Define a class StatePair with two generic types (Type1 and Type2), a constructor, mutators, accessors, and a printinfo() method. Three ArrayLists have been pre-filled with StatePair data in main(): • ArrayList> fillArray1(ArrayList> statePair: Scanner inFS) { } } } StatePair pair; int intValue; String stringValue; while (inFS.hasNextLine()) { inFS.nextInt (); public static ArrayList> fillArray2(ArrayList> statePairs, ⠀⠀ Scanner inFS) { StatePair pair; } String>> zipCodeState: Contains ZIP code/state abbreviation pairs string>> abbrevstate: Contains state abbreviation/state name pairs Integer>> statePopulation: Contains state name/population pairs return statePairs; intValue stringValue inFS.next(); pair = new StatePair (intValue, stringValue); statePairs.add(pair); public static ArrayList> fillArray3(ArrayList> statePair: Scanner inFS) { StatePair pair; String stringValuel; String stringValue2; while (inFS.hasNextLine()) { stringValue1= InFS.next(); inFS.nextLine(); stringValue2= inFS.nextLine(); pair = new StatePair (stringValuel, stringValue2); statePairs.add(pair); return statePairs; String stringValue; int intValue; while (inFS.hasNextLine()) { stringValue = inFS.nextLine(); intValue inFS.nextInt(); } return statePairs; inFS.nextLine(); pair = new StatePair (stringValue, intValue); statePairs.add(pair); public static void main(String[] args) throws IOException { Scanner scnr = new Scanner(System.in); FileInputStream fileByteStream = null; // File input stream Scanner inFS = null; // Scanner object int myZipCode; int i; // ZIP code- state abbrev. pairs ArrayList> zipCodeState = new ArrayList>(); // state abbrev. state name pairs ArrayList> abbrevState = new ArrayList>(); // state name population pairs ArrayList> statePopulation = new ArrayList>(); // FELL the three ArrayLists // Try to open zip_code_state.txt file fileByteStream = new FileInputStream("zip_code_state.txt"); inFS = new Scanner (fileByteStream); zipCodeState = fillArray1(zipCodeState, inFS); fileByteStream.close(); // close() may throw IOException if fails // Try to open abbreviation_state.txt file fileByteStream = new FileInputStream("abbreviation_state.txt"); inFS new Scanner (fileByteStream); abbrevState = fillArray2(abbrevState, inFS); fileByteStream.close(); // Try to open state_population. txt file fileByteStream = new FileInputStream("state_population.txt"); inFS = new Scanner (fileByteStream); statePopulation = fillArray3(statePopulation, inFS); fileByteStream.close(); // Read in ZIP code from user myZipCode= scnr.nextInt(); } for (i = 0; i < zipCodeState.size(); ++i) { // TODO: Using ZIP code, find state abbreviation } for (i = 0; i , Type2 extends Comparable> private Typel valuel; private Type2 value2; // TODO: Define a constructor, mutators, and accessors for StatePair 8 // TODO: Define printInfo() method 9 H Load default template..
Define a class StatePair with two generic types (Type1 and Type2), a constructor, mutators, accessors, and a printinfo() method. Three ArrayLists have been pre-filled with StatePair data in main(): • ArrayList> fillArray1(ArrayList> statePair: Scanner inFS) { } } } StatePair pair; int intValue; String stringValue; while (inFS.hasNextLine()) { inFS.nextInt (); public static ArrayList> fillArray2(ArrayList> statePairs, ⠀⠀ Scanner inFS) { StatePair pair; } String>> zipCodeState: Contains ZIP code/state abbreviation pairs string>> abbrevstate: Contains state abbreviation/state name pairs Integer>> statePopulation: Contains state name/population pairs return statePairs; intValue stringValue inFS.next(); pair = new StatePair (intValue, stringValue); statePairs.add(pair); public static ArrayList> fillArray3(ArrayList> statePair: Scanner inFS) { StatePair pair; String stringValuel; String stringValue2; while (inFS.hasNextLine()) { stringValue1= InFS.next(); inFS.nextLine(); stringValue2= inFS.nextLine(); pair = new StatePair (stringValuel, stringValue2); statePairs.add(pair); return statePairs; String stringValue; int intValue; while (inFS.hasNextLine()) { stringValue = inFS.nextLine(); intValue inFS.nextInt(); } return statePairs; inFS.nextLine(); pair = new StatePair (stringValue, intValue); statePairs.add(pair); public