Fun math practice You Python programming teacher wants to see you make a program that accepts input for a name and a few numbers from the user, and then display some fun output using some of the math and built in functions you have learned to use in python.



#Program to enter name and few number and display some fun outputs using math built in function
import math
#define main function
def main():
#display welcome messsage
print("----------------------------------------")
print("Welcome to my fun math program!")
print("----------------------------------------")
#prompt user to enter name
name = input("\nFirst what is your name? ")
#display message, ask user to enter three numbers
print(f"\nHello {name}. Next I am going to ask for three numbers and show you some fun math using them")
#prompt user to enter three numbers
first_num = int(input("\nEnter first number and make sure it is a whole number: "))
second_num = int(input("\nEnter second number and make sure it is a whole number with three digit: "))
third_num = float(input("\nEnter third number and make sure it is a real number with two decimal places: "))
print(f"\nOkay {name}, you are ready to see some random math...")
#display all math operations
print(f"\n1. {first_num} squared is {first_num*first_num}")
print(f"2. The square root of {second_num} is {math.sqrt(second_num)}")
print(f"3. {third_num} times pi is {third_num*math.pi}")
print(f"4. {first_num} can be displayed as real number like this {float(first_num)}")
print(f"5. {third_num} can be displayed rounded to 1 decimal places like this", "{:.1f}".format(third_num))
print(f"6. The integer value of {third_num} is {int(third_num)}")
#calling main program
main()
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

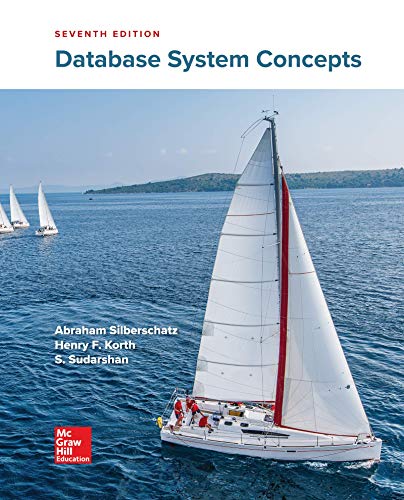
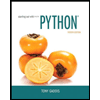
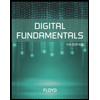
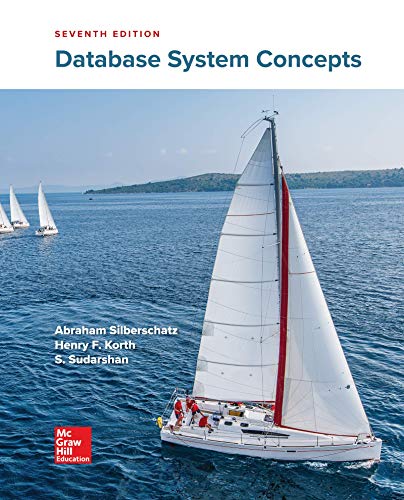
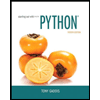
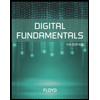
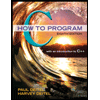
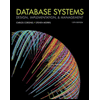
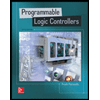