Extend the program to display which player has the highest batting average (hits / atBats) and that average. ---------------------- stats.txt file info: Andrus 574 148 Beltre 543 151 Choo 531 146 Fielder 592 182 Odor 405 108 ---------- Program to modify: /* COSC 1437 Activity 3 Baseball Statistics */ #include #include #include using namespace std; // Structure to hold batting statistics struct Player { string name; int atBats; int hits; }; int main() { ifstream statFile; string playerName; int bats, hits; Player * baseballArray[100]; Player * aPlayer; int numPlayers = 0; statFile.open("stats.txt"); // Continue reading until the end of file while (statFile >> playerName) { statFile >> bats >> hits; // Dynamically create a new Player aPlayer = new Player; aPlayer->name = playerName; aPlayer->atBats = bats; aPlayer->hits = hits; // Store the pointer to the Player struct in the array baseballArray[numPlayers++] = aPlayer; // Display this information cout << aPlayer->name << " has " << aPlayer->atBats << " at bats and " << aPlayer->hits << " hits" << endl; } // For Activity 3: Display which player has the highest batting average. // Display the player's name and average }
Extend the
----------------------
stats.txt file info:
Andrus 574 148
Beltre 543 151
Choo 531 146
Fielder 592 182
Odor 405 108
----------
Program to modify:
/* COSC 1437 Activity 3 Baseball Statistics */
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
// Structure to hold batting statistics
struct Player
{
string name;
int atBats;
int hits;
};
int main()
{
ifstream statFile;
string playerName;
int bats, hits;
Player * baseballArray[100];
Player * aPlayer;
int numPlayers = 0;
statFile.open("stats.txt");
// Continue reading until the end of file
while (statFile >> playerName)
{
statFile >> bats >> hits;
// Dynamically create a new Player
aPlayer = new Player;
aPlayer->name = playerName;
aPlayer->atBats = bats;
aPlayer->hits = hits;
// Store the pointer to the Player struct in the array baseballArray[numPlayers++] = aPlayer;
// Display this information
cout << aPlayer->name << " has " << aPlayer->atBats << " at bats and " << aPlayer->hits << " hits" << endl;
}
// For Activity 3: Display which player has the highest batting average. // Display the player's name and average }

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

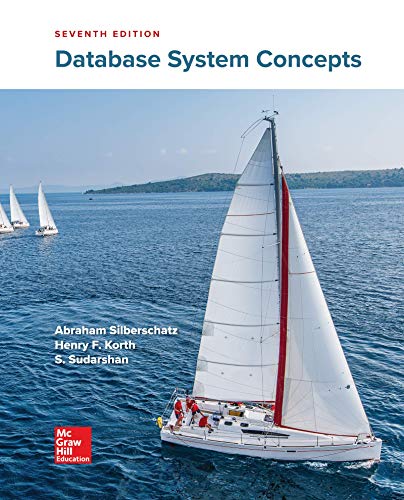
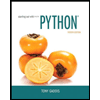
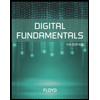
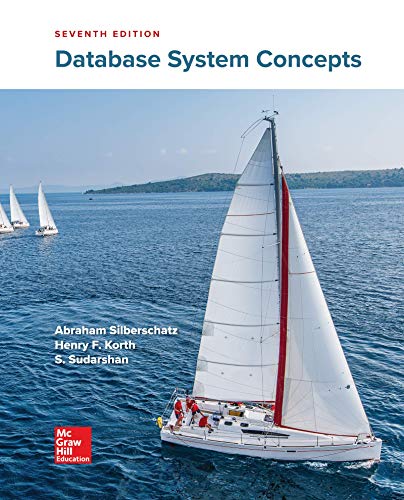
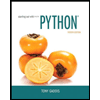
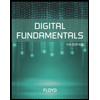
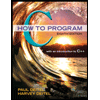
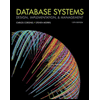
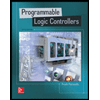