def weighted_mse_grad(w, X, y, V): """ Calculate gradient of the weight MSE loss in the given point w Parameters ---------- w: array_like Current point, in which we want to evaluate gradient (vector with k+1 elements) X: array_like Design matrix with N rows and k+1 columns y: array_like Vector with N observations of the target variable V: array_like Diagonal matrix N x N, with the weights Returns ---------- out: ndarray vector of gradient, k+1 """ # your code here # TEST weighted_mse_grad() w = np.zeros(5) X = np.random.randn(10, 5) y = np.ones(10) V = np.diag(np.ones(10)) L_grad = weighted_mse_grad(w, X, y, V) assert(L_grad.shape == (5,)) w = np.zeros(5) X = np.eye(5) y = np.ones(5) V = np.diag(np.ones(5)) L_grad = weighted_mse_grad(w, X, y, V) assert(L_grad.shape == (5,)), 'The shape of the output on the test case is wrong' assert(np.allclose(5 * L_grad,- y * 2)), 'The values of the output on the test case is wrong'
def weighted_mse_grad(w, X, y, V):
"""
Calculate gradient of the weight MSE loss in the given point w
Parameters
----------
w: array_like
Current point, in which we want to evaluate gradient (
X: array_like
Design matrix with N rows and k+1 columns
y: array_like
Vector with N observations of the target variable
V: array_like
Diagonal matrix N x N, with the weights
Returns
----------
out: ndarray
vector of gradient, k+1
"""
# your code here
# TEST weighted_mse_grad()
w = np.zeros(5)
X = np.random.randn(10, 5)
y = np.ones(10)
V = np.diag(np.ones(10))
L_grad = weighted_mse_grad(w, X, y, V)
assert(L_grad.shape == (5,))
w = np.zeros(5)
X = np.eye(5)
y = np.ones(5)
V = np.diag(np.ones(5))
L_grad = weighted_mse_grad(w, X, y, V)
assert(L_grad.shape == (5,)), 'The shape of the output on the test case is wrong'
assert(np.allclose(5 * L_grad,- y * 2)), 'The values of the output on the test case is wrong'


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

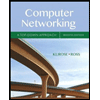
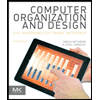
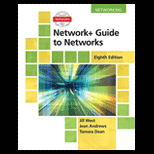
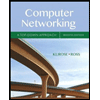
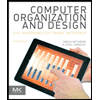
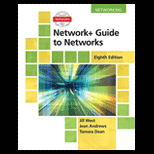
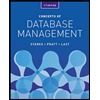
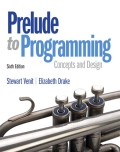
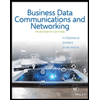