Define a function cycle that takes in three functions f1, f2, f3, as arguments. cycle will return another function that should take in an integer argument n and return another function. That final function should take in an argument x and cycle through applying f1, f2, and f3 to x, depending on what n was. Here's the what the final function should do to x for a few values of n: ● ● ● ● n = 0, return x n = 1, apply f1 to x, or return f1(x) n = 2, apply f1 to x and then f2 to the result of that, or return f2(f1(x)) n = 3, apply f1 to x, f2 to the result of applying f1, and then f3 to the result of applying f2, or f3 (f2(f1(x))) ● n = 4, start the cycle again applying f1, then f2, then f3, then f1 again, or f1(f3(f2(f1(x)))) . And so forth.
Define a function cycle that takes in three functions f1, f2, f3, as arguments. cycle will return another function that should take in an integer argument n and return another function. That final function should take in an argument x and cycle through applying f1, f2, and f3 to x, depending on what n was. Here's the what the final function should do to x for a few values of n: ● ● ● ● n = 0, return x n = 1, apply f1 to x, or return f1(x) n = 2, apply f1 to x and then f2 to the result of that, or return f2(f1(x)) n = 3, apply f1 to x, f2 to the result of applying f1, and then f3 to the result of applying f2, or f3 (f2(f1(x))) ● n = 4, start the cycle again applying f1, then f2, then f3, then f1 again, or f1(f3(f2(f1(x)))) . And so forth.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Define a function cycle that takes in three functions f1, f2, f3, as arguments. cycle will return another function that
should take in an integer argument n and return another function. That final function should take in an argument x and cycle
through applying f1, f2, and f3 to x, depending on what n was. Here's the what the final function should do to x for a
few values of n:
• n = 0, return x
n = : 1, apply f1 to x, or return f1(x)
●
n = 2, apply f1 to x and then f2 to the result of that, or return f2(f1(x))
n = 3, apply f1 to x, f2 to the result of applying f1, and then f3 to the result of applying f2, or
f3 (f2(f1(x)))
•
n = 4, start the cycle again applying f1, then f2, then f3, then f1 again, or f1(f3 (f2(f1(x))))
. And so forth.
Hint: most of the work goes inside the most nested function.
Hint 2: given n, how many function calls are made on x ?
Hint 3: for help with how to cycle through the functions (i.e., how to go back to applying f1 as your outermost function call
when n = 4), consider looking at this python tutor demo which has similar cycling behaviour.
def cycle(f1, f2, f3):
""" Returns a function that is itself a higher order function
>>> def add1(x):
return x + 1
>>> def times2(x):
return x * 2
☐☐☐
III
>>> def add3(x):
return x + 3
>>> my_cycle
>>> identity
>>> identity (5)
5
4
=
=
cycle (add1, times2, add3)
my_cycle (0)
>>>add_one_then_double = my_cycle (2)
>>>add_one_then_double (1)
>>>do_all_functions =
>>>do_all_functions (2)
my_cycle (3)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
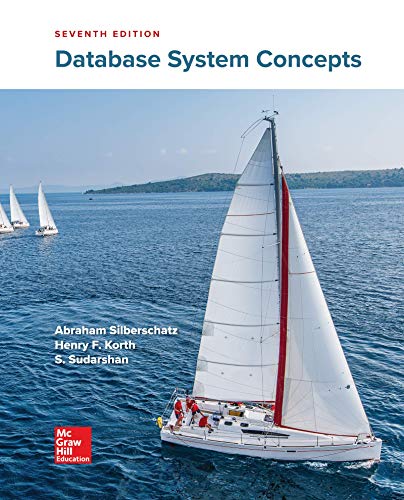
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
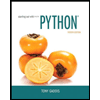
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
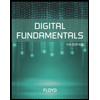
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
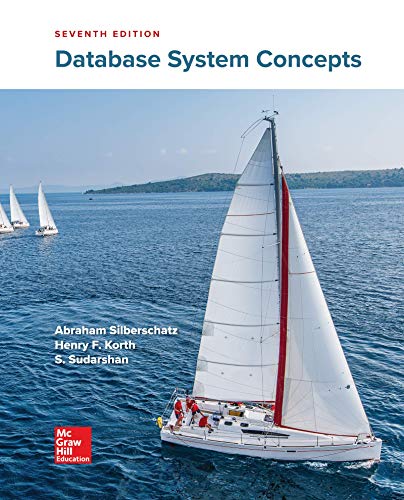
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
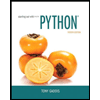
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
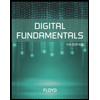
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
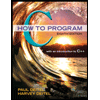
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
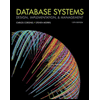
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
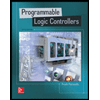
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education