static void main(String[] args) throws IOException { Scanner scnr = new Scanner(System.in); FileInputStream fileByteStream = null; // File input stream Scanner inFS = null; // Scanner object int myZipCode; int i; // ZIP code- state abbrev. pairs ArrayList> zipCodeState = new ArrayList>(); // state abbrev. state name pairs ArrayList> abbrevState = new ArrayList>(); // state name population pairs ArrayList> statePopulation = new ArrayList>(); // FELL the three ArrayLists // Try to open zip_code_state.txt file fileByteStream = new FileInputStream("zip_code_state.txt"); inFS = new Scanner (fileByteStream); zipCodeState = fillArray1(zipCodeState, inFS); fileByteStream.close(); // close() may throw IOException if fails // Try to open abbreviation_state.txt file fileByteStream = new FileInputStream("abbreviation_state.txt"); inFS new Scanner (fileByteStream); abbrevState = fillArray2(abbrevState, inFS); fileByteStream.close(); // Try to open state_population. txt file fileByteStream = new FileInputStream("state_population.txt"); inFS = new Scanner (fileByteStream); statePopulation = fillArray3(statePopulation, inFS); fileByteStream.close(); // Read in ZIP code from user myZipCode= scnr.nextInt(); } for (i = 0; i < zipCodeState.size(); ++i) { // TODO: Using ZIP code, find state abbreviation } for (i = 0; i , Type2 extends Comparable> private Typel valuel; private Type2 value2; // TODO: Define a constructor, mutators, and accessors for StatePair 8 // TODO: Define printInfo() method 9 H Load default template..
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Java (Generic Types) - Zip Code and Population
![Define a class statePair with two generic types (Type1 and Type2), a constructor, mutators, accessors, and a printinfo() method. Three
ArrayLists have been pre-filled with StatePair data in main():
• ArrayList<StatePair<Integer,
• ArrayList<StatePair<string,
• ArrayList<StatePair<string,
.
Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the ArrayList zipCodeState. Then use the state
abbreviation to retrieve the state name from the ArrayList abbrevState. Lastly, use the state name to retrieve the correct state
name/population pair from the ArrayList statePopulation and output the pair.
Ex: If the input is:
21044
the output is:
Maryland: 6079602
1 import java.util.Scanner;
2 import java.io.FileInputStream;
3 import java.io.IOException;
4 import java.util.ArrayList;
5
6 public class StatePopulations
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
187
108
109}
4
5
public static ArrayList<StatePair<Integer, String>> fillArray1(ArrayList<StatePair<Integer, String>> statePair:
Scanner inFS) {
}
StatePair<Integer, String> pair;
int intValue;
String stringValue;
while (inFS.hasNextLine()) {
}
intValue
inFS.nextInt();
stringValue = inFS.next();
pair= new StatePair<Integer, String> (intValue, stringValue);
statePairs.add(pair);
}
return statePairs;
}
public static ArrayList<StatePair<String, String>> fillArray2(ArrayList<StatePair<String, String>> statePairs,
⠀⠀⠀⠀ Scanner inFS) {
StatePair<string, String> pair;
string>> zipCodestate: Contains ZIP code/state abbreviation pairs
string>> abbrevstate: Contains state abbreviation/state name pairs
Integer>> statePopulation: Contains state name/population pairs
String stringValuel;
String stringValue2;
while (inFS.hasNextLine()) {
stringValue1= inFS.next();
inFS.nextLine();
stringValue2= inFS.nextLine();
pair = new StatePair<String, String> (stringValuel, stringValue2);
statePairs.add(pair);
}
return statePairs;
public static ArrayList<StatePair<String, Integer>> fillArray3(ArrayList<StatePair<String, Integer>> statePair:
Scanner inFS) {
StatePair<string, Integer> pair;
String stringValue;
int intValue;
while (inFS.hasNextLine()) {
stringValue = inFS.nextLine();
intValue inFS.nextInt ();
inFS.nextLine();
pair = new StatePair<String, Integer> (stringValue, intValue);
statePairs.add(pair);
return statePairs;
public static void main(String[] args) throws IOException {
Scanner scnr = new Scanner(System.in);
FileInputStream fileByteStream = null; // File input stream
Scanner inFS = null;
// Scanner object
int nyZipCode;
int i;
// ZIP code- state abbrev. pairs.
