Creation of a PHP and MySQL comment and response system and javascript A system that allows users to comment on your blog post and get responses from other users
Creation of a PHP and MySQL comment and response system and javascript A system that allows users to comment on your blog post and get responses from other users
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Creation of a PHP and MySQL comment and response system and javascript
A system that allows users to comment on your blog post and get responses from other users
Expert Solution

Step 1
Source Code:
index.html:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Comments System</title>
<link href="style.css" rel="stylesheet" type="text/css">
<link href="comments.css" rel="stylesheet" type="text/css">
</head>
<body>
<nav class="navtop">
<div>
<h1>PHP, MYSQL and JS using Comments System</h1>
</div>
</nav>
<div class="content_home">
<h2>Bartleby</h2>
<p>Bartleby is the best expert platform for students. Keep Bartleby. Thank you...</p>
</div>
<div class="comments"></div>
<script>
const comments_page_id = 1;
fetch("comments.php?page_id=" + comments_page_id).then(response => response.text()).then(data => {
document.querySelector(".comments").innerHTML = data;
document.querySelectorAll(".comments .write_comment_btn, .comments .reply_comment_btn").forEach(element => {
element.onclick = event => {
event.preventDefault();
document.querySelectorAll(".comments .write_comment").forEach(element => element.style.display = 'none');
document.querySelector("div[data-comment-id='" + element.getAttribute("data-comment-id") + "']").style.display = 'block';
document.querySelector("div[data-comment-id='" + element.getAttribute("data-comment-id") + "'] input[name='name']").focus();
};
});
document.querySelectorAll(".comments .write_comment form").forEach(element => {
element.onsubmit = event => {
event.preventDefault();
fetch("comments.php?page_id=" + comments_page_id, {
method: 'POST',
body: new FormData(element)
}).then(response => response.text()).then(data => {
element.parentElement.innerHTML = data;
});
};
});
});
</script>
</body>
</html>
comments.php:
<?php
$db_Host = 'localhost';
$db_User = 'root';
$db_Pass = '';
$db_Name = 'phpcomments';
try {
$pdo = new PDO('mysql:host=' . $db_Host . ';dbname=' . $db_Name . ';charset=utf8', $db_User, $db_Pass);
} catch (PDOException $exception) {
exit('Failed to connect to database!');
}
function time_elapsed_string($date_time, $full = false) {
$current = new DateTime;
$later = new DateTime($date_time);
$diff = $current->diff($later);
$diff->w = floor($diff->d / 7);
$diff->d -= $diff->w * 7;
$string = array('y' => 'year', 'm' => 'month', 'w' => 'week', 'd' => 'day', 'h' => 'hour', 'i' => 'minute', 's' => 'second');
foreach ($string as $k => &$v) {
if ($diff->$k) {
$v = $diff->$k . ' ' . $v . ($diff->$k > 1 ? 's' : '');
} else {
unset($string[$k]);
}
}
if (!$full) $string = array_slice($string, 0, 1);
return $string ? implode(', ', $string) . ' later' : 'just current';
}
function show_comments($comments, $parent_id = -1) {
$html = '';
if ($parent_id != -1) {
array_multisort(array_column($comments, 'submit_date'), SORT_ASC, $comments);
}
foreach ($comments as $comment) {
if ($comment['parent_id'] == $parent_id) {
$html .= '
<div class="comment">
<div>
<h3 class="name">' . htmlspecialchars($comment['name'], ENT_QUOTES) . '</h3>
<span class="date">' . time_elapsed_string($comment['submit_date']) . '</span>
</div>
<p class="content">' . nl2br(htmlspecialchars($comment['content'], ENT_QUOTES)) . '</p>
<a class="reply_comment_btn" href="#" data-comment-id="' . $comment['id'] . '">Reply</a>
' . show_write_comment_form($comment['id']) . '
<div class="replies">
' . show_comments($comments, $comment['id']) . '
</div>
</div>
';
}
}
return $html;
}
function show_write_comment_form($parent_id = -1) {
$html = '
<div class="write_comment" data-comment-id="' . $parent_id . '">
<form>
<input name="parent_id" type="hidden" value="' . $parent_id . '">
<input name="name" type="text" placeholder="Your Name" required>
<textarea name="content" placeholder="Write your comment here..." required></textarea>
<button type="submit">Submit Comment</button>
</form>
</div>
';
return $html;
}
if (isset($_GET['page_id'])) {
if (isset($_POST['name'], $_POST['content'])) {
$stmt = $pdo->prepare('INSERT INTO comments (page_id, parent_id, name, content, submit_date) VALUES (?,?,?,?,NOW())');
$stmt->execute([ $_GET['page_id'], $_POST['parent_id'], $_POST['name'], $_POST['content'] ]);
exit('Your comment has been submitted Successfully');
}
$stmt = $pdo->prepare('SELECT * FROM comments WHERE page_id = ? ORDER BY submit_date DESC');
$stmt->execute([ $_GET['page_id'] ]);
$comments = $stmt->fetchAll(PDO::FETCH_ASSOC);
$stmt = $pdo->prepare('SELECT COUNT(*) AS total_comments FROM comments WHERE page_id = ?');
$stmt->execute([ $_GET['page_id'] ]);
$comments_info = $stmt->fetch(PDO::FETCH_ASSOC);
} else {
exit('No page ID specified!');
}
?>
<div class="comment_header">
<span class="total"><?=$comments_info['total_comments']?> comments</span>
<a href="#" class="write_comment_btn" data-comment-id="-1">Write Comment</a>
</div>
<?=show_write_comment_form()?>
<?=show_comments($comments)?>
Step by step
Solved in 3 steps with 6 images

Recommended textbooks for you
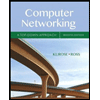
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
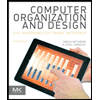
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
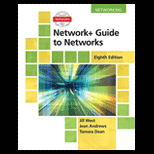
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
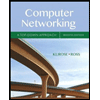
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
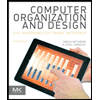
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
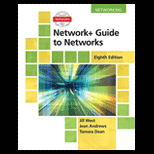
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
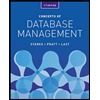
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
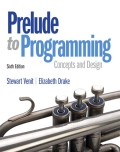
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
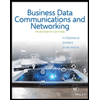
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY