Explain in English sentences what the following C++ given below will do: In your explanation state what each step of the algorithm in the C++ program is performing. For example, make English statements that state facts such as: The #include statement is using the iostream library so that cout and cin can be used in the program. It's basically asking to add comments to the program.
Explain in English sentences what the following C++ given below will do: In your explanation state what each step of the algorithm in the C++ program is performing. For example, make English statements that state facts such as: The #include statement is using the iostream library so that cout and cin can be used in the program. It's basically asking to add comments to the program.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Explain in English sentences what the following C++ given below will do:
In your explanation state what each step of the algorithm in the
C++ program is performing. For example, make English statements that state facts such as:
The #include statement is using the iostream library so that cout and cin can be used in the program.
It's basically asking to add comments to the program.

Transcribed Image Text:```cpp
else
{
cout << " Main Street Pizza Company\n";
cout << " Corner Cafe\n";
cout << " Mama's Fine Italian\n";
cout << " The Chef's Kitchen\n";
}
}
else
{
if (vegan == "yes")
{
if (gluten_free == "yes" ||
gluten_free == "no")
{
cout << " Corner Cafe\n";
cout << " The Chef's Kitchen\n";
}
}
else
{
if (gluten_free == "yes")
{
cout << " Main Street Pizza Company\n";
cout << " Corner Cafe\n";
cout << " The Chef's Kitchen\n";
}
else
{
cout << " Joe's Gourmet Burgers\n";
cout << " Main Street Pizza Company\n";
cout << " Corner Cafe\n";
cout << " Mama's Fine Italian\n";
cout << " The Chef's Kitchen\n";
}
}
}
else
{
cout << "\nError. 'Yes' or 'No' must be chosen.\n"
<< "Rerun the program and try again."
<< endl;
}
}
else
{
cout << "\nError. 'Yes' or 'No' must be chosen.\n"
<< "Rerun the program and try again."
<< endl;
}
else
{
cout << "\nError. 'Yes' or 'No' must be chosen.\n"
<< "Rerun the program and try again."
<< endl;
}
return 0;
}
```
This code is a simple console application written in C++ designed to suggest restaurants based on dietary preferences. Key features:
- **Dietary Options Check**: The program checks if the user prefers vegan and/or gluten-free options and suggests appropriate restaurants.
- **Output**: Based on the selections, various combinations of restaurants are printed.
- **Error Handling**: The program includes error messages if the input does not match the expected 'Yes' or 'No' format.
### Explanation of Logic:
1. **Main Structure**: The code uses nested if-else statements to evaluate dietary preferences (`vegan` and `gluten_free`).
2. **Input Validation**: There are checks to ensure that only 'Yes' or 'No' inputs are deemed valid. If invalid inputs are detected, an error message

Transcribed Image Text:```cpp
#include <iostream>
using namespace std;
int main()
{
string vegetarian,
vegan,
gluten_free;
cout << "Is anyone in your party a vegetarian? ";
cin >> vegetarian;
if (vegetarian == "yes" || vegetarian == "no")
{
cout << "Is anyone in your party a vegan? ";
cin >> vegan;
if (vegan == "yes" || vegan == "no")
{
cout << "Is anyone in your party a gluten-free? ";
cin >> gluten_free;
if (gluten_free == "yes" || gluten_free == "no")
{
cout << "Here are your restaurant choices:"
<< endl;
if (vegetarian == "yes")
{
if (vegan == "yes")
{
if (gluten_free == "yes" ||
gluten_free == "no")
{
cout << " Corner Cafe\n";
cout << " The Chef's Kitchen\n";
}
}
else
{
if (gluten_free == "yes")
{
cout << " Main Street Pizza Company\n";
cout << " Corner Cafe\n";
cout << " The Chef's Kitchen\n";
}
}
}
}
}
}
}
```
### Explanation
This code is a C++ program that helps determine suitable restaurant choices based on dietary preferences such as vegetarian, vegan, and gluten-free options.
#### Key Components
- **Variables:** The program uses three string variables: `vegetarian`, `vegan`, and `gluten_free` to store user input responses.
- **User Input:** The program prompts the user to answer whether anyone in their party is vegetarian, vegan, or gluten-free.
- **Conditional Logic (if statements):** The program then uses a series of nested `if` statements to evaluate the input and determine which restaurants to recommend:
- If a person is vegetarian and vegan, only two options are available: "Corner Cafe" and "The Chef's Kitchen."
- If a person is vegetarian and not vegan but is gluten-free, additional options are available, including "Main Street Pizza Company."
This logical flow aims to filter restaurant options based on dietary needs, ensuring that the meal preferences of the group are accommodated.
Expert Solution

Step 1
Program Explanation:
- The #include statement is using the iostream library so that cout and cin can be used in the program.
- The using statement uses the pre-defined namespace called std in which the functions cout and cin are defined.
- In the main function, string variables vegetarian, vegan, and gluten_free are declared.
- The program reads a user input and stores it in vegetarian. The user inputs whether he/she is a vegetarian or not by using the strings "yes" and "no".
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
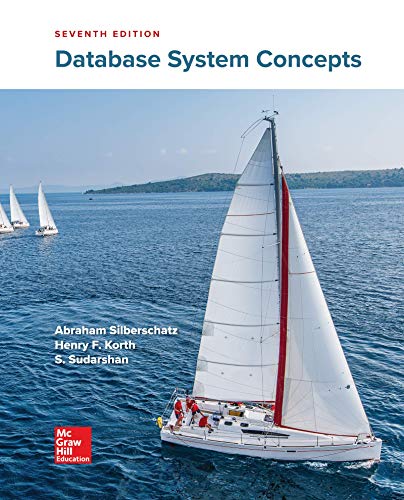
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
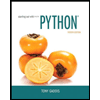
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
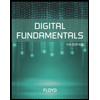
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
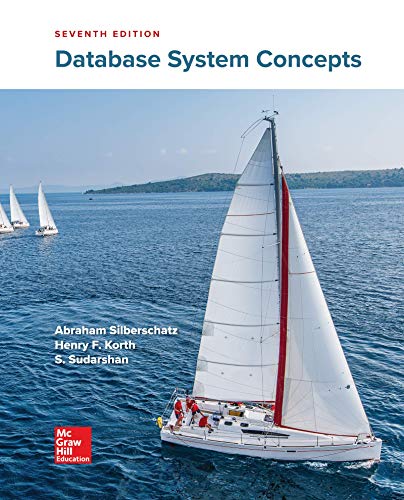
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
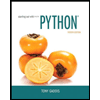
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
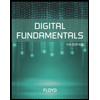
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
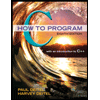
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
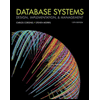
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
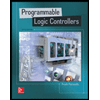
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education