ArrayList<StatePair<Integer, String>> zipCodeState = new ArrayList<StatePair<Integer, String>>();
// state abbrev. state name pairs
ArrayList<StatePair<String, String>> abbrevState = new ArrayList<StatePair<String, String>>();
// state name population pairs
ArrayList<StatePair<String, Integer>> statePopulation = new ArrayList<StatePair<String, Integer>>();
// FELL the three ArrayLists
// Try to open zip_code_state.txt file
fileByteStream = new FileInputStream("zip_code_state.txt");
inFS new Scanner (fileByteStream);
zipCodeState = fillArray1(zipCodeState, inFS);
fileByteStream.close(); // close() may throw IOException if fails
// Try to open abbreviation_state.txt file
fileByteStream = new FileInputStream("abbreviation_state.txt");
inFS = new Scanner (fileByteStream);
abbrevState = fillArray2(abbrevState, inFS);
fileByteStream.close();
// Try to open state_population.txt file
fileByteStream = new FileInputStream("state_population.txt");
inFS = new Scanner (fileByteStream);
statePopulation = fillArray3(statePopulation, inFS);
fileByteStream.close();
// Read in ZIP code from user
myZipCode= scnr.nextInt();
for (i = 0; i < zipCodeState.size(); ++i) {
// TODO: Using ZIP code, find state abbreviation
}
for (i = 0; i <abbrevState.size(); ++i) {
I // TODO: Using state abbreviation, find state name
}
for (i = 0; i < statePopulation.size(); ++i) {
// TODO: Using state name, find population. Print pair info.
}
Current file: StatePair.java →
1 public class StatePair <Typel extends Comparable<Type1>, Type2 extends Comparable<Type2>> {
private Typel valuel;
2
private Type2 value2;
// TODO: Define a constructor, mutators, and accessors.
for StatePair
6
7
8 // TODO: Define printInfo() method
9}
Load default template.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd863c081-8226-42e9-a15c-9cd471d16ef3%2Fe5d2b3a9-42c2-4707-bf9f-622f032026bc%2Fqvfci2p_processed.png&w=3840&q=75)
Transcribed Image Text:Define a class statePair with two generic types (Type1 and Type2), a constructor, mutators, accessors, and a printinfo() method. Three
ArrayLists have been pre-filled with StatePair data in main():
• ArrayList<StatePair<Integer,
• ArrayList<StatePair<string,
• ArrayList<StatePair<string,
.
Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the ArrayList zipCodeState. Then use the state
abbreviation to retrieve the state name from the ArrayList abbrevState. Lastly, use the state name to retrieve the correct state
name/population pair from the ArrayList statePopulation and output the pair.
Ex: If the input is:
21044
the output is:
Maryland: 6079602
1 import java.util.Scanner;
2 import java.io.FileInputStream;
3 import java.io.IOException;
4 import java.util.ArrayList;
5
6 public class StatePopulations
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
187
108
109}
4
5
public static ArrayList<StatePair<Integer, String>> fillArray1(ArrayList<StatePair<Integer, String>> statePair:
Scanner inFS) {
}
StatePair<Integer, String> pair;
int intValue;
String stringValue;
while (inFS.hasNextLine()) {
}
intValue
inFS.nextInt();
stringValue = inFS.next();
pair= new StatePair<Integer, String> (intValue, stringValue);
statePairs.add(pair);
}
return statePairs;
}
public static ArrayList<StatePair<String, String>> fillArray2(ArrayList<StatePair<String, String>> statePairs,
⠀⠀⠀⠀ Scanner inFS) {
StatePair<string, String> pair;
string>> zipCodestate: Contains ZIP code/state abbreviation pairs
string>> abbrevstate: Contains state abbreviation/state name pairs
Integer>> statePopulation: Contains state name/population pairs
String stringValuel;
String stringValue2;
while (inFS.hasNextLine()) {
stringValue1= inFS.next();
inFS.nextLine();
stringValue2= inFS.nextLine();
pair = new StatePair<String, String> (stringValuel, stringValue2);
statePairs.add(pair);
}
return statePairs;
public static ArrayList<StatePair<String, Integer>> fillArray3(ArrayList<StatePair<String, Integer>> statePair:
Scanner inFS) {
StatePair<string, Integer> pair;
String stringValue;
int intValue;
while (inFS.hasNextLine()) {
stringValue = inFS.nextLine();
intValue inFS.nextInt ();
inFS.nextLine();
pair = new StatePair<String, Integer> (stringValue, intValue);
statePairs.add(pair);
return statePairs;
public static void main(String[] args) throws IOException {
Scanner scnr = new Scanner(System.in);
FileInputStream fileByteStream = null; // File input stream
Scanner inFS = null;
// Scanner object
int nyZipCode;
int i;
// ZIP code- state abbrev. pairs.
ArrayList<StatePair<Integer, String>> zipCodeState = new ArrayList<StatePair<Integer, String>>();
// state abbrev. state name pairs
ArrayList<StatePair<String, String>> abbrevState = new ArrayList<StatePair<String, String>>();
// state name population pairs
ArrayList<StatePair<String, Integer>> statePopulation = new ArrayList<StatePair<String, Integer>>();
// FELL the three ArrayLists
// Try to open zip_code_state.txt file
fileByteStream = new FileInputStream("zip_code_state.txt");
inFS new Scanner (fileByteStream);
zipCodeState = fillArray1(zipCodeState, inFS);
fileByteStream.close(); // close() may throw IOException if fails
// Try to open abbreviation_state.txt file
fileByteStream = new FileInputStream("abbreviation_state.txt");
inFS = new Scanner (fileByteStream);
abbrevState = fillArray2(abbrevState, inFS);
fileByteStream.close();
// Try to open state_population.txt file
fileByteStream = new FileInputStream("state_population.txt");
inFS = new Scanner (fileByteStream);
statePopulation = fillArray3(statePopulation, inFS);
fileByteStream.close();
// Read in ZIP code from user
myZipCode= scnr.nextInt();
for (i = 0; i < zipCodeState.size(); ++i) {
// TODO: Using ZIP code, find state abbreviation
}
for (i = 0; i <abbrevState.size(); ++i) {
I // TODO: Using state abbreviation, find state name
}
for (i = 0; i < statePopulation.size(); ++i) {
// TODO: Using state name, find population. Print pair info.
}
Current file: StatePair.java →
1 public class StatePair <Typel extends Comparable<Type1>, Type2 extends Comparable<Type2>> {
private Typel valuel;
2
private Type2 value2;
// TODO: Define a constructor, mutators, and accessors.
for StatePair
6
7
8 // TODO: Define printInfo() method
9}
Load default template.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
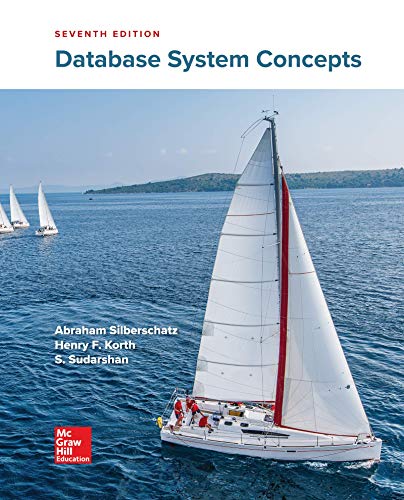
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
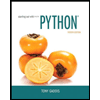
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
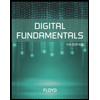
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
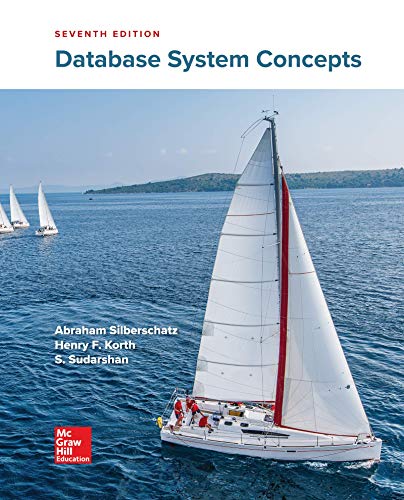
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
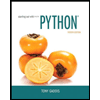
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
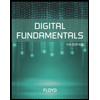
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
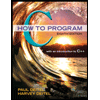
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
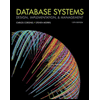
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
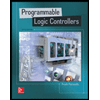
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education