Examine the tester program to see how the functions are used. overload.cpp 1 #include "overload.h" 2 3 4 5 overload.h 1 #ifndef OVERLOAD H 2 #define OVERLOAD H 3 4 #include 5 6 7 8 9 10 Tester.cpp 4 5 6 1 #include 2 using namespace std; 3 #include "overload.h" 7 8 9 10 // Write your functions here #endif 1121314 15 int main() { } // 2 arguments cout << "product(1.9, 3.4): " << product (1.9, 3.4) << endl; cout << "Expected: 6.46" << endl; // 3 arguments cout << "product (7.5, 9.2, 2.1): " << product (7.5, 9.2, 2.1) << endl; cout << "Expected: 144.9" << endl;
Examine the tester program to see how the functions are used. overload.cpp 1 #include "overload.h" 2 3 4 5 overload.h 1 #ifndef OVERLOAD H 2 #define OVERLOAD H 3 4 #include 5 6 7 8 9 10 Tester.cpp 4 5 6 1 #include 2 using namespace std; 3 #include "overload.h" 7 8 9 10 // Write your functions here #endif 1121314 15 int main() { } // 2 arguments cout << "product(1.9, 3.4): " << product (1.9, 3.4) << endl; cout << "Expected: 6.46" << endl; // 3 arguments cout << "product (7.5, 9.2, 2.1): " << product (7.5, 9.2, 2.1) << endl; cout << "Expected: 144.9" << endl;
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter11: Introduction To Classes
Section11.3: Adding Class Functions
Problem 8E
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Need Help with this c++ problem
Write a pair of functions product which return the product of their arguments. The first takes two, and the second takes three.

Transcribed Image Text:Examine the tester program to see how the functions are used.
overload.cpp
1 #include "overload.h"
2
3
4
5
overload.h
1 #ifndef OVERLOAD H
2 #define OVERLOAD H
3
4 #include <string>
5
6
7
8
9
10
Tester.cpp
9
10
// Write your functions here
#endif
1 #include <iostream>
2 using namespace std;
3
4
#include "overload.h"
5
6
7
8
11
12
13
14
15
int main()
{
}
// 2 arguments
cout << "product (1.9, 3.4): << product (1.9, 3.4) << endl;
cout << "Expected: 6.46" << endl;
II
// 3 arguments
cout << "product (7.5, 9.2, 2.1): " << product (7.5, 9.2, 2.1) << endl;
cout << "Expected: 144.9" << endl;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
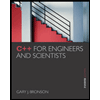
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
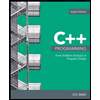
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
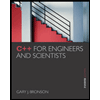
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
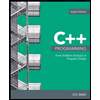
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning