Define a class Money with proper constructors, accessors, mutators, and other member functions The class will be storing money information using coins values only The class should have following data members (Quarter, Dime, Nickel, Penny) Write A function to print out the object money info expressed in dollars and Cents only Overload the + operator, so that adding two objects of this class works
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Part II:
Define a class Money with proper constructors, accessors, mutators, and other member functions
The class will be storing money information using coins values only
The class should have following data members (Quarter, Dime, Nickel, Penny)
Write A function to print out the object money info expressed in dollars and Cents only
Overload the + operator, so that adding two objects of this class works
Example: if you have following money objects
$2.99 could be constructed based on available coins Money. m1(11,1,2,4)
m1could also expressed as Money m2(10,4,1,4)
(m1 + m2).print(); should display $4.98
Overload the + operator, so that adding two objects of this class works
Class money
{
Public:
money();
money( int, int, int, int);
void printMoney();
money operator+( money m)
{
// add addition
}
// accessors
int getquarter();
int getdime ();
int getnickel();
int getpenny();
// mutators
Vopid setquarter();
Void setdime ();
Void setnickel();
void setpenny();
private:
int quarter;
int dime;
int nickel;
int penny;
}
money::money()
{
}
money::money(int penny=0, int nickel =0)
{
This->penny=penny
This-> nickel = nickel
}
Void money:: printMoney ()
{
Int dollars;
Int cents:
Int pennies= quarter * 25 + dime * 10 + nickel * 5 + penny;
dollars = pennies/ 100;
cents = pennies % 100;
cout << “ Money is: $” << dollars << “.” << cents<< endl;
}
int money::getquarter()
{
return quarter;
}
int money::getdime ()
{
return dime;
}
int money::getnickel()
{
return nickel;
}
int money::getpenny()
{
return penny;
}
// mutators
Vopid money::setquarter( int quarter)
{
this-> quarter = quarter;
}
Void money::setdime ( int dime)
{
this-> dime = dime;
}
Void money::setnickel( int nickel)
{
this-> nickel = nickel;
}
void money::setpenny(int penny){
this->penny=penny;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

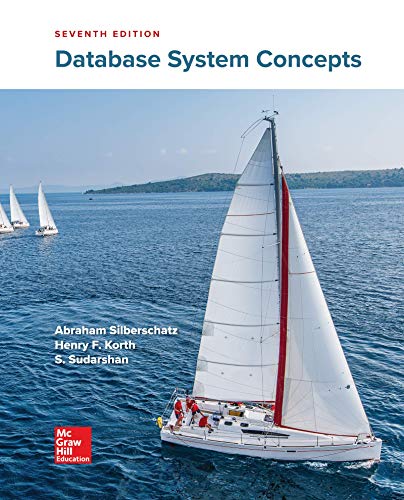
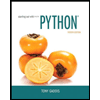
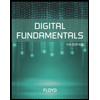
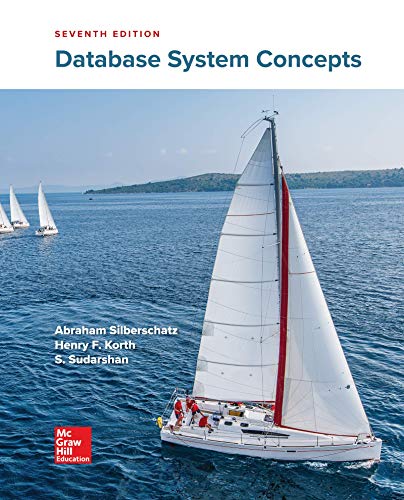
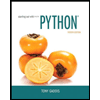
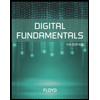
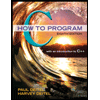
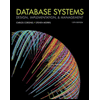
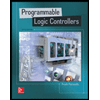