Design a console program that will print details of prescribed textbooks of students. Make use of an abstract class Book with variables that will store the author, title, category and price of a book. Create a constructor that accepts the author, title and category through parameters and assign these to the class variables. Create an abstract set method for the price of a book; also create get methods for the variables. Create a subclass TextBook that extends the Book class and implements an iPrintable interface. The interface that must be added is shown below: public interface iPrintable { String DisplayDetails(); The TextBook subclass has a private variable named yearPrescribed for which a get method must be written. The constructor of the TextBook class must accept the author, title, category and yearPrescribed through parameters. Write the code for the setPrice() and DisplayDetails() methods.
Please help with the question on the picture


Book.java
public abstract class Book
{
public String author,title,category,price;
//constructor
public Book(String author,String title,String category)
{
this.author=author;
this.title=title;
this.category=category;
}
//setprice abstrac method
public abstract void setPrice(String price);
//get methods for all other variables
public String getAuthor() {
return this.author;
}
public String getTtile() {
return this.title;
}
public String getCategory() {
return this.category;
}
}
iPrintable.java
public interface iPrintable {
String DisplayDetails();
}
TextBool.java
public class TextBook extends Book implements iPrintable{
private String yearPrescribed;//instance varibale
//counstructor
public TextBook(String author, String title, String category,String year) {
super(author, title, category);
this.yearPrescribed=year;
}
//get yearPrescribed method
String getYearPrescribed()
{
return this.yearPrescribed;
}
@Override
public void setPrice(String price) {
this.price=price;
//throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public String DisplayDetails() {
String str="Author:"+getAuthor()+"\nTitel:"+getTtile()+"\nCategory:"+getCategory()+"\nYear Prescribed:"+getYearPrescribed()+"\nPrice:"+this.price;
return str;
}
}useBook.java
public class useBook {
public static void main(String[] args) {
TextBook book1=new TextBook("N.O.Body", "Intro to programming", "Non-fiction", "DISD1");
book1.setPrice("R350");
TextBook book2=new TextBook("S.O.Mebody","Web Development Intermediate","Non-fiction", "DISD2");
book2.setPrice("R350");
System.out.println("Book Details");
System.out.println(book1.DisplayDetails());
System.out.println("\n\n");
System.out.println(book2.DisplayDetails());
}
}
Step by step
Solved in 2 steps with 1 images

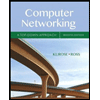
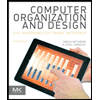
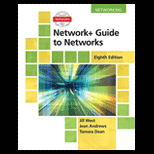
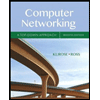
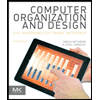
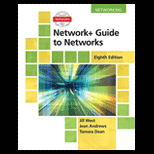
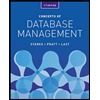
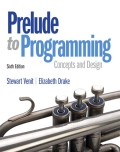
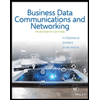