.h>
3
4 void PrintArray (int* array, int size) {
5 for (int j = 0; j < size; ++i) {
printf("%d ", array[j]);
}
6
7
8}
9
10 // Return the first n Fibonacci numbers
11 // fibonacci(0) = 0, fibonacci(1) = 1, fibonacci (2) = 1
12 // Ex: n = 5, seq = 0 1 1 2 3
13 int* Fibonacci(int n) {
14
15
16
17
18
19
20 }
21
int* seq;
int i;
/* Type your code here. 3/
return seq;
22 // Return sequence of squares for 1..n (inclusive)
23 // Ex: sqrn = 3, seq = 149
24 int* Squares (int n) {
25
int* seq;
26
27
28
29
30 }
31
/* Type your code here. */
return seq;
32 // Return an array that is a copy of array1 followed by
33 // the elements of array2
34 int* Concatenate(int* array1, int sizel, int* array2, int size2) {
35 int j;
36
37
int* seq;
38
2256&9&ARODB%
40
41}
46
43 int main(void) {
44
47
48
49
50
51
53
54
55
56
57
58
59
60
61
/* Type your code here. */
62
63
64
65
66
67
68}
69
return seq;
int fibn;
| || | || |
|| |
int sqrn;
scanf("%d %d", &fibn, &sqrn);
int* fibs;
int* sqrs;
int* conc;
fibs Fibonacci(fibn);
PrintArray (fibs, fibn);
printf("\n");
sqrs Squares (sqrn);
PrintArray (sqrs, sqrn);
printf("\n");
// sea of first fibn Fibonacci numbers
// Ex: fibn = 5, seq = 0 1 1 2 3
// number of squares starting with 1
// Ex: sqrn = 3, seq = 149
conc = Concatenate (fibs, fibn, sqrs, sqrn);
PrintArray (conc, fibn + sqrn);
printf("\n");
return 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5779ee7a-d811-43a6-ba75-7d41285b789d%2F8e3570b4-39bc-493d-953d-fb4f9f48dc67%2F4q0oh1q_processed.png&w=3840&q=75)
Transcribed Image Text:Complete the following functions to generate different sequences of numbers:
int* Fibonacci(int n)
• Create an array of integers and size n
• Fill in the array with the first n Fibonacci numbers. The Fibonacci sequence begins with O and then 1 follows. All subsequent
values are the sum of the previous two, ex: 0, 1, 1, 2, 3, 5, 8, 13.
• Return the array
int" Squares (int n)
• Create an array of integers and size n
• Fill in the array with the squares of 1 to n (inclusive). Ex: 1, 4, 9, ..., n²
• Return the array
int* Concatenate (int* array¹, int sizel, int* array2, int size2)
• Create an array of integers and size = size1 + size2
• Fill in the array with the elements of array1 followed by the elements of array2
• Return the array
main() reads the size of the Fibonacci and the squares sequences and calls the three functions above to create the arrays. Then main()
calls PrintArray() provided in the template to print the arrays.
Ex: If the input is:
64
the output is:
0 1 1 2 3 5
1 4 9 16
0 1 1 2 3 5 1 4 9 16
1 #include <stdio.h>
2 #include <stdlib.h>
3
4 void PrintArray (int* array, int size) {
5 for (int j = 0; j < size; ++i) {
printf("%d ", array[j]);
}
6
7
8}
9
10 // Return the first n Fibonacci numbers
11 // fibonacci(0) = 0, fibonacci(1) = 1, fibonacci (2) = 1
12 // Ex: n = 5, seq = 0 1 1 2 3
13 int* Fibonacci(int n) {
14
15
16
17
18
19
20 }
21
int* seq;
int i;
/* Type your code here. 3/
return seq;
22 // Return sequence of squares for 1..n (inclusive)
23 // Ex: sqrn = 3, seq = 149
24 int* Squares (int n) {
25
int* seq;
26
27
28
29
30 }
31
/* Type your code here. */
return seq;
32 // Return an array that is a copy of array1 followed by
33 // the elements of array2
34 int* Concatenate(int* array1, int sizel, int* array2, int size2) {
35 int j;
36
37
int* seq;
38
2256&9&ARODB%
40
41}
46
43 int main(void) {
44
47
48
49
50
51
53
54
55
56
57
58
59
60
61
/* Type your code here. */
62
63
64
65
66
67
68}
69
return seq;
int fibn;
| || | || |
|| |
int sqrn;
scanf("%d %d", &fibn, &sqrn);
int* fibs;
int* sqrs;
int* conc;
fibs Fibonacci(fibn);
PrintArray (fibs, fibn);
printf("\n");
sqrs Squares (sqrn);
PrintArray (sqrs, sqrn);
printf("\n");
// sea of first fibn Fibonacci numbers
// Ex: fibn = 5, seq = 0 1 1 2 3
// number of squares starting with 1
// Ex: sqrn = 3, seq = 149
conc = Concatenate (fibs, fibn, sqrs, sqrn);
PrintArray (conc, fibn + sqrn);
printf("\n");
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
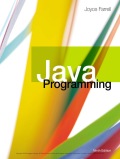
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
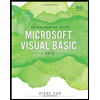
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
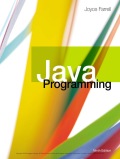
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
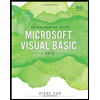
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning