Design/Implementation: Are there noticeable issues/flaws in the design and implementation of the code? How would you remedy such issues?
Reviewing this
- Design/Implementation: Are there noticeable issues/flaws in the design and implementation of the code? How would you remedy such issues?
- Readability: Is the code well organized and easy to follow? Can you understand what is going on, easily?
- Documentation: Is the documentation well-written? does it explain what the code is accomplishing and how? Remember good documentation includes descriptive variable and function names in addition to clear/concise annotation.
- Efficiency: Was the code efficient, without sacrificing readability and understanding?
#include <iostream>
using namespace std;
int main()
{
double meal, tax, tip, total; //create variables
double taxPercentage = .0675, tipPercentage = .2; //initiate percentage values
cout << "Enter a meal charge: \n";
cin >> meal; //enter meal
tax = meal * taxPercentage;
tip = (meal + tax) * tipPercentage;
total = meal+tax+tip;
cout << "Meal Charge: " << meal << endl; /*output the meal charge*/
cout << "Tax: " << tax << endl; /*output the tax*/
cout << "Tip: " << tip << endl; /*output the tip*/
cout << "Total: " << total << endl; /*output the total*/
return 0;
}
#include <iostream>
using namespace std;
int main()
{
double mealCharge; // declaring variables to be used
double Tax;
double Tip;
double Total;
cout << "Enter a meal charge: "; // asking user for meal charge input
cin >> mealCharge; // assigning user input to mealCharge
Tax = mealCharge * .0675; // math, deriving tip tax and total from mealCharge
Tip = (mealCharge + Tax) * .2;
Total = mealCharge + Tip + Tax;
cout << "Meal Charge: " << mealCharge << endl; // Outputing answers
cout << "Tax: " << Tax << endl;
cout << "Tip: " << Tip << endl;
cout << "Total: " << Total << endl;
return 0; // program end
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

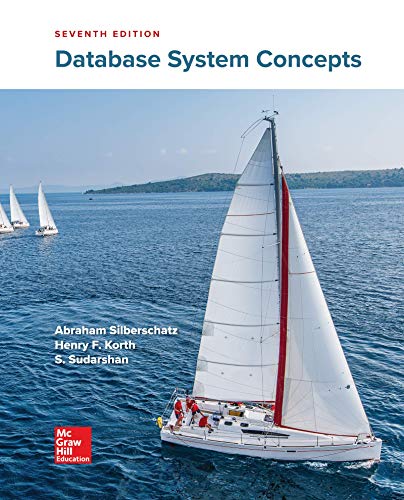
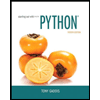
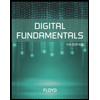
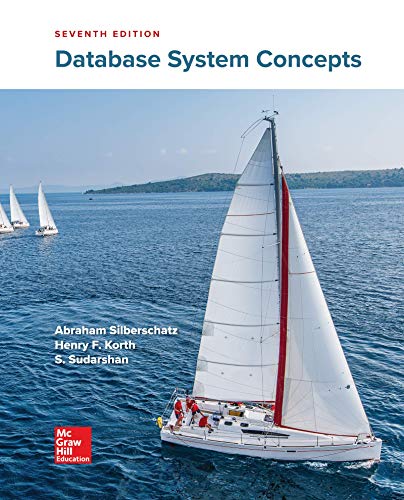
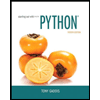
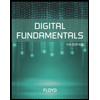
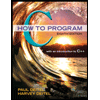
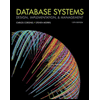
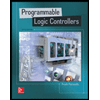