For this c++ homework fill in the code Use functions and function declarations (headers) to calculate the payment schedule for a loan. Read from the user: initialLoan, annualInterest, MonthlyPayment Create a loan payment function based on the 3 params above. For each month, print on one line, for each month#: Month, Balance, Payment, PaidOnBalance, Interest, Total Int. Paid Until the monthbalance (currentBalance)<=0 At the end of every year (12 months), draw a line of 65 '$' characters. If the MonthlyPayment < 1st month's monthlyInterest, reject the payment code: #include <iostream>using namespace std; // make a header here for drawline // make a function called printLoanPayments that// takes in the loanAmount, annualInterest, and monthly payment// (each of the above are doubles) // print a header for multiple columns// convert annualInterest to monthly interest // keep updating the current balance// keep updating the total Interest paid// calculate the current month's 'interest accrued' (owed)// calculate the amount of the loan balance that is 'paid down'.// This is the amount that is paid toward the balance.// Note: monthly payment = AmountPaidTowardBalance + interestAccrued. // each month (starting at 1), print on a single line:// Month Balance Payment PaidOnBalance Interest and Total Int. Paid// until the Balance is 0 // Reject a monthly payment that is not able to cover at least the first// month's interest. // Remember to print so that dollars and cents are shown // Copy your drawLine function here int main(){ double loanAmount, annualInterest, monthlyPayment; cout << "What is the loan amount? "; cin >> loanAmount; cout << "What is the annual interest? Type answer as a decimal (.1 for 10%, etc) "; cin >> annualInterest; cout << "What is the monthly payment? "; cin >> monthlyPayment; // add a call to printLoanPayments here return 0;}
For this c++ homework fill in the code
Use functions and function declarations (headers) to calculate the payment schedule for a loan.
Read from the user: initialLoan, annualInterest, MonthlyPayment
Create a loan payment function based on the 3 params above.
- For each month, print on one line, for each month#:
Month, Balance, Payment, PaidOnBalance, Interest, Total Int. Paid
Until the monthbalance (currentBalance)<=0
- At the end of every year (12 months), draw a line of 65 '$' characters.
- If the MonthlyPayment < 1st month's monthlyInterest, reject the payment
code:
#include <iostream>
using namespace std;
// make a header here for drawline
// make a function called printLoanPayments that
// takes in the loanAmount, annualInterest, and monthly payment
// (each of the above are doubles)
// print a header for multiple columns
// convert annualInterest to monthly interest
// keep updating the current balance
// keep updating the total Interest paid
// calculate the current month's 'interest accrued' (owed)
// calculate the amount of the loan balance that is 'paid down'.
// This is the amount that is paid toward the balance.
// Note: monthly payment = AmountPaidTowardBalance + interestAccrued.
// each month (starting at 1), print on a single line:
// Month Balance Payment PaidOnBalance Interest and Total Int. Paid
// until the Balance is 0
// Reject a monthly payment that is not able to cover at least the first
// month's interest.
// Remember to print so that dollars and cents are shown
// Copy your drawLine function here
int main()
{
double loanAmount, annualInterest, monthlyPayment;
cout << "What is the loan amount? ";
cin >> loanAmount;
cout << "What is the annual interest? Type answer as a decimal (.1 for 10%, etc) ";
cin >> annualInterest;
cout << "What is the monthly payment? ";
cin >> monthlyPayment;
// add a call to printLoanPayments here
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

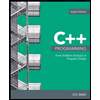
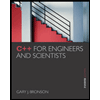
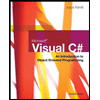
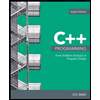
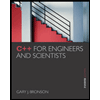
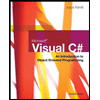
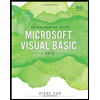
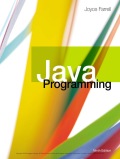
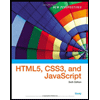