Data compression is a very important research topic in Computer Science that enabled the creation of a variety of products over the last years. It is especially helpful when we have to store or transmit large quantities of data. As a small example, remember our previous lab where we calculate the storage requirements for a single image? Let's extend that for a single video. If we have a colored video at Full-HD resolution (AKA 1080p), there will be 1920x1080x8x3 bits per image. Movies are typically recorded at 24 frames (images) per second, so if we have one hour of content, we will have 1920x1080x8x3x24x60x60, which would result in 4.299817e+12 bits, which is roughly 500GB (and we are not even considering audio here too!). Many of those bits of data are inherently redundant -- continuing with the video example -- the changes from one frame to the next are typically very small since they capture 1/24th of a second. Similarly, the frames themselves present a lot of redundancy as we might have sequences of pixels with the same color value. One common way of compressing the data is commonly referred as Run-Length Encoding (RLE), which consists of counting how many times the same symbol appears in the sequence, then encode it as [symbol][repetitions]. For example, suppose we are trying to transmit the following sequence: 1111000111 Its RLE would be: [14, 03, 13] Since 1 appears 4 times in a row, 0 appears 3 times, then 1 appears again 3 times. Requirements Write a function called RLE () that takes as input one string to be encoded and returns the encoded version of that string using the scheme presented above. Your function should return a list where each entry in the list is a string representing a pair of symbols and its number of repetitions (as they appear when scanning the input string from left to right). Your code should be able to handle any symbol, not only 1 and 0. For example ###000ccc is a valid input that would return ['#3', '03', 'c3'] In your main program, print the encoded sequence. You can use the assert statements to test that your function behaves as expected.
Data compression is a very important research topic in Computer Science that enabled the creation of a variety of products over the last years. It is especially helpful when we have to store or transmit large quantities of data. As a small example, remember our previous lab where we calculate the storage requirements for a single image? Let's extend that for a single video. If we have a colored video at Full-HD resolution (AKA 1080p), there will be 1920x1080x8x3 bits per image. Movies are typically recorded at 24 frames (images) per second, so if we have one hour of content, we will have 1920x1080x8x3x24x60x60, which would result in 4.299817e+12 bits, which is roughly 500GB (and we are not even considering audio here too!). Many of those bits of data are inherently redundant -- continuing with the video example -- the changes from one frame to the next are typically very small since they capture 1/24th of a second. Similarly, the frames themselves present a lot of redundancy as we might have sequences of pixels with the same color value. One common way of compressing the data is commonly referred as Run-Length Encoding (RLE), which consists of counting how many times the same symbol appears in the sequence, then encode it as [symbol][repetitions]. For example, suppose we are trying to transmit the following sequence: 1111000111 Its RLE would be: [14, 03, 13] Since 1 appears 4 times in a row, 0 appears 3 times, then 1 appears again 3 times. Requirements Write a function called RLE () that takes as input one string to be encoded and returns the encoded version of that string using the scheme presented above. Your function should return a list where each entry in the list is a string representing a pair of symbols and its number of repetitions (as they appear when scanning the input string from left to right). Your code should be able to handle any symbol, not only 1 and 0. For example ###000ccc is a valid input that would return ['#3', '03', 'c3'] In your main program, print the encoded sequence. You can use the assert statements to test that your function behaves as expected.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Compress a signal in python
![Data compression is a very important research topic in Computer Science that enabled the creation of a variety of products over the last
years. It is especially helpful when we have to store or transmit large quantities of data.
As a small example, remember our previous lab where we calculate the storage requirements for a single image? Let's extend that for a
single video. If we have a colored video at Full-HD resolution (AKA 1080p), there will be 1920x1080x8x3 bits per image. Movies are typically
recorded at 24 frames (images) per second, so if we have one hour of content, we will have 1920x1080x8x3x24x60x60, which would result
in 4.299817e+12 bits, which is roughly 500GB (and we are not even considering audio here too!).
Many of those bits of data are inherently redundant -- continuing with the video example -- the changes from one frame to the next are
typically very small since they capture 1/24th of a second. Similarly, the frames themselves present a lot of redundancy as we might have
sequences of pixels with the same color value.
One common way of compressing the data is commonly referred as Run-Length Encoding (RLE), which consists of counting how many
times the same symbol appears in the sequence, then encode it as [symbol][repetitions].
