Edit the following code to read the input for 10 students from a file testData1.txt and output the average of scores for 10 students using a loop. #include using namespace std; #include int main(){ string name1,name2,name3; double score1,score2,score3; char c1,c2,c3; double avg; ifstream inputFile; ofstream outputFile; inputFile.open("testData1.txt"); outputFile.open("Results.txt"); inputFile.clear(); inputFile>>name1>>score1>>c1; inputFile.clear(); inputFile.ignore(200,'\n'); inputFile>>name2>>score2>>c2; inputFile.clear(); inputFile.ignore(200,'\n'); inputFile>>name3>>score3>>c3; avg =(score1 + score2 +score3)/3.0; outputFile << endl<
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
(This is for DevC++. I use version 5.11)
Edit the following code to read the input for 10 students from a file testData1.txt and output the average of scores for 10 students using a loop.
#include <fstream>
using namespace std;
#include <iomanip>
int main(){
string name1,name2,name3;
double score1,score2,score3;
char c1,c2,c3;
double avg;
ifstream inputFile;
ofstream outputFile;
inputFile.open("testData1.txt");
outputFile.open("Results.txt");
inputFile.clear();
inputFile>>name1>>score1>>c1;
inputFile.clear();
inputFile.ignore(200,'\n');
inputFile>>name2>>score2>>c2;
inputFile.clear();
inputFile.ignore(200,'\n');
inputFile>>name3>>score3>>c3;
avg =(score1 + score2 +score3)/3.0;
outputFile << endl<<setw(20)<<left<<"Name" <<setw(20)<<"Score"<<setw(20)<<left<<"School Year";
outputFile << endl<<setw(20)<<left<<"----" <<setw(20)<<"-----"<<setw(20)<<left<<"-----------";
outputFile << endl<<setw(20)<<left<<name1 <<setw(20)<<score1<<setw(20)<<left<<c1;
outputFile << endl<<setw(20)<<left<<name2 <<setw(20)<<score2<<setw(20)<<left<<c2;
outputFile << endl<<setw(20)<<left<<name3 <<setw(20)<<score3<<setw(20)<<left<<c3;
outputFile << "\n\nAverage score is "<<fixed<<showpoint<<setprecision(2)<<avg<<endl;
inputFile.close();
outputFile.close();
}
Suppose testDat1.txt has following info
Rose 6.1 Senior
Nate 2.1 J
Kim 2.3 Freshman
Robert 10 Junior
Kale 9 F
Netalie 8 Senior
Melanie 10 J
Elan 1 F
Tom 7 J
Keen 10 S

In this problem, you need to edit given code, you need to use loop in it.
In given code, to read 3 students data, they have written separate 3 statements.
They have used different variables to store each student information like 'name1' for first student, 'name2' for second student and so on.
But when you will use loop, you don't need to declare different variables for each student.
You can declare one variable for all students like 'name' for all students.
You can use this 'name' variable in loop, so for each iteration of loop 'name' variable will store each student name.
So there will be only one variable for name called 'name' , only one variable for score called 'score' and only one variable for character called 'c' .
In loop, you need to read the data line by line from input file and simultaneously need to write the data in output file.
To calculate average, you will require sum of all scores of students, so you can do addition of scores also in loop.
After loop, you can calculate average by dividing sum of scores. Then you can write that average value in output file.
Step by step
Solved in 3 steps with 2 images

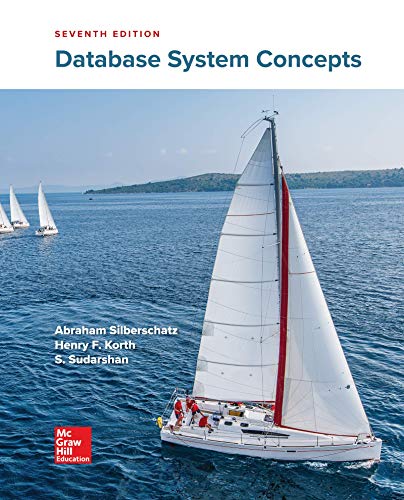
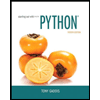
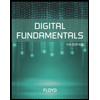
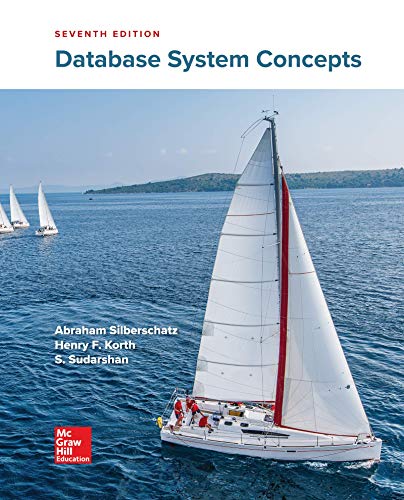
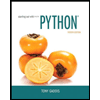
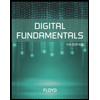
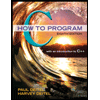
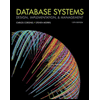
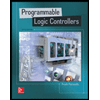