Description of the Problem Write a program to allow the academic staff to enter the coursework marks and final marks for the students. The program reads scores in three categories: test, assignments and final. Each category is weighted: its points are scaled up to a fraction of the 100 percent grade for the course. As the program begins reading each category, it first prompts for the category's weight. The user begins by entering student id and name follows by the scores earned on test. The program asks whether test scores were shifted, interpreting an answer of 1 to mean "yes" and 2 to mean "no." If there is a shift, the program prompts for the shift amount, and the shift is added to the user's test score. Exam scores are capped at a max of 100; for example, if the user got 95 and there was a shift of 10, the score to use would be 100. The midterm's "weighted score" is printed, which is equal to the user's score multiplied by the exam's weight. Next, the program prompts for data about the final. The behavior for each is the same as the behavior for test. Next, the user enters information about his/her homework, including the weight and how many assignments were given. For each assignment, the user enters a score and points possible. Use a cumulative sum as described in lecture. Part of the homework score comes from sections attended. We will simplify the formula to assume that each section attended is worth 3 points, up to a maximum of 34 points. Once the program has read the user information for both exams and homework, it prints the student's overall percentage earned in the course, which is the sum of the weighted scores from the three categories, as shown below: Grade=Weighted TestScore+WeightedFinal ExamScore + WeightedHomeworkScore 78 (14+17+19+(5x3) Grade (700x 20)+(100×30)+(14 Grade=15.6+30.0+34.6 :50 Grade=80.2 The program prints a loose guarantee about a minimum grade the student will get in the course, based on the following scale. See the logs of execution on the course web site to see the expected output for each grade range. 90% and above: A; 89.99% -80%: B; 79.99%-70%: C; 69.99% -60%: D; under 60%: F After printing the guaranteed minimum grade, print a custom message of your choice about the grade. This message should be different for each grade range shown above. It should be at least 1 line of any non-offensive text you like. Next, provide an option to repeat the next grading for the students with an appropriate prompt message. You should handle the following two special cases of input: • A student can receive extra credit on an individual assignment, but the total points for homework are capped at the maximum possible. For example, a student can receive a score of 22/20 on one homework assignment, but if their total homework score for all assignments is 63/60, this score should be capped at 60/60. Section points are capped at 34. • Cap exam scores at 100. If the raw or shifted exam score exceeds 100, a score of 100 is used. Otherwise, you may assume the user enters valid input. When prompted for a value, the user will enter an integer in the proper range. The user will enter a number of homework
Description of the Problem Write a program to allow the academic staff to enter the coursework marks and final marks for the students. The program reads scores in three categories: test, assignments and final. Each category is weighted: its points are scaled up to a fraction of the 100 percent grade for the course. As the program begins reading each category, it first prompts for the category's weight. The user begins by entering student id and name follows by the scores earned on test. The program asks whether test scores were shifted, interpreting an answer of 1 to mean "yes" and 2 to mean "no." If there is a shift, the program prompts for the shift amount, and the shift is added to the user's test score. Exam scores are capped at a max of 100; for example, if the user got 95 and there was a shift of 10, the score to use would be 100. The midterm's "weighted score" is printed, which is equal to the user's score multiplied by the exam's weight. Next, the program prompts for data about the final. The behavior for each is the same as the behavior for test. Next, the user enters information about his/her homework, including the weight and how many assignments were given. For each assignment, the user enters a score and points possible. Use a cumulative sum as described in lecture. Part of the homework score comes from sections attended. We will simplify the formula to assume that each section attended is worth 3 points, up to a maximum of 34 points. Once the program has read the user information for both exams and homework, it prints the student's overall percentage earned in the course, which is the sum of the weighted scores from the three categories, as shown below: Grade=Weighted TestScore+WeightedFinal ExamScore + WeightedHomeworkScore 78 (14+17+19+(5x3) Grade (700x 20)+(100×30)+(14 Grade=15.6+30.0+34.6 :50 Grade=80.2 The program prints a loose guarantee about a minimum grade the student will get in the course, based on the following scale. See the logs of execution on the course web site to see the expected output for each grade range. 90% and above: A; 89.99% -80%: B; 79.99%-70%: C; 69.99% -60%: D; under 60%: F After printing the guaranteed minimum grade, print a custom message of your choice about the grade. This message should be different for each grade range shown above. It should be at least 1 line of any non-offensive text you like. Next, provide an option to repeat the next grading for the students with an appropriate prompt message. You should handle the following two special cases of input: • A student can receive extra credit on an individual assignment, but the total points for homework are capped at the maximum possible. For example, a student can receive a score of 22/20 on one homework assignment, but if their total homework score for all assignments is 63/60, this score should be capped at 60/60. Section points are capped at 34. • Cap exam scores at 100. If the raw or shifted exam score exceeds 100, a score of 100 is used. Otherwise, you may assume the user enters valid input. When prompted for a value, the user will enter an integer in the proper range. The user will enter a number of homework
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question

Transcribed Image Text:Description of the Problem
Write a program to allow the academic staff to enter the coursework marks and final marks for
the students. The program reads scores in three categories: test, assignments and final. Each
category is weighted: its points are scaled up to a fraction of the 100 percent grade for the
course. As the program begins reading each category, it first prompts for the category's weight.
