else
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
![The image shows an error log or message from a programming environment, indicating issues found within a function. Below is a transcription of the provided content:
**File Paths and Errors:**
1. **File Path:** `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\`
- **Line:** 10
- **Column:** 33
- **Function:** `void IsolateTargetSoloAsTail(Node*, int)`
- **Error Message:** `[Error] 'countTarget' was not declared in this scope`
2. **File Path:** `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\`
- **Line:** 12
- **Column:** 22
- **Error Message:** `[Error] 'append' was not declared in this scope`
3. **File Path:** `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\`
- **Line:** 16
- **Column:** 32
- **Error Message:** `[Error] 'deleteNode' was not declared in this scope`
4. **File Path:** `C:\Users\r1821655\OneDrive\Dropbox\Screenshots\`
- **Line:** 17
- **Column:** 28
- **Error Message:** `[Error] 'append' was not declared in this scope`
**Explanation:**
This log snippet can be useful for debugging code, and the errors suggest that certain functions or variables (`countTarget`, `append`, `deleteNode`) are not recognized in the given scope of the function `void IsolateTargetSoloAsTail(Node*, int)`. This may be due to missing declarations or failed imports of necessary libraries. Users should check to ensure these functions or variables are properly declared and accessible in the current context of the program.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3632b9ca-0e22-48c8-943d-c9e08fc0f04c%2F2268963c-8cb1-4263-bb19-5826eb0f65c8%2Fiw16dw_processed.png&w=3840&q=75)


By looking at the errors we can understand the function like-
1- If in the counttarget function there is nothing in the list then -
We have to append the target which we take.
2- If there is something in the list then we have to delete the node at headptr* then append the target at the place.
Step by step
Solved in 2 steps

How do I fix the problem? What should I can?
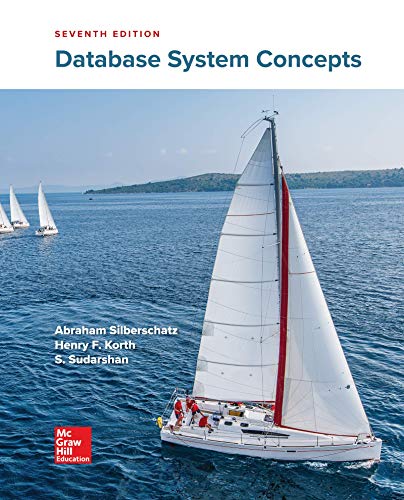
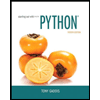
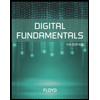
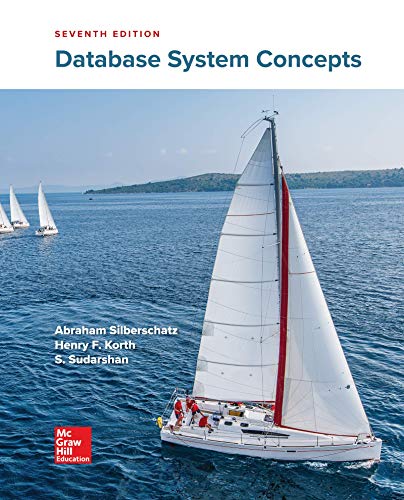
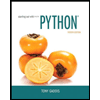
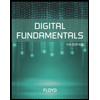
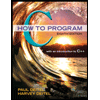
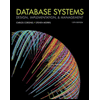
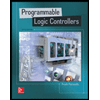