Define a function in R that takes a number n as a parameter and prints the factors of the number n in the output.
Define a function in R that takes a number n as a parameter and prints the factors of the number n in the output.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Also test the function for some sample values of n.

Transcribed Image Text:**Defining a Function in R to Print Factors**
To tackle this problem, we need to define a function in R that takes an integer `n` as a parameter. The function should calculate and print the factors of `n`.
### Steps to Implement the Function:
1. **Initialize the Function**: Start by creating a function in R using the `function()` keyword.
2. **Parameter Setup**: The function should take a single parameter `n`.
3. **Find Factors**: Use a loop or vectorization to determine the factors of `n`. A factor is a number that divides `n` without leaving a remainder.
4. **Print Factors**: Display these factors using a print or return statement within the function.
### Example Function:
Here is a simple example of how to define such a function in R:
```r
factorize <- function(n) {
factors <- NULL # Initialize an empty vector to store factors
for (i in 1:n) {
if (n %% i == 0) {
factors <- c(factors, i) # Add factor to the list
}
}
print(factors)
}
# Example Usage
factorize(12)
```
**Explanation**:
- **Loop**: The function utilizes a `for` loop from 1 to `n` to check each integer.
- **Modulo Operator**: `%%` is used to determine if the remainder of the division is zero.
- **Concatenation**: The factor is concatenated to the `factors` vector if it's valid.
- **Output**: Finally, it prints the list of factors.
This is a basic implementation and can be optimized for performance with larger numbers or altered to include additional features such as returning the factors instead of printing them directly.
Expert Solution

Step 1: R Code
Find_factors = function(n) {
print(paste("The factors of the",n,"are:"))
for(i in 1:n) {
if((n %% i) == 0) {
print(i)
}
}
}
Find_factors(15)
Find_factors(176)
Find_factors(120)
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
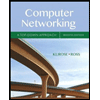
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
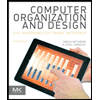
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
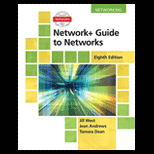
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
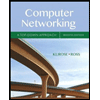
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
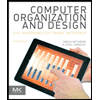
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
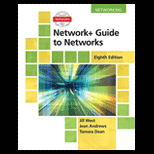
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
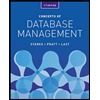
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
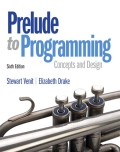
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
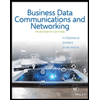
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY