Define a method findEmployeeTax() that takes two integer parameters as a person's salary and the number of dependents, and returns the person's tax percent as a double. The tax percent is returned as follows: Salary ≤ 1 dependent 2 - 5 dependents ≥ 6 dependents < 450000 0.15 0.05 0 450000 - 824999 0.25 0.15 0.15 ≥ 825000 0.4 0.25 0.25 Ex: If the input is 425000 0, then the output is: 0.15 import java.util.Scanner; public class TaxFinder { public static double findEmployeeTax(int salary, int numDepen) { double i; if (salary < 450000) { if(numDepen <= 1) i = 0.15; else if (numDepen >= 2 && numDepen <= 5) i = 0.05; else if (numDepen >= 6) i = 0; } else if (salary >= 450000 && salary <= 824999) { if(numDepen <= 1) i = 0.25; else if (numDepen >= 2 && numDepen <= 5) i = 0.15; else if (numDepen >= 6) i = 0.15; } else if (salary >= 825000){ if(numDepen <= 1) i = 0.4; else if (numDepen >= 2 && numDepen <= 5) i = 0.25; else if (numDepen >= 6) i = 0.25; } return i; } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int income; int userDependents; income = scnr.nextInt(); userDependents = scnr.nextInt(); System.out.println(findEmployeeTax(income, userDependents)); } }
Define a method findEmployeeTax() that takes two integer parameters as a person's salary and the number of dependents, and returns the person's tax percent as a double. The tax percent is returned as follows:
Salary | ≤ 1 dependent | 2 - 5 dependents | ≥ 6 dependents |
---|---|---|---|
< 450000 | 0.15 | 0.05 | 0 |
450000 - 824999 | 0.25 | 0.15 | 0.15 |
≥ 825000 | 0.4 | 0.25 | 0.25 |
Ex: If the input is 425000 0, then the output is:
0.15
import java.util.Scanner;
public class TaxFinder {
public static double findEmployeeTax(int salary, int numDepen) {
double i;
if (salary < 450000) {
if(numDepen <= 1)
i = 0.15;
else if (numDepen >= 2 && numDepen <= 5)
i = 0.05;
else if (numDepen >= 6)
i = 0;
}
else if (salary >= 450000 && salary <= 824999) {
if(numDepen <= 1)
i = 0.25;
else if (numDepen >= 2 && numDepen <= 5)
i = 0.15;
else if (numDepen >= 6)
i = 0.15;
}
else if (salary >= 825000){
if(numDepen <= 1)
i = 0.4;
else if (numDepen >= 2 && numDepen <= 5)
i = 0.25;
else if (numDepen >= 6)
i = 0.25;
}
return i;
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int income;
int userDependents;
income = scnr.nextInt();
userDependents = scnr.nextInt();
System.out.println(findEmployeeTax(income, userDependents));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

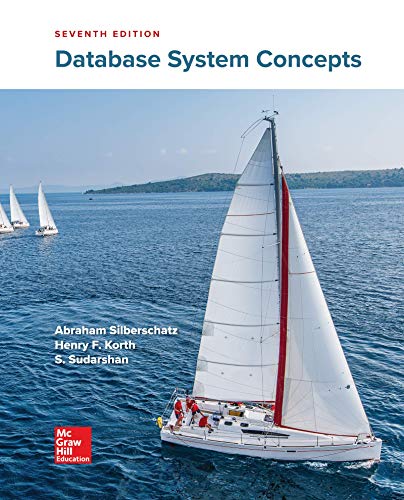
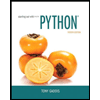
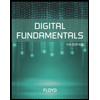
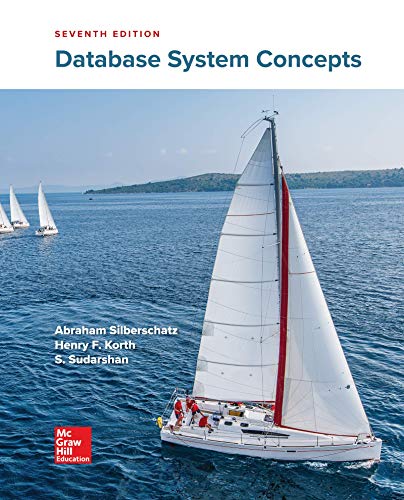
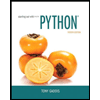
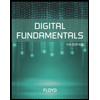
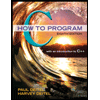
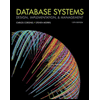
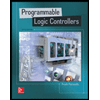