For simplicity, we'll assume that only the three "base" logical connectors: conjunction, disjunction, and negation (A, V, -), are allowed in the statements given, since we've shown that implications (→) and biconditionals (+) can be simplified into combinations of the above. This turns out to be a very helpful simplification, because it means that we can take advantage of Python's built in boolean logic operators (and, or, and not). wwww
For simplicity, we'll assume that only the three "base" logical connectors: conjunction, disjunction, and negation (A, V, -), are allowed in the statements given, since we've shown that implications (→) and biconditionals (+) can be simplified into combinations of the above. This turns out to be a very helpful simplification, because it means that we can take advantage of Python's built in boolean logic operators (and, or, and not). wwww
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:For simplicity, we'll assume that only the three "base" logical connectors: conjunction,
disjunction, and negation (A, V, ¬), are allowed in the statements given, since we've shown that
implications (→) and biconditionals (+) can be simplified into combinations of the above. This
turns out to be a very helpful simplification, because it means that we can take advantage of
Python's built in boolean logic operators (and, or, and not).
You're welcome to try a different approach, but a simple way to get Python to compute the "truth
table" for a proposition like (P A Q) is to follow these steps:
1. Express the proposition as a string in Python: '-(P A Q)'
2. Replace each 'A' with 'and', each 'V' with 'or', and each '' with 'not '. Be careful about
spacing: the negation symbol - is generally not written with any space between it and
the expression it's negating, but the Python not operator will need a space. With our
example string, we'd now have 'not (P and Q)'
3. For any given row of the truth table, replace each variable with the corresponding truth
value ('True' or 'False'). For example, if you were looking at the P = True, Q = False
row, the example string would now be 'not (True and False)'
4. Use eval(), a built-in Python function, to evaluate the string as though it was Python
code, which should return either True or False.
5. Check that you get the same result for both propositions, across every combination of
inputs. For the example above, we'd need to check four possibilities: P = True and Q =
True, P = True and Q = False, P = False and Q = True, P = False and Q = False. Note
that the number of combinations grows exponentially with the number of propositional
variables, so this approach definitely doesn't scale well, but it will work for simple
propositions.
![The file contains one function, logically equivalent (prop1, prop2, var list), that you
will need to complete in order to receive credit for the assignment. The function takes in two
strings representing propositions, and a list of all of the propositional variables present (these
will be single, capital letters). The function should return the boolean value True if the two
propositions are logically equivalent, or False otherwise.
You can assume that the proposition strings only contain the following components:
Propositional variables: capital letters like P, Q, R, etc. You can assume that T and F
will not be used to avoid complications when replacing the variables with True/False.
Operators (A, v, -). You can assume that there will be a space between the A and v
operators and their operands, but not the - and its single operand.
Parentheses ( ) to force order of operations
You can write any number of helper functions in addition to the above function.
Examples:
>>> logically.equivalent ('-¬P', 'P', ['P'])
True
>>> logicallyequivalent('¬(P A Q)', '-P V -Q', ['P', 'Q'])
True
>>> logically.equivalent ('Q v -(P V -Q) ', '-P v Q', ['P', 'Q'])
False
>>> logically..equivalent ('PA Q', 'Q A R', ['P', 'Q', 'R'])
False
>>> logicallyequivalent('-(s v ¬R) V (P A ¬(R A -P))',
'(P V (P V (R A ¬R)) V -S) A (P V R)',
['P', 'R', 'S'])
True
Hints:
The trickiest part of this is trying every possible combination of True/False for the
propositional variables. One approach is to try using a recursive helper function: at each
step you recursively try both possibilities for a single variable, and only return True if
both those branches return True.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F96f2d7be-9b3e-4031-8282-ea2192f8de5b%2F836f319e-af22-416f-869a-ac1e47d25d30%2Fz8dis9b_processed.jpeg&w=3840&q=75)
Transcribed Image Text:The file contains one function, logically equivalent (prop1, prop2, var list), that you
will need to complete in order to receive credit for the assignment. The function takes in two
strings representing propositions, and a list of all of the propositional variables present (these
will be single, capital letters). The function should return the boolean value True if the two
propositions are logically equivalent, or False otherwise.
You can assume that the proposition strings only contain the following components:
Propositional variables: capital letters like P, Q, R, etc. You can assume that T and F
will not be used to avoid complications when replacing the variables with True/False.
Operators (A, v, -). You can assume that there will be a space between the A and v
operators and their operands, but not the - and its single operand.
Parentheses ( ) to force order of operations
You can write any number of helper functions in addition to the above function.
Examples:
>>> logically.equivalent ('-¬P', 'P', ['P'])
True
>>> logicallyequivalent('¬(P A Q)', '-P V -Q', ['P', 'Q'])
True
>>> logically.equivalent ('Q v -(P V -Q) ', '-P v Q', ['P', 'Q'])
False
>>> logically..equivalent ('PA Q', 'Q A R', ['P', 'Q', 'R'])
False
>>> logicallyequivalent('-(s v ¬R) V (P A ¬(R A -P))',
'(P V (P V (R A ¬R)) V -S) A (P V R)',
['P', 'R', 'S'])
True
Hints:
The trickiest part of this is trying every possible combination of True/False for the
propositional variables. One approach is to try using a recursive helper function: at each
step you recursively try both possibilities for a single variable, and only return True if
both those branches return True.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
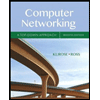
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
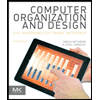
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
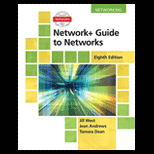
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
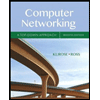
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
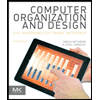
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
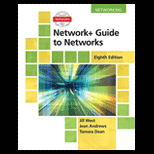
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
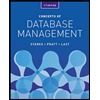
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
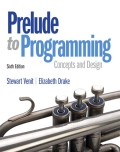
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
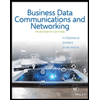
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY