e a JAVA program in which a for loop is used to prompt user to enter five integers one at a time. The program will print the sum and the product of the integers. For example, if the integers are: 3, 5, 2, 1, 2, then the sum will be 3+5+2+1+2 = 13, and the product will be (3)(5)(2)(1)(2) = 60.
Write a JAVA

Here's an example of a Java program that prompts the user to enter five integers and calculates the sum and product of those integers:-
Java Code:-
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int sum = 0;
int product = 1;
for (int i = 1; i <= 5; i++) {
System.out.print("Enter an integer: ");
int num = scanner.nextInt();
sum += num;
product *= num;
}
System.out.println("The sum of the integers is: " + sum);
System.out.println("The product of the integers is: " + product);
}
}
Output:-
Enter an integer: 3
Enter an integer: 5
Enter an integer: 2
Enter an integer: 1
Enter an integer: 2
The sum of the integers is: 13
The product of the integers is: 60
Step by step
Solved in 3 steps with 1 images

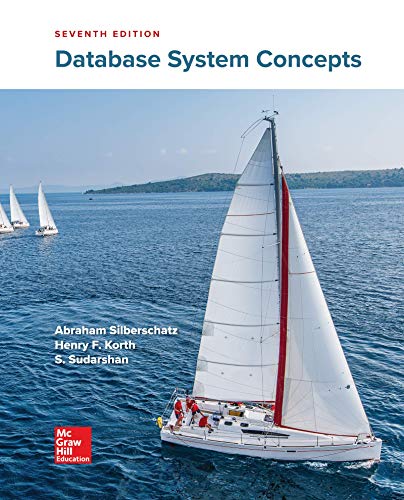
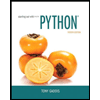
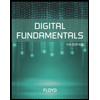
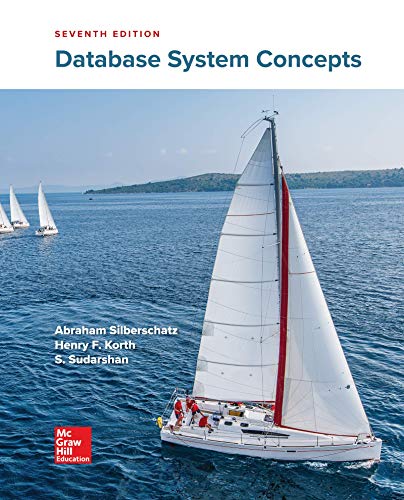
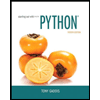
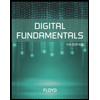
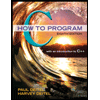
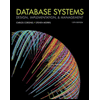
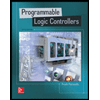