Explore a specific way to delete the root node of the Binary Search Tree (BST) while maintaining the Binary Search Tree (BST) property after deletion. Implementation will be as stated below: [1] Delete the root node value of the BST and replace the root value with the appropriate value of the existing BST . [2] Perform the BST status check by doing an In-Order Traversal of the BST such that even after deletion the BST is maintained. # Class to represent Tree node class Node: # A function to create a new node def __init__(self, key): self.data = key self.left = None self.right = None The root element of the Binary Search Tree is given to you. Below is an illustrated sample of Binary Search Tree nodes for your reference, which in-fact is the same example we discussed in the lecture. root = Node(4) root.left = Node(2) root.right = Node(6) root.left.left = Node(1) root.left.right = Node(3) root.right.left = Node(5) root.right.right = Node(7) Delete the root node value provided and replace the root value with appropriate value such that the BST property is maintaned which would be known by performing the In-Order Traversal of the new BST. Obviously, the In-order traversal of the resulting BST would need to be ascending order When you follow the deletion process and the BST traversal specified - the complexity of the solution will be as below. Time Complexity: O(n) Space Complexity: O(n)
Explore a specific way to delete the root node of
the Binary Search Tree (BST) while maintaining the Binary Search Tree
(BST) property after deletion.
Implementation will be as stated below:
[1] Delete the root node value of the BST and replace the root value with
the appropriate value of the existing BST .
[2] Perform the BST status check by doing an In-Order Traversal of the
BST such that even after deletion the BST is maintained.
# Class to represent Tree node
class Node:
# A function to create a new node
def __init__(self, key):
self.data = key
self.left = None
self.right = None
The root element of the Binary Search Tree is given to you. Below is an illustrated sample of Binary Search Tree nodes for your reference, which in-fact is
the same example we discussed in the lecture.
root = Node(4)
root.left = Node(2)
root.right = Node(6)
root.left.left = Node(1)
root.left.right = Node(3)
root.right.left = Node(5)
root.right.right = Node(7)
Delete the root node value provided and replace the root value
with appropriate value such that the BST property is maintaned which would
be known by performing the In-Order Traversal of the new BST. Obviously, the
In-order traversal of the resulting BST would need to be ascending order
When you follow the deletion process and the BST traversal specified - the complexity of the solution will be as below.
Time Complexity: O(n)
Space Complexity: O(n)

Step by step
Solved in 3 steps with 1 images

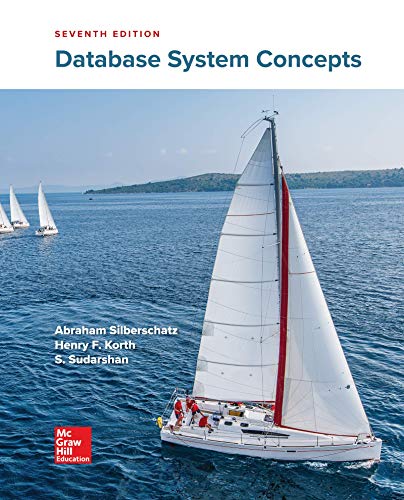
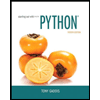
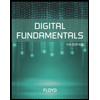
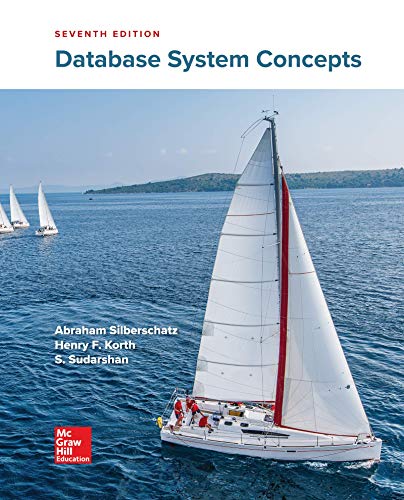
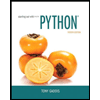
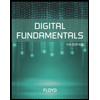
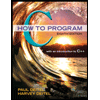
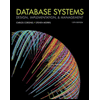
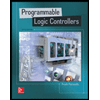