The user begins by entering student id and name follows by the scores earned on test. The
program asks whether test scores were shifted, interpreting an answer of 1 to mean "yes" and
2 to mean "no." If there is a shift, the program prompts for the shift amount, and the shift is
added to the user's test score. Exam scores are capped at a max of 100; for example, if the user
got 95 and there was a shift of 10, the score to use would be 100. The midterm's "weighted
score" is printed, which is equal to the user's score multiplied by the exam's weight. Next, the
program prompts for data about the final. The behavior for each is the same as the behavior
for test.
Next, the user enters information about his/her homework, including the weight and how many
assignments were given. For each assignment, the user enters a score and points possible. Use
a cumulative sum as described in lecture. Part of the homework score comes from sections
attended. We will simplify the formula to assume that each section attended is worth 3 points,
up to a maximum of 34 points.
Once the program has read the user information for both exams and homework, it prints the
student's overall percentage earned in the course, which is the sum of the weighted scores from
the three categories, as shown below:
Grade Weighted TestScore+WeightedFinal ExamScore + WeightedHomeworkScore
Grade= 78 x 20)+(100×30)+(15+20+25+34
100
Grade 15.6+30.0+34.6
Grade 80.2
*50
The program prints a loose guarantee about a minimum grade the student will get in the course,
based on the following scale. See the logs of execution on the course web site to see the
expected output for each grade range.
90% and above: A; 89.99% -80%: B; 79.99% -70%: C; 69.99% -60%: D; under 60%: F
After printing the guaranteed minimum grade, print a custom message of your choice about the
grade. This message should be different for each grade range shown above. It should be at
least 1 line of any non-offensive text you like. Next, provide an option to repeat the next grading
for the students with an appropriate prompt message.
You should handle the following two special cases of input:
• A student can receive extra credit on an individual assignment, but the total points for
homework are capped at the maximum possible. For example, a student can receive a
score of 22/20 on one homework assignment, but if their total homework score for all
assignments is 63/60, this score should be capped at 60/60. Section points are capped at 34.
• Cap exam scores at 100. If the raw or shifted exam score exceeds 100, a score of 100 is
used.
Otherwise, you may assume the user enters valid input. When prompted for a value, the user
will enter an integer in the proper range. The user will enter a number of homework
![assignments > 1, and the sum of the four weights will be exactly 100. The weight of each
category will be a non-negative number. Exam shifts will be ≥ 0.
The program should be developed in a modular way using the functions, parameters, and
returns value to eliminate redundancy.
Give meaningful names to variables, and use proper indentation and whitespace. Follow
Python's naming standards as specified in lecture. Localize variables when possible; declare
them in the smallest scope needed. Include meaningful comment headers at the top of your
program and at the start of each function. Limit line lengths to 100 chars. Consider to use some
customized functions to reduce the code redundancy and promote the code reusability.
