Create a program which will plot the “uneclipsed” area of the star as a function of time - just like our analytical solution for the square star/planet - starting at the time between when the planet first starts to overlap the star to when the whole planet has passed in front of the star. You will need variables for the velocity of the planet, the side lengths of the star and planet. For the velocity of the planet use an arbitrary number such as 0.001 (in Jupiters/second). For now, don’t worry about a realistic velocity of the planet. You will need arrays for the area and the time that has passed. Hint: the time array can be initialized with a list of times like earlier in this handout and the area array can be empty. You will need to loop over time, and use logic statements to determine what expression from the analytical solution you will need to use for the “uneclipsed” area. Hint: you can then add the area at each time to the area array with area.append(uneclipsedArea) Finally, you will need to plot the data you just generated! If the brightness is proportional to the area, what would you use to plot the transit lightcurve shape from previous lessons?
Create a program which will plot the “uneclipsed” area of the star as a function of time - just like our analytical solution for the square star/planet - starting at the time between when the planet first starts to overlap the star to when the whole planet has passed in front of the star.
You will need variables for the velocity of the planet, the side lengths of the star and planet. For the velocity of the planet use an arbitrary number such as 0.001 (in Jupiters/second). For now, don’t worry about a realistic velocity of the planet.
You will need arrays for the area and the time that has passed. Hint: the time array can be initialized with a list of times like earlier in this handout and the area array can be empty.
You will need to loop over time, and use logic statements to determine what expression from the analytical solution you will need to use for the “uneclipsed” area. Hint: you can then add the area at each time to the area array with area.append(uneclipsedArea)
Finally, you will need to plot the data you just generated! If the brightness is proportional to the area, what would you use to plot the transit lightcurve shape from previous lessons?

Here's an example Python program that plots the "uneclipsed" area of a square planet passing in front of a square star as a function of time. The program assumes that the planet is moving at a constant velocity of 0.001 Jupiters/second and that the side lengths of the star and planet are 2 and 1, respectively (in arbitrary units).
import numpy as np
import matplotlib.pyplot as plt
# Define constants
v_planet = 0.001 # Jupiters/second
a_star = 2.0 # units
a_planet = 1.0 # units
# Initialize time array
t = np.linspace(0, 20, 1000) # seconds
# Initialize area array
area = []
# Loop over time and calculate uneclipsed area
for time in t:
x_planet = v_planet * time # position of planet's leading edge
x_overlap = x_planet - a_planet # position of overlap
if x_overlap < -a_star:
# planet hasn't reached star yet
uneclipsed_area = a_planet**2
elif x_overlap < a_star:
# planet partially overlaps star
uneclipsed_area = a_planet**2 - (a_star + x_overlap)**2
else:
# planet has passed star
uneclipsed_area = a_planet**2 - 4*a_star**2
area.append(uneclipsed_area)
# Plot uneclipsed area as a function of time
plt.plot(t, area)
plt.xlabel('Time (seconds)')
plt.ylabel('Uneclipsed area')
plt.show()
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

for the transit lightcurve I get an error message:
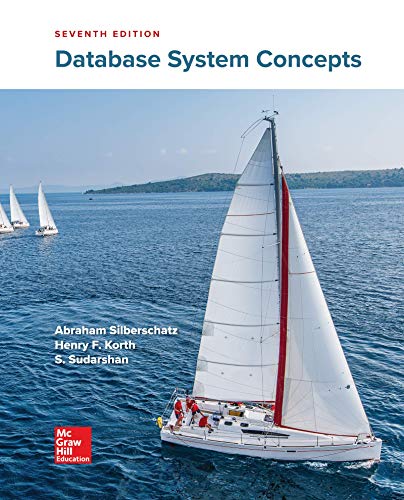
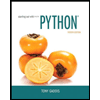
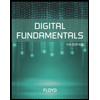
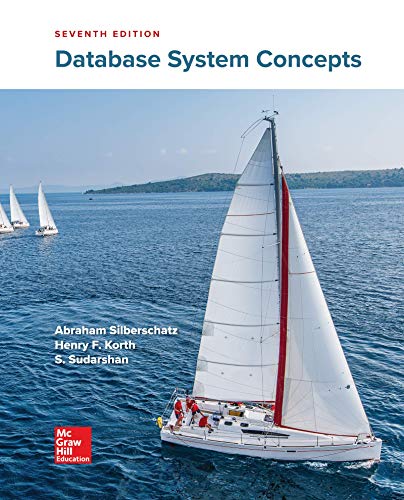
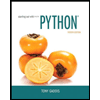
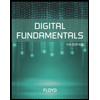
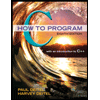
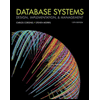
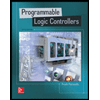