Design a c++ program that creates a menu that gives the choice of two “magic” math formulas. You will be declaring variables, using while loops, for loops and if statements, taking in and printing out values. There will be a lot of curly brackets so make sure you properly indent AND comment each closing curly bracket with line comments noting which statement it is closing. Also remember that if you have more than one statement, you MUST use curly brackets. Finally, do a little at a time and print out values as you go along. Test your for loops with just a couple of values that you can manually compute. Test your menu first with just simple print statements when you choose a menu number. See an abbreviated example below to give you an idea of how your output should look. When your program starts it should print out your name and student ID. Then display a menu with 3 options. Almost Always 99 Always 3 Exit If the user presses 1: Use print (cout) statements to show every mathematical step. For Every number from 0 to (and including) 99 do the following: If the number (first) is less than 10, multiply it by 10. Break the two digit number into a tens digit and a ones digit (Remember that an integer / integer results in an truncated integer, not rounded up or down) For example 26/10 = 2 (tens), 2*10 = 20, 26-20 = 6 (ones) Reverse the digits (second) (Again think of this as reversing the tens digit with the ones digit). Subtract the second number from the first (third) If the result (third) is negative, multiple by -1. If the result (third) is less than 10, multiple it by 10. Take the result (third), reverse the digits (fourth) Add (third) to (fourth). If the answer is 99 print out a congratulations message. If not, print a message that you may have done something wrong If the user presses 2: Use print (cout) statements to show every mathematical step. Ask somebody to pick a number. Double that number (B). Add 9 to the result. (C) Subtract 3 from that result (D). Divide that result (E) by 2. And finally, subtract the original number. If the answer is 3 print out a congratulations message. If not, print a message that you may have done something wrong If the user presses 3: Give an ending message and exit out of the program If the user presses any number other than 1, 2 or 3 Go back to the top of the menu You should create a file called yourlastnamePA1.cpp (where yourlastname is your last name), test it on the csegrid by typing g++ -o pa1 yourlastnamePA1.cpp (that’s a dash Oh, and stands for output) then if it compiles without errors ./yourlastnamePA1.cpp When it is ready move it over to your PC or Mac and submit it to canvas under PA1. Extra Credit: You can get up to 10% extra credit for successfully a) using switch statements for your top level menu items and b) creating a 4th menu item which does the same thing as item 2, but reads information in from a file instead of from the console. For the entire program you don’t have to worry about building in error checking. The user will always enter valid information and make valid choices. You are expected to fully comment your code including: /*Name: Last Date Modified: Assignment: Description Status: Compiled and ran successfully on csegrid.ucdenver.edu Met all requirements */
Design a c++ program that creates a menu that gives the choice of two “magic” math formulas.
You will be declaring variables, using while loops, for loops and if statements, taking in and printing out values. There will be a lot of curly brackets so make sure you properly indent AND comment each closing curly bracket with line comments noting which statement it is closing. Also remember that if you have more than one statement, you MUST use curly brackets. Finally, do a little at a time and print out values as you go along. Test your for loops with just a couple of values that you can manually compute. Test your menu first with just simple print statements when you choose a menu number.
See an abbreviated example below to give you an idea of how your output should look.
When your program starts it should print out your name and student ID. Then display a menu with 3 options.
Almost Always 99
Always 3
Exit
If the user presses 1:
Use print (cout) statements to show every mathematical step.
For Every number from 0 to (and including) 99 do the following:
If the number (first) is less than 10, multiply it by 10.
Break the two digit number into a tens digit and a ones digit
(Remember that an integer / integer results in an truncated integer, not rounded up or down)
For example 26/10 = 2 (tens), 2*10 = 20, 26-20 = 6 (ones)
Reverse the digits (second) (Again think of this as reversing the tens digit with the ones digit).
Subtract the second number from the first (third)
If the result (third) is negative, multiple by -1.
If the result (third) is less than 10, multiple it by 10.
Take the result (third), reverse the digits (fourth)
Add (third) to (fourth).
If the answer is 99 print out a congratulations message. If not, print a message that you may have done something wrong
If the user presses 2:
Use print (cout) statements to show every mathematical step.
Ask somebody to pick a number.
Double that number (B).
Add 9 to the result. (C)
Subtract 3 from that result (D).
Divide that result (E) by 2.
And finally, subtract the original number.
If the answer is 3 print out a congratulations message. If not, print a message that you may have done something wrong
If the user presses 3:
Give an ending message and exit out of the program
If the user presses any number other than 1, 2 or 3
Go back to the top of the menu
You should create a file called yourlastnamePA1.cpp (where yourlastname is your last name), test it on the csegrid by typing
g++ -o pa1 yourlastnamePA1.cpp (that’s a dash Oh, and stands for output)
then if it compiles without errors
./yourlastnamePA1.cpp
When it is ready move it over to your PC or Mac and submit it to canvas under PA1.
Extra Credit: You can get up to 10% extra credit for successfully a) using switch statements for your top level menu items and b) creating a 4th menu item which does the same thing as item 2, but reads information in from a file instead of from the console.
For the entire program you don’t have to worry about building in error checking. The user will always enter valid information and make valid choices.
You are expected to fully comment your code including:
/*Name:
Last Date Modified:
Assignment:
Description
Status: Compiled and ran successfully on csegrid.ucdenver.edu
Met all requirements
*/

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

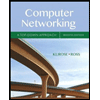
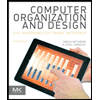
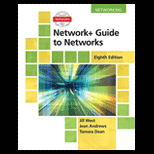
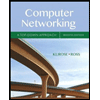
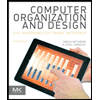
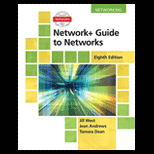
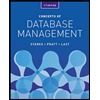
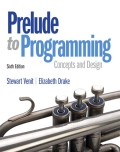
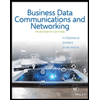