Develop an application to do the following: 1. Client read a line of characters (data) from its keyboard and send the data to the server. 2. The server receives the data and converts characters to uppercase. 3. The server sends the modified data to the client. 4. The client receives the modified data and displays the line on its screen.


Server Algorithm:
1. Create a socket object for the server.
2. Bind the socket to a specific address and port (e.g., localhost:12345).
3. Listen for incoming connections.
4. Display "Server is listening for connections..." message.
Loop:
5. Accept a connection from a client, establishing a client socket and client address.
6. Display "Connection established with [client_address]" message.
Sub-Loop:
7. Receive data from the client (up to 1024 bytes).
8. If no data is received, exit the sub-loop.
9. Convert the received data to uppercase.
10. Send the modified data back to the client.
End of Sub-Loop.
11. Close the client socket.
End of Loop.
Client Algorithm:
1. Create a socket object for the client.
2. Connect to the server at a specific address and port (e.g., localhost:12345).
Loop:
3. Prompt the user to enter a line of characters or 'exit' to quit.
4. Read the user's input.
5. Send the input data to the server.
6. If the input is 'exit', exit the loop and close the socket.
7. Receive the modified data from the server (up to 1024 bytes).
8. Display the modified data on the screen.
End of Loop.
9. Close the client socket.
Step by step
Solved in 4 steps with 3 images

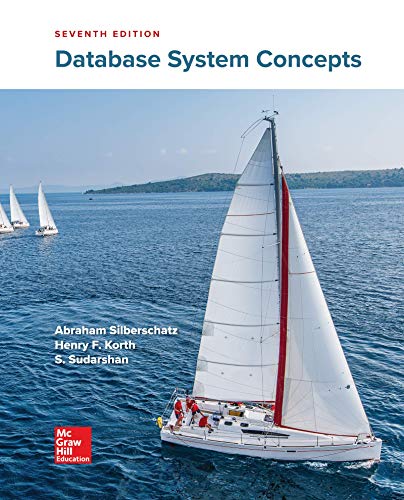
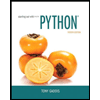
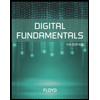
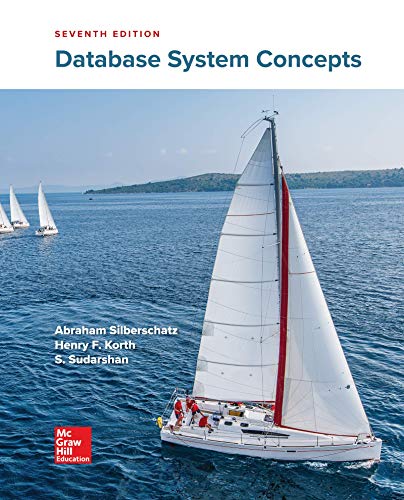
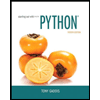
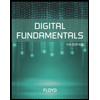
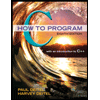
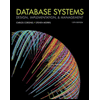
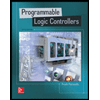