Develop a simple table of examples in some domain, such as classifying plants by species, and trace the construction of a decision tree by the ID3 algorithm. Construct a decision tree using py
Develop a simple table of examples in some domain, such as classifying plants by species, and trace the construction of a decision tree by the ID3

ID3 Algorithm for Decision Tree Construction
Input:
- Data: A dataset with features and a target column.
- Attributes: A list of attributes (features) to consider for splitting.
- Target_column: The column representing the target variable (class labels).
Output:
- Decision Tree: A hierarchical tree structure used for classification.
Algorithm:
1. If all samples in the dataset belong to the same class:
- Return a leaf node with the class label.
2. If there are no attributes left to split on:
- Return a leaf node with the majority class label in the dataset.
3. Calculate the entropy of the current dataset with respect to the target column:
- Entropy(S) = -Σ(p_i * log2(p_i)) for each unique class label in the target column.
4. For each attribute in the Attributes list:
a. Calculate the weighted entropy for each unique value of the attribute:
- Calculate the entropy of the subset of data for each unique value of the attribute.
- Weighted_entropy(Attribute) = Σ(weight * Entropy(subset)) for all unique values of the attribute.
b. Calculate the Information Gain for the attribute:
- Information Gain(Attribute) = Entropy(S) - Weighted_entropy(Attribute).
5. Select the attribute with the highest Information Gain as the best attribute to split on.
6. Create a decision tree node with the best attribute as the attribute name.
7. Remove the best attribute from the Attributes list.
8. For each unique value of the best attribute:
a. Create a branch from the decision tree node with the value of the best attribute.
b. Recursively call the ID3 algorithm on the subset of data with the best attribute equal to the current value.
9. Return the decision tree.
The resulting decision tree represents a hierarchy of decisions based on the selected attributes, with leaf nodes containing the predicted class labels.
Step by step
Solved in 4 steps with 3 images

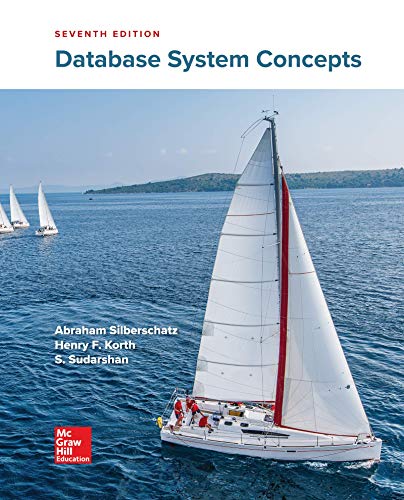
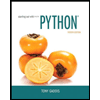
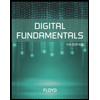
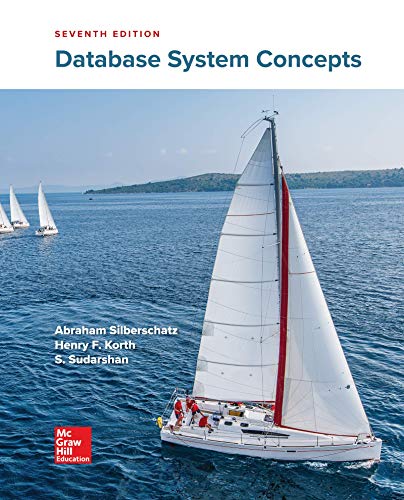
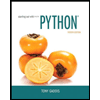
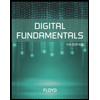
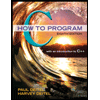
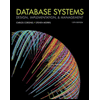
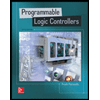