Floor F1 2 3 4 5 Rooms Occupied 20 14 70.0 Occupancy Rate Room Price Revenue $ 2365.86 % $ 168.99 33 18 54.5 % $ 152.99 $ 2753.82 33 12 36.4 % $ 152.99 $ 1835.88 33 15 45.5 % $ 152.99 $ 2294.85 16 8 50.0 % $ 254.99 $ 2039.92 ---jGRASP: operation complete. Floor Rooms Occupied Occupancy Rate Room Price 1 20 14 70.0% 2 33 18 54.5% 3 33 12 36.4% $ 168.99 $ 152.99 $ 152.99 Revenue $ 2,365.86 $ 2,753.82 4 33 15 45.5% $ 152.99 $ 1,835.88 $ 2,294.85 5 16 8 50.0% $ 254.99 $ 2,039.92
//Main.java
public class Main {
public static void main(String[] args) {
final int NUM_FLOORS = 5;
Hotel[] floor_number = new Hotel[NUM_FLOORS];
floor_number[0] = new Hotel(20, 14, 168.99);
floor_number[1] = new Hotel(33, 18, 152.99);
floor_number[2] = new Hotel(33, 12, 152.99);
floor_number[3] = new Hotel(33, 15, 152.99);
floor_number[4] = new Hotel(16, 8, 254.99);
System.out.printf("%-6s %-8s %-10s %-15s %-12s %-10s\n",
"Floor", "Rooms", "Occupied", "Occupancy Rate", "Room Price", "Revenue");
for (int i = 0; i < floor_number.length; i++) {
Hotel current_hotel_number = floor_number[i];
int rooms = current_hotel_number.getRooms();
int occupiedRooms = current_hotel_number.getOccupiedRooms();
double roomCost = current_hotel_number.getRoomCost();
double occupancyRate = current_hotel_number.getFloorOccupancyRate();
double revenue = current_hotel_number.getFloorRevenue();
System.out.printf("%-6d %-8d %-10d %-14.1f%% $ %-10.2f $ %-9.2f\n",
i + 1, rooms, occupiedRooms, occupancyRate, roomCost, revenue);
}
}
}
//Hotel.java
public class Hotel
{
private int rooms ;
private int occRooms ;
private double roomCost ;
public Hotel()
{
rooms = 0 ;
occRooms = 0 ;
roomCost = 0.0 ;
}
public Hotel(int rms, int occRms, double rmCst)
{
rooms = rms ;
occRooms = occRms ;
roomCost = rmCst ;
}
public Hotel(String rms, String occRms, String rmCst)
{
rooms = Integer.parseInt(rms) ;
occRooms = Integer.parseInt(occRms) ;
roomCost = Double.parseDouble(rmCst) ;
}
public void setRooms(int rms)
{
rooms = rms ;
}
public void setRooms(String rms)
{
rooms = Integer.parseInt(rms) ;
}
public void setOccupiedRooms(int occRms)
{
occRooms = occRms ;
}
public void setOccupiedRooms(String occRms)
{
occRooms = Integer.parseInt(occRms) ;
}
public void setRoomCost(double rmCst)
{
roomCost = rmCst ;
}
public void setRoomCost(String rmCst)
{
roomCost = Double.parseDouble(rmCst) ;
}
public int getRooms()
{
return rooms ;
}
public int getOccupiedRooms()
{
return occRooms ;
}
public double getRoomCost()
{
return roomCost ;
}
public double getFloorRevenue()
{
return occRooms * roomCost ;
}
public double getFloorOccupancyRate()
{
return ((double)occRooms / (double)rooms) * 100 ;
}
}
When I compile and run the 2 java files I get an output with incorrect formatting which is attached. I can't find a way to get it to have the correct formatting like the example attached. Can you please help me?



Step by step
Solved in 2 steps with 1 images

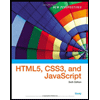
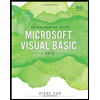
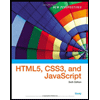
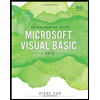
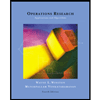