Create pointers for a character, a string, an integer, a floating point number and for an array of 10 integers. For each pointer (5 of them), dynamically allocate the memory and then assign values as follows. You can use malloc and or calloc. Use your last name for the string (array of characters). Use 25 for the integer. The character should be an ‘E’. The floating point number should be 32.76 The array of integers should be the numerals 0 through 9. Print out values for all five dynamically allocated variables. Free all dynamically allocated memory. Output should look something like: Character: E Integer: 25 String: Doe Floating Point: 32.76 Array of Integers: 0 1 2 3 4 5 6 7 8 9
- You do not need getopt for this program as there are no arguments. Call the executable memact.
- Create pointers for a character, a string, an integer, a floating point number and for an array of 10 integers.
- For each pointer (5 of them), dynamically allocate the memory and then assign values as follows. You can use malloc and or calloc.
- Use your last name for the string (array of characters).
- Use 25 for the integer.
- The character should be an ‘E’.
- The floating point number should be 32.76
- The array of integers should be the numerals 0 through 9.
- Print out values for all five dynamically allocated variables.
- Free all dynamically allocated memory.
- Output should look something like:
Character: E
Integer: 25
String: Doe
Floating Point: 32.76
Array of Integers: 0 1 2 3 4 5 6 7 8 9

#include<stdio.h>
#include<stdlib.h>
int main()
{
//declaring given Five Types Of Pointers
char* ptr_str;
int* ptr_int;
char* ptr_char;
float* ptr_float;
int* ptr_int_arr;
//allocating the memory to ptr_str dynamically by using malloc()...
ptr_str = (char*)malloc(5*sizeof(char));
//setting the string "Sunny" to ptr_str...
ptr_str[0] = 'D';
ptr_str[1] = 'o';
ptr_str[2] = 'e';
//allocating the memory to ptr_int dynamically by using malloc()...
ptr_int=(int*)malloc(1*sizeof(int)); //set the ptr_int value to 25...
*ptr_int = 25;
//allocating the memory to ptr_char dynamically by using malloc()...
ptr_char = (char*)malloc(1*sizeof(char)); //set the Chracter to E...
*ptr_char = 'E';
//allocate the memory to ptr_float dynamically by using malloc()...
ptr_float= (float*)malloc(1*sizeof(float));
//set the value at ptr_float to 32.76...
*ptr_float = 32.76;
//allocating the memory to ptr_int_arr dynamically by using malloc()...
ptr_int_arr =(int*)malloc(10*sizeof(int));
//set values 0 to 9 in the int array
for(int i=0;i<10;i++)
{
ptr_int_arr[i] = i;
}
//print the all result in given format
printf("\nCharacter:%c",*ptr_char);
printf("\nInteger:%d",*ptr_int);
printf("\nString: ");
for(int i=0;i<5;i++)
{
printf("%c",ptr_str[i]);
}
printf("\nFloating point:%.2f",*ptr_float);
printf("\nArray of integer: ");
for(int i=0;i<10;i++)
{
printf("%d",ptr_int_arr[i]);
}
//Deallocating the five pointers using free() function
free(ptr_str);
free(ptr_int);
free(ptr_char);
free(ptr_float);
free(ptr_int_arr);
return 0;
}
Step by step
Solved in 2 steps with 1 images

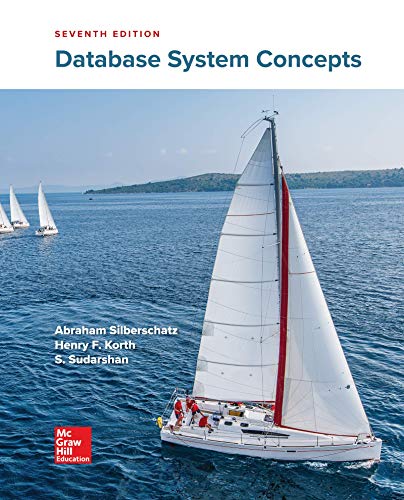
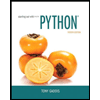
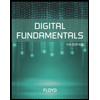
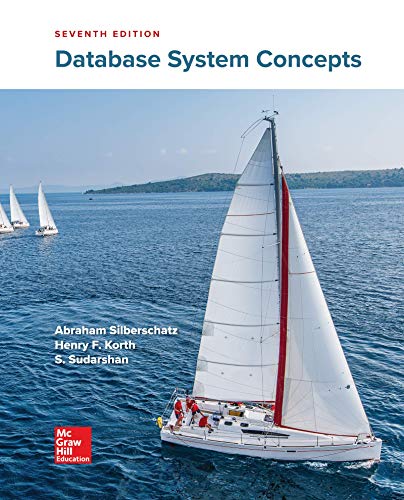
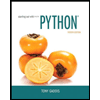
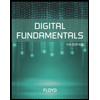
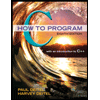
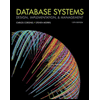
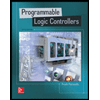