Sample Run
Enter Student Name: Derrick
Enter Student ID: P1234567
Test:
Weight (0-100) ? 20
Score earned? 78
Were scores shifted (1-yes, 2=no)? 2
Total points =
78 / 1007
Weighted score = 15.6 / 20
Final:
Weight (0-100)? 30
Score earned? 95
Were scores shifted (1-yes, 2=no)? 1
Shift amount? 10
Total points=T00 / 100
Weighted score = 30.0 / 30
Homework:
Weight (0-100)? 50
Number of assignments? 3
Assignment 1 score? 14
Assignment 1 max? 15
Assignment 2 score? 17
Assignment 2 max? 20
Assignment 3 score? 19
Assignment 3 max? 25
How many sections did you attend? 5
Section points = 15 / 34
Total points = 65 / 94
Weighted score = 34.6 / 50
Overall percentage = 80.2
Your grade
will be at least: B
<< your custom grade message here >>
Next grading [Y/N]:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa1babbee-ce29-4977-814e-f4001afb45bc%2F8f5e5f42-03cb-497b-abeb-933b6564e5d9%2Fza9nyfm_processed.jpeg&w=3840&q=75)
Transcribed Image Text:assignments > 1, and the sum of the four weights will be exactly 100. The weight of each
category will be a non-negative number. Exam shifts will be ≥ 0.
The program should be developed in a modular way using the functions, parameters, and
returns value to eliminate redundancy.
Give meaningful names to variables, and use proper indentation and whitespace. Follow
Python's naming standards as specified in lecture. Localize variables when possible; declare
them in the smallest scope needed. Include meaningful comment headers at the top of your
program and at the start of each function. Limit line lengths to 100 chars. Consider to use some
customized functions to reduce the code redundancy and promote the code reusability.
Sample Run
Enter Student Name: Derrick
Enter Student ID: P1234567
Test:
Weight (0-100) ? 20
Score earned? 78
Were scores shifted (1-yes, 2=no)? 2
Total points =
78 / 1007
Weighted score = 15.6 / 20
Final:
Weight (0-100)? 30
Score earned? 95
Were scores shifted (1-yes, 2=no)? 1
Shift amount? 10
Total points=T00 / 100
Weighted score = 30.0 / 30
Homework:
Weight (0-100)? 50
Number of assignments? 3
Assignment 1 score? 14
Assignment 1 max? 15
Assignment 2 score? 17
Assignment 2 max? 20
Assignment 3 score? 19
Assignment 3 max? 25
How many sections did you attend? 5
Section points = 15 / 34
Total points = 65 / 94
Weighted score = 34.6 / 50
Overall percentage = 80.2
Your grade
will be at least: B
<< your custom grade message here >>
Next grading [Y/N]:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
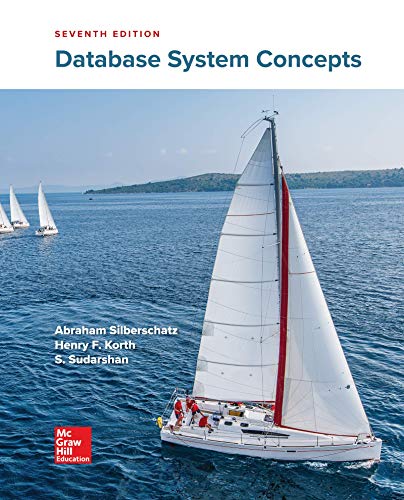
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
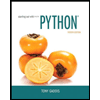
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
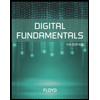
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
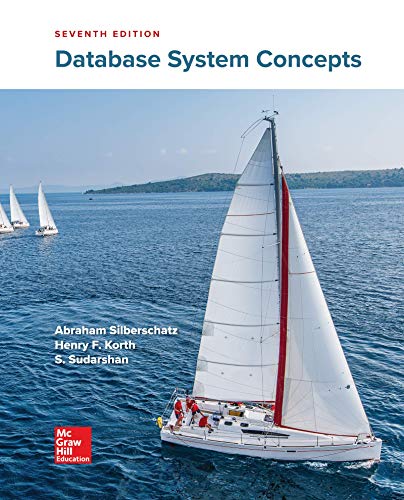
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
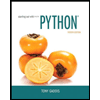
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
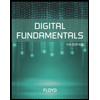
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
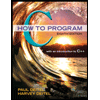
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
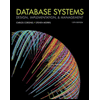
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
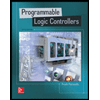
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education