Design a class named Complex for representing complex numbers with the methods add, subtract, multiply, divide, and abs for performing complex number operations, and override toString method for returning a string representation for a complex number. The toString method returns (a + bi) as a string. If b is 0, it simply returns a. Your Complex class should also implement Cloneable and Comparable. Compare two complex numbers using their absolute values. Provide three constructors Complex(a, b), Complex(a), and Complex(). Complex() creates a Complex object for number 0, and Complex(a) creates a Complex object with 0 for b. Also provide the getRealPart() and getImaginaryPart() methods for returning the real part and the imaginary part of the complex number, respectively.
Design a class named Complex for representing complex numbers with the methods add, subtract, multiply, divide, and abs for performing complex number operations, and override toString method for returning a string representation for a complex number. The toString method returns (a + bi) as a string. If b is 0, it simply returns a. Your Complex class should also implement Cloneable and Comparable. Compare two complex numbers using their absolute values. Provide three constructors Complex(a, b), Complex(a), and Complex(). Complex() creates a Complex object for number 0, and Complex(a) creates a Complex object with 0 for b. Also provide the getRealPart() and getImaginaryPart() methods for returning the real part and the imaginary part of the complex number, respectively.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I need some help generating a UML diagram from this assigment.

Transcribed Image Text:Design a class named Complex for representing complex numbers with the methods add,
subtract, multiply, divide, and abs for performing complex number operations, and
override toString method for returning a string representation for a complex number. The
toString method returns (a + bi) as a string. If b is 0, it simply returns a. Your Complex
class should also implement Cloneable and Comparable. Compare two complex numbers
using their absolute values.
Provide three constructors Complex(a, b), Complex(a), and Complex(). Complex()
creates a Complex object for number 0, and Complex(a) creates a Complex object with 0
for b. Also provide the getRealPart() and getImaginaryPart() methods for returning the
real part and the imaginary part of the complex number, respectively.
Expert Solution

Step 1 of 2
public class Complex implements Cloneable { private double a; private double b; public Complex(double a, double b) { this.a = a; this.b = b; } public Complex(double a) { this.a = a; b = 0; } public Complex() { this.a = 0; this.b = 0; } public double getRealPart() { return this.a; } public double getImaginaryPart() { return this.b; } @Override public String toString() { if (b < 0) { return this.a + " " + this.b + "i"; } else if (b > 0) { return this.a + " + " + this.b + "i"; } else { return String.valueOf(this.a); } } @Override protected Object clone() throws CloneNotSupportedException { return super.clone(); } public Complex add(Complex c) { double real = this.a + c.getRealPart(); double imag = this.b + c.getImaginaryPart(); return new Complex(real, imag); } public Complex subtract(Complex c) { double real = this.a - c.getRealPart(); double imag = this.b - c.getImaginaryPart(); return new Complex(real, imag); } public Complex multiply(Complex c) { double real = this.a * c.getRealPart(); double imag = this.b * c.getImaginaryPart(); return new Complex(real, imag); } public Complex divide(Complex c) { double real = this.a / c.getRealPart(); double imag = this.b / c.getImaginaryPart(); return new Complex(real, imag); } public Complex abs() { double real = this.a < 0 ? -this.a : this.a; double imag = this.b < 0 ? -this.b : this.b; return new Complex(real, imag); } }
===========================================================================
import java.util.Scanner; public class Test { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter the first complex number: "); double a = input.nextDouble(); double b = input.nextDouble(); Complex c1 = new Complex(a, b); System.out.print("Enter the second complex number: "); double c = input.nextDouble(); double d = input.nextDouble(); Complex c2 = new Complex(c, d); System.out.println("(" + c1 + ")" + " + " + "(" + c2 + ")" + " = " + c1.add(c2)); System.out.println("(" + c1 + ")" + " - " + "(" + c2 + ")" + " = " + c1.subtract(c2)); System.out.println("(" + c1 + ")" + " * " + "(" + c2 + ")" + " = " + c1.multiply(c2)); System.out.println("(" + c1 + ")" + " / " + "(" + c2 + ")" + " = " + c1.divide(c2)); System.out.println("|" + c1 + "| = " + c1.abs()); Complex c3 = null; try { c3 = (Complex)c1.clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } System.out.println(c1 == c3); System.out.println(c3.getRealPart()); System.out.println(c3.getImaginaryPart()); } }
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
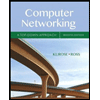
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
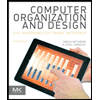
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
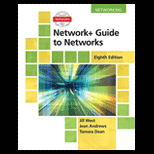
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
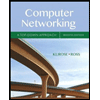
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
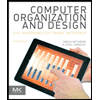
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
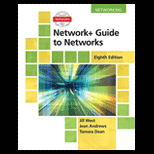
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
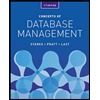
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
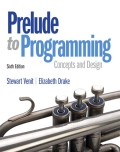
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
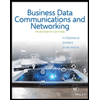
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY