for c++ please List Reverse Modify the linked list class you created in the previous programming challenges by adding a member function named reverse that rearranges the nodes in the list so that their order is reversed. Demonstrate the function in a simple driver program. here is my first programming #include using namespace std; struct node { int data; node *next; }; class list { private: node *head,*tail; public: list() { head = NULL; tail = NULL; } ~list() { } void append() { int value; cout<<"Enter the value to append: "; cin>>value; node *temp=new node; node *s; temp->data = value; temp->next = NULL; s = head; if(head==NULL) head=temp; else { while (s->next != NULL) s = s->next; s->next = temp; } } void insert() { int value,pos,i,count=0; cout<<"Enter the value to be inserted: "; cin>>value; node *temp=new node; node *s, *ptr; temp->data = value; temp->next = NULL; cout<<"Enter the postion at which node to be inserted: "; cin>>pos; s = head; while (s!=NULL) { s = s->next; count++; } if (pos==1) { if(head==NULL) { head=temp; head->next=NULL; } else { ptr=head; head=temp; head->next=ptr; } } else if(pos>1 && pos<=count) { s=head; for(i=1;inext; } ptr->next=temp; temp->next=s; } else cout<<"Positon out of range"<>pos; node *s,*ptr; s=head; if(pos==1) head=s->next; else { while(s!=NULL) { s=s->next; count++; } if(pos>0 && pos<=count) { s=head; for(i=1;inext; } ptr->next=s->next; } else cout<<"Position out of range"<next!=NULL) { cout<data<<"->"; temp = temp->next; } cout<data<
for c++ please
List Reverse
Modify the linked list class you created in the previous programming challenges by
adding a member function named reverse that rearranges the nodes in the list so that
their order is reversed. Demonstrate the function in a simple driver program.
here is my first programming
#include <iostream>
using namespace std;
struct node
{
int data;
node *next;
};
class list
{
private:
node *head,*tail;
public:
list()
{
head = NULL;
tail = NULL;
}
~list()
{
}
void append()
{
int value;
cout<<"Enter the value to append: ";
cin>>value;
node *temp=new node;
node *s;
temp->data = value;
temp->next = NULL;
s = head;
if(head==NULL)
head=temp;
else
{
while (s->next != NULL)
s = s->next;
s->next = temp;
}
}
void insert()
{
int value,pos,i,count=0;
cout<<"Enter the value to be inserted: ";
cin>>value;
node *temp=new node;
node *s, *ptr;
temp->data = value;
temp->next = NULL;
cout<<"Enter the postion at which node to be inserted: ";
cin>>pos;
s = head;
while (s!=NULL)
{
s = s->next;
count++;
}
if (pos==1)
{
if(head==NULL)
{
head=temp;
head->next=NULL;
}
else
{
ptr=head;
head=temp;
head->next=ptr;
}
}
else if(pos>1 && pos<=count)
{
s=head;
for(i=1;i<pos;i++)
{
ptr=s;
s=s->next;
}
ptr->next=temp;
temp->next=s;
}
else
cout<<"Positon out of range"<<endl;
}
void deleting()
{
int pos,i,count=0;
if (head==NULL)
{
cout<<"List is empty"<<endl;
return;
}
cout<<"Enter the position of value to be deleted: ";
cin>>pos;
node *s,*ptr;
s=head;
if(pos==1)
head=s->next;
else
{
while(s!=NULL)
{
s=s->next;
count++;
}
if(pos>0 && pos<=count)
{
s=head;
for(i=1;i<pos;i++)
{
ptr=s;
s=s->next;
}
ptr->next=s->next;
}
else
cout<<"Position out of range"<<endl;
free(s);
cout<<"Element Deleted"<<endl;
}
}
void display()
{
node *temp;
if(head==NULL)
{
cout<<"The List is Empty"<<endl;
return;
}
temp=head;
cout<<"Elements of list are: "<<endl;
while(temp->next!=NULL)
{
cout<<temp->data<<"->";
temp = temp->next;
}
cout<<temp->data<<endl;
}
};
int main()
{
list s;
s.append();
s.append();
s.append();
s.append();
s.display();
s.insert();
s.display();
s.append();
s.display();
s.deleting();
s.display();
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

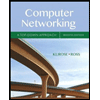
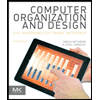
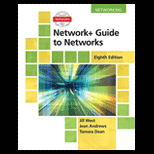
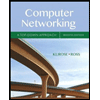
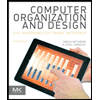
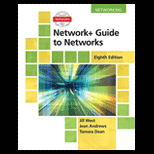
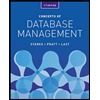
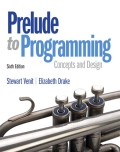
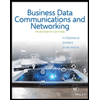