Create the function Sum, which when called returns the sum of all the integers preceding and inclusive of the integer passed in its argument. For example Sum(4) === > 10 Show a screen shot of your code and its output.
Create the function Sum, which when called returns the sum of all the integers preceding and inclusive of the integer passed in its argument. For example Sum(4) === > 10 Show a screen shot of your code and its output.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:### Task
**Objective:**
Create the function `Sum`, which when called, returns the sum of all the integers preceding and inclusive of the integer passed in its argument.
**For Example:**
When the function `Sum(4)` is called, it should return `10`.
**Instructions:**
1. Define the function `Sum`.
2. Ensure that when called with an integer `n`, the function returns the sum of all integers from `1` to `n`.
3. Provide a screenshot of your code along with its output to demonstrate its functionality.
### Example Code:
```python
def Sum(n):
return sum(range(1, n + 1))
# Example Usage
result = Sum(4) # Expected output is 10
print(result) # Prints 10
```
**Explanation:**
- The function `Sum` uses Python’s built-in `sum()` function to calculate the sum of a range of numbers.
- `range(1, n + 1)` generates a sequence of numbers from 1 to `n` inclusive.
- The `sum()` function then adds up all these numbers and returns the result.
### Expected Output:
When you run the code above with the input `4`, the output should be `10`, as it calculates `1 + 2 + 3 + 4 = 10`.
### Screenshot:
Make sure to attach a screenshot of your code and its output as a demonstration.
This exercise is helpful in practicing the creation of simple functions and understanding the use of fundamental built-in functions in Python.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
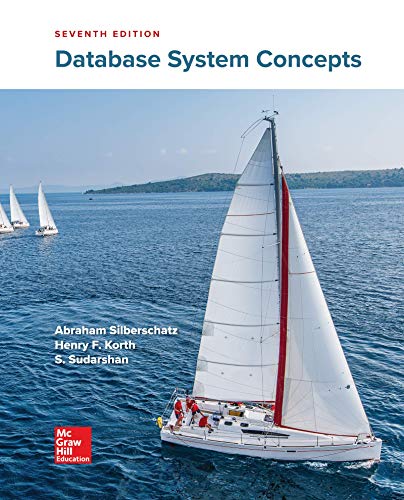
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
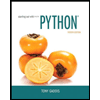
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
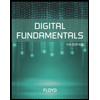
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
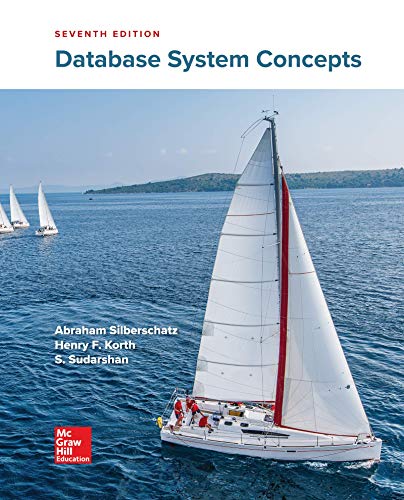
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
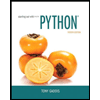
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
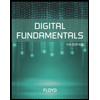
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
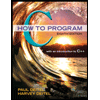
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
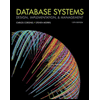
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
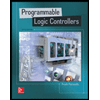
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education