Description Java you will create a backend class called SortableSet. A set, in computer science, is an unordered collection of unique objects. In our SortableSet, all of the objects in the set will also be immutable at the set level. This means that even if the data class used to create objects is not immutable, once they are in the set they are. We do this by making a deep copy of any objects being added to the set or returned from the set. The objects stored in the SortableSet must implement an interface called Sortable that provides a numeric sorting key and a deep copy method. SortableSet also takes advantage of two custom exception classes: DuplicateException and InvalidKeyException. Finally, the "sortable" part of SortableSet is implemented by a getSortedList method that makes a deep copy of every object in the set, saves them to an array, and then sorts them based on each object's sorting key. The sort algorithm used is a recursive radix sort modified to work with objects. Please see the attached image for details.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
![Details
The overall architecture of this lab is as shown here:
Sortable Set
- set: ArrayList<Sortable>
+ add(item: Sortable): void
+ delete(key: long): void
+ update(item: Sortable): void
+ get(key: long): Sortable
+ getKeys(): long[]
+ getSorted List(): Sortable[]
- sort(a: Sortable[]); void
- sort(a: Sortable[], digit: int, numDigits: int): void
- getMaxDigits(a: Sortable[]): int
sortByDigit(a: Sortable[], digit int): void
Sortable
<<interface>>
+ getSortKey(): long
+ getDeepCopy(): Sortable
Duplicate Exception
key: long
+ Duplicate Exception(message: String, key: long)
+ getKey(): long
InvalidKeyException
- key: long
+ InvalidKeyException(message: String, key: long)
+ getKey(): long
SomeConcreteClass
someldField: long
other fields required
+ full constructor
+ getters and setters
+ to String(): String
+ getSortKey(): long
+ getDeepCopy(): Sortable
java.lang. Exception
All classes must be named as shown in the above UML
diagram except the concrete class.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc3acae65-05d5-4940-8762-2c9a874e450a%2F0d4ea900-0fe5-449b-a2af-941efc74863a%2Fb18k2qy_processed.jpeg&w=3840&q=75)

The SortableSet class which refers to the one it is a generic class in Java that implements the Set interface. It provides a mechanism to store a collection of unique objects, where the order of the elements is determined by the Comparator or Comparable interface that is passed to the constructor. It also provides methods to add, remove, and search for elements in the set, as well as for sorting the elements in the set.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

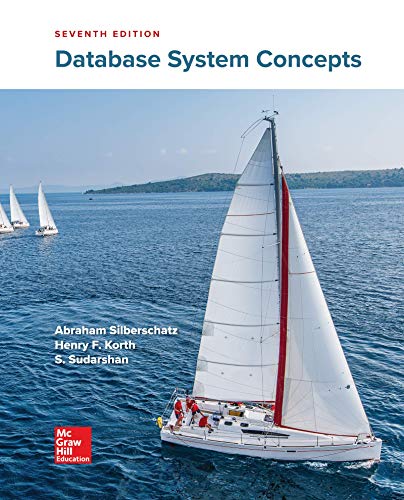
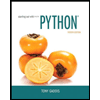
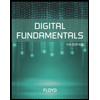
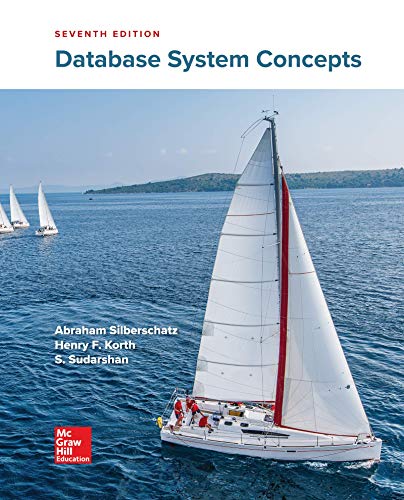
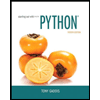
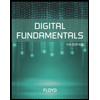
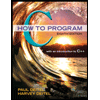
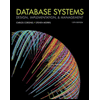
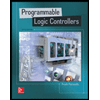