For example, suppose we are trying to transmit the following sequence:
1111000111
Its RLE would be:
[14, 03, 13]
Since 1 appears 4 times in a row, 0 appears 3 times, then 1 appears again 3 times.
Requirements
Write a function called RLE () that takes as input one string to be encoded and returns the encoded version of that string using the scheme
presented above.
Your function should return a list where each entry in the list is a string representing a pair of symbols and its number of repetitions (as
they appear when scanning the input string from left to right).
Your code should be able to handle any symbol, not only 1 and 0. For example ###000ccc is a valid input that would return ['#3',
'03', 'c3']
In your main program, print the encoded sequence.
You can use the assert statements to test that your function behaves as expected.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3524954f-433f-4919-9862-20e9f2015c73%2F139fb9c3-cd82-4957-bc13-f2215e31d922%2Ffo3mnqa_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Data compression is a very important research topic in Computer Science that enabled the creation of a variety of products over the last
years. It is especially helpful when we have to store or transmit large quantities of data.
As a small example, remember our previous lab where we calculate the storage requirements for a single image? Let's extend that for a
single video. If we have a colored video at Full-HD resolution (AKA 1080p), there will be 1920x1080x8x3 bits per image. Movies are typically
recorded at 24 frames (images) per second, so if we have one hour of content, we will have 1920x1080x8x3x24x60x60, which would result
in 4.299817e+12 bits, which is roughly 500GB (and we are not even considering audio here too!).
Many of those bits of data are inherently redundant -- continuing with the video example -- the changes from one frame to the next are
typically very small since they capture 1/24th of a second. Similarly, the frames themselves present a lot of redundancy as we might have
sequences of pixels with the same color value.
One common way of compressing the data is commonly referred as Run-Length Encoding (RLE), which consists of counting how many
times the same symbol appears in the sequence, then encode it as [symbol][repetitions].
For example, suppose we are trying to transmit the following sequence:
1111000111
Its RLE would be:
[14, 03, 13]
Since 1 appears 4 times in a row, 0 appears 3 times, then 1 appears again 3 times.
Requirements
Write a function called RLE () that takes as input one string to be encoded and returns the encoded version of that string using the scheme
presented above.
Your function should return a list where each entry in the list is a string representing a pair of symbols and its number of repetitions (as
they appear when scanning the input string from left to right).
Your code should be able to handle any symbol, not only 1 and 0. For example ###000ccc is a valid input that would return ['#3',
'03', 'c3']
In your main program, print the encoded sequence.
You can use the assert statements to test that your function behaves as expected.
![Hints
1. Use a variable to keep track of what symbol you are currently look for, and then branch if you encounter a different one.
2. Use string concatenation and type conversion to form the pair that will be inserted into the output list
422020.2684366.qx3zqy7
LAB
ACTIVITY
1 def RLE(...):
L2345678
4.22.1: LAB: Compress a signal
9
10
5 if __name__ ==
11
12
13
# Insert your code here
'__main__':
assert RLE('1111000111')
['14', '03', '13']
#Insert other tests here to further evaluate your code
=
input()
RLE(...)
print (...)
main.py
==
Load default](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3524954f-433f-4919-9862-20e9f2015c73%2F139fb9c3-cd82-4957-bc13-f2215e31d922%2Fmfagj_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Hints
1. Use a variable to keep track of what symbol you are currently look for, and then branch if you encounter a different one.
2. Use string concatenation and type conversion to form the pair that will be inserted into the output list
422020.2684366.qx3zqy7
LAB
ACTIVITY
1 def RLE(...):
L2345678
4.22.1: LAB: Compress a signal
9
10
5 if __name__ ==
11
12
13
# Insert your code here
'__main__':
assert RLE('1111000111')
['14', '03', '13']
#Insert other tests here to further evaluate your code
=
input()
RLE(...)
print (...)
main.py
==
Load default
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
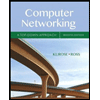
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
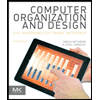
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
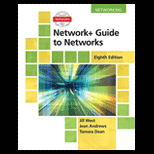
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
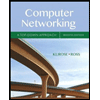
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
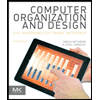
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
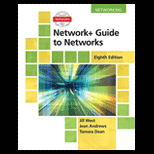
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
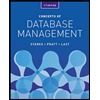
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
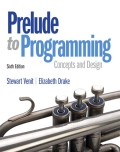
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
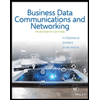
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY