Define an abstrat super class called Employee that contains a name (String), a social security number (String) and the respective accessor and mutator functions. Include a two-parameter constructor. IH should also contain a teString method whose task is to return the employee's name and social security number (in that order) and an equals method (two employees are equal if they have the same social security nurnber, regardless of type). The Employee dass shoukl also dedare an abstract getCalculatedSalany method which will be used to calculate the total 1. salary in the derived classes Next, derive a cdass called HourlvĒrnlovee to store two data members the hourty rate (double) and the number of hours (double). Provide corresponding constructor and accessors and mutators. Create a method to compute the overtime amount The overtime is calculated when an employee works more than 40 hours, the extra hours (>40) are paid at one and one half times the employee's regular hourty rate. Include a toString function that must return a String containing the name, social security number, hourty rate, hours, overtime arnount and total weekly salary. It will need to call the super class toString Please include the data formatting as listed on the sample output. Next, derive a class called SalariedEunlovee to store two data members: the bonus rate (double) and the yearly salary (double). Provide corresponding constructor and accessors and mutators. Create a method to compute the bonus amount based on the bonus percentage and the yearty salary. Include a toString function that must return a String containing the name, social security number, bonus rate, base salary, bonus amount and total annual salary. It will need to call the super class teString, Please include the data formatting as listed on the sample output. 2. 3.
Define an abstrat super class called Employee that contains a name (String), a social security number (String) and the respective accessor and mutator functions. Include a two-parameter constructor. IH should also contain a teString method whose task is to return the employee's name and social security number (in that order) and an equals method (two employees are equal if they have the same social security nurnber, regardless of type). The Employee dass shoukl also dedare an abstract getCalculatedSalany method which will be used to calculate the total 1. salary in the derived classes Next, derive a cdass called HourlvĒrnlovee to store two data members the hourty rate (double) and the number of hours (double). Provide corresponding constructor and accessors and mutators. Create a method to compute the overtime amount The overtime is calculated when an employee works more than 40 hours, the extra hours (>40) are paid at one and one half times the employee's regular hourty rate. Include a toString function that must return a String containing the name, social security number, hourty rate, hours, overtime arnount and total weekly salary. It will need to call the super class toString Please include the data formatting as listed on the sample output. Next, derive a class called SalariedEunlovee to store two data members: the bonus rate (double) and the yearly salary (double). Provide corresponding constructor and accessors and mutators. Create a method to compute the bonus amount based on the bonus percentage and the yearty salary. Include a toString function that must return a String containing the name, social security number, bonus rate, base salary, bonus amount and total annual salary. It will need to call the super class teString, Please include the data formatting as listed on the sample output. 2. 3.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 2PE
Related questions
Question
Solve it.

Transcribed Image Text:Rough skeleton
Asg2YourName.java-
class BaseClass1
" provide implementation as per instructions
class DerivedClass#2
Il provide implementation as per instructions
class DerivedClass#3
{
l provide implementation as per instructions
class Class#4
l provide implementation as per instructions
public class Asg2YourName
public static void main(String args)
EmployeeList r= new Employeelist);
Ladd(new HourlMEmplovee("Jim", "400-00-0000", 15.60, 50)),
Laddinew SalanedEmplovee( Alex", "500-00-0000", 81090, 05)),
Laddinew SalanedEmplovee(Tim", "700-00-0000", 111577, 08)),
Ladd(new HoudxEmployee("Mark", "600-00-0000", 21.75, 60)),
Ladd(new HourdvEmploxee("Bill", "100-00-0000", 30.00, 45));
Ladd(new SalariedEmployee(Bob", "200-00-0000", 61090, .02));
Ladd(new SalariedEmplovee("Joe", "300-00-0000", 31690, .10));
Ladd(new HourdyEmploxee("Jane", "700-00-0000", 25.50, 60));
Ladd(new HourlxEmployee("Emma", "800-00-0000", 12.50, 30):
System.out.printia(r).
}
-End of Asq2YourName.java-

Transcribed Image Text:Instructions:
Define an abstrad super class called Enployee that contains a name (String), a social security number (String) and
the respective accessor and mutator functions. Include a two-parameter constructor. H should also conlain
a toString method whose task is to returm the ermployee's name and social security number (in thal order) and
an equals method (two employees are equal if they have the same social security nurnber, regardless of type).
The Employee dass shoukl also dedare an abstract getCalculatedSalax method which will be used to calculate the total
1.
salary in the derived classes
Next, derive a cdass called HourlyEruoloveg to store two data members the hourty rate (double) and the number of
hours (double) Provide corresponding constructor and accessors and mutators. Create a method to compute the
overtime amount The overtime is calculated when an employee works more than 40 hours, the extra hours (>40) are paid
at one and one half times the employee's regular hourty rate. Include a toString function that must return
a String containing the name, social security number, hourly rate, hours, overtime arnount and total weekly salary. It vwil
need to call the super class toString Please include the data formatting as listed on the sample output
Next, derive a class called SallariedEnuolovee to store two data members: the bonus rate (double) and the yearly
salary (double) Provide corresponding constructor and accessors and mutators. Create a method to compute the bonus
amount based on the bonus percentage and the yearly salary. Include a toString function that must return
a String containing the name, social security number, bonusS rate, base salary, bonus amount and total annual salary. It
will need to call the super class testring, Please include the data formating as listed on the sample output.
2.
3.
In order to hold al employees, create a class called Emploveelist that is able to hold any number of Eupl
references. EraploveeList should have an ArrayList of Employee as its data member. Create a method to provide the
capability to add an employee. An employee must not be added if it has a duplicate social security number. Display an
error message if a duplicate is found. Please see example of the error message below:
4.
es
ERROR - Employee Not Added: (display the name of the employee) Duplicate SSN (display the duplicate SSN).
Include a tostring function that displays the total number of employees on the list like the following
There are (number of employees) employees on the list
It should also display the entire Employee List Please include the data formatting as displayed on the sample output.
Sample Output with sample data:
ERROR - Employee Not Added John Duplicate SSN: 111-00-0000
There are 2 employees on the list.
Name: Albert SSN 111-00-0000 Hourly Rate: $10.50 Hours: 40.00
Salary: $420.00
Name: Peter SSN: 222-00-0000 Bonus Rate:10%
Salary $55,000.00
Overtime Amount: $0.00
Total Weekly
Base Salary: $50,000 00
Bonus Amount $5,000.00
Total Annual
You must use the provided data on the main method.
Please DO NOT CHANGE THE MAIN METHOD
All classes, methods, calculations and data elements must be properly documented as discussed in class.
Please submit only one file with the naming convention: Asg2YourName.java
Follow the rough skeleton below:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
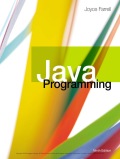
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
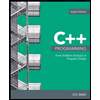
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
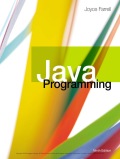
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
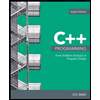
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
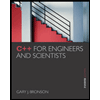
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
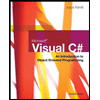
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,