f(x) = sin Optimization: make the output as big or as small as possible 1 0.24 +1.42 +2.9z+1.5,

![import math
import numpy as np
import matplotlib.pyplot as plt
matplotlib inline
def f(x):
return 10*np.log(1/((pow (x, 2)+1))) *math.exp(-(pow(x, 2)+x))
def plot_curve (numPoints, a,b):
X = np.linspace(a, b, numPoints)
Y = np.zeros (numPoints)
for i in range (0, numPoints):
f(x[i])
Y[i]
plt.figure(figsize=(8,16))
=
plt.plot(X,Y)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34c2a7c6-20d8-45e7-8c90-b1c224068cda%2F1463e017-12cf-4020-9e8d-4e6d86dd30da%2Fprvn92b_processed.jpeg&w=3840&q=75)

Step1/4
import math
def f(x):
a = (0.2*x)**4+(1.4*x)**2+2.9*x+1.5
return math.sin(1/a)
def d_f(x):
return -(10*(8*x**3+28*x+29)*math.cos(1/(x**4/5+(7*x**2)/5+(29*x)/10+3/2)))/(2*x**4+14*x**2+29*x+15)**2
Explanation:
f(x) is the function given in the question
d_f(x) is the derivative of the function given in the question
Step2/4
Your newton method
a = 0.5
tol = 10**-15
defect = abs(f(a))
count = 0
while defect > tol and count<100:
a = a-f(a)/d_f(a)
defect = abs(f(a))
count = count + 1
print()
print(a)
print(f(a))
print(count)
print()
Generated out for this function
563145.6947875244
6.2143676404312025e-21
9
Explanation: Please refer to solution in this step. Step3/4
Your code for bisection method
import time
import math
tol = 10**-15
a = 0.5
b = 1.5
Error = (b-a)/2
c = math.nan
count = 0
tic = time.time()
if f(a)*f(b) < 0:
print(1)
while (Error>tol) and (count<1000):
print(2)
c = (a+b)/2
if f(a)*f(c) < 0:
b = c
elif f(b)*f(c) < 0:
a = c
Error = (b - a)/2
count = count + 1
toc = time.time()
runTime = toc - tic
print()
print(c)
print(f(c))
print(runTime)
print(count)
print()
Generated output,
nan
nan
0.0
0
Explanation:
Such a out is arriving because the values of a=0.5 and b=1.5 are not bound to roots
Step4/4
Alternatively another bisection function from python opensource code,
import numpy as np
def my_bisection(f, a, b, tol):
# approximates a root, R, of f bounded
# by a and b to within tolerance
# | f(m) | < tol with m the midpoint
# between a and b Recursive implementation
# check if a and b bound a root
if np.sign(f(a)) == np.sign(f(b)):
raise Exception(
"The scalars a and b do not bound a root")
# get midpoint
m = (a + b)/2
if np.abs(f(m)) < tol:
# stopping condition, report m as root
return m
elif np.sign(f(a)) == np.sign(f(m)):
# case where m is an improvement on a.
# Make recursive call with a = m
return my_bisection(f, m, b, tol)
elif np.sign(f(b)) == np.sign(f(m)):
# case where m is an improvement on b.
# Make recursive call with b = m
return my_bisection(f, a, m, tol)
r1 = my_bisection(f, 0, 2, 0.1)
print("r1 =", r1)
r01 = my_bisection(f, 0, 2, 0.01)
print("r01 =", r01)
print("f(r1) =", f(r1))
print("f(r01) =", f(r01))
Explanation:
Its clearly indicating the below msg for the given function and the values of a and b,
Exception: The scalars a and b do not bound a root
Step by step
Solved in 2 steps

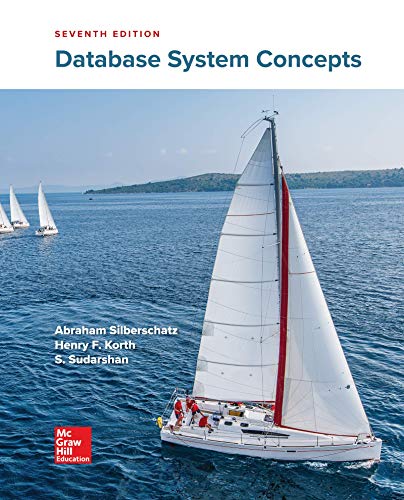
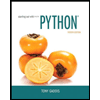
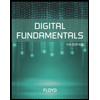
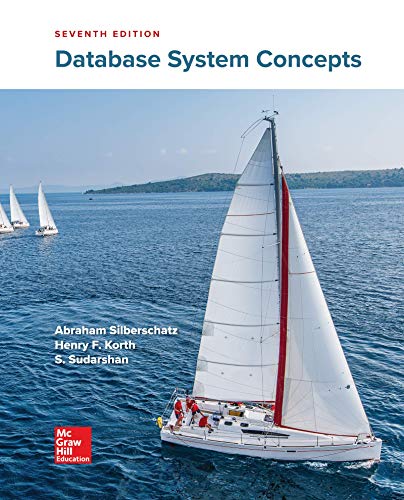
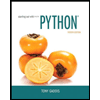
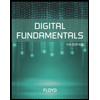
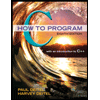
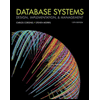
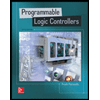