Create an application that allows user to build a Queue of Circle Elements . The Queue must be built using a Linked List (i.e., refer to Linked List Implementation, Figure 4.9 & 4.10 pp. 142 - 144 [uses C++ template; Figure 4.13 uses the template]). The application must be menu controlled and provide the following. • Allow insertion of a "Circle" object/structure in the Queue data structures. • Allow display of all elements from Queue data structure by Invoking a method/function "DisplayQueue" (uses "DeQueue" method). • Allow for deletion of the Queue
Create an application that allows user to build a Queue of Circle Elements . The Queue must be built using a Linked List (i.e., refer to Linked List Implementation, Figure 4.9 & 4.10 pp. 142 - 144 [uses C++ template; Figure 4.13 uses the template]). The application must be menu controlled and provide the following. • Allow insertion of a "Circle" object/structure in the Queue data structures. • Allow display of all elements from Queue data structure by Invoking a method/function "DisplayQueue" (uses "DeQueue" method). • Allow for deletion of the Queue
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I cannot figure out how to code this program. I attached an example of the code & where I went with it. Please help me understand this.
![Create an application that allows user to build a Queue of Circle Elements
. The Queue must be built using a Linked List (i.e., refer to Linked List
Implementation, Figure 4.9 & 4.10 pp. 142 - 144 [uses C++ template;
Figure 4.13 uses the template]). The application must be menu controlled
and provide the following.
• Allow insertion of a "Circle" object/structure in the Queue data
structures.
• Allow display of all elements from Queue data structure by Invoking a
method/function "DisplayQueue" (uses "DeQueue" method).
Allow for deletion of the Queue](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F35b1a49f-318f-4190-9cbc-933b3a48d3d2%2Fafd795c7-ca9a-4d04-8ee6-bffad3af93dd%2Flmf3oxa_processed.png&w=3840&q=75)
Transcribed Image Text:Create an application that allows user to build a Queue of Circle Elements
. The Queue must be built using a Linked List (i.e., refer to Linked List
Implementation, Figure 4.9 & 4.10 pp. 142 - 144 [uses C++ template;
Figure 4.13 uses the template]). The application must be menu controlled
and provide the following.
• Allow insertion of a "Circle" object/structure in the Queue data
structures.
• Allow display of all elements from Queue data structure by Invoking a
method/function "DisplayQueue" (uses "DeQueue" method).
Allow for deletion of the Queue
![#include <iostream>
#include <cstdlib>
using namespace std;
#include "genQueue.h"
int option (int percents []) {
register int i = 0, choice = rand () %100+1, perc;
for (perc = percents[0]; perc < choice; perc += percents[i+l], i++);
return i;
int main() {
int arrivals [] = {15,20,25,10,30};
int service []
= {0,0,0,10,5,10,10,0,15,25,10,15};
int clerks[] = {0,0,0,0}, numOfClerks = sizeof(clerks)/sizeof(int);
int customers, t, i, numofMinutes = 100, x;
double maxWait = 0.0, currWait = 0.0, thereIsLine = 0.0;
Queue<int> simulQ;
cout.precision (2);
for (t = 1; t <= numOfMinutes; t++) {
cout << "E = " << t;
for (i = 0; i < numOfClerks; i++)// after each minute subtract
if (clerks[i] < 60)
// at most 60 seconds from time
clerks (i] = 0;
// left to service the current
else clerks (i] -= 60;
// customer by clerk i;
customers
option (arr
als);
for (i = 0; i < customers; i++) {// enqueue all new customers
x = option (service) *10;
simulQ.enqueue (x);
// (or rather service time
// they require);
currWait += X;
}
// dequeue customers when clerks are available:
for (i = 0; i < numOfClerks && !simulQ.isEmpty (); )
if (clerks [i] < 60)
{
// assign more than one customer
x = simulQ.dequeue () ;
clerks (i] += X;
// to a clerk if service time
// is still below 60 sec;
currWait
-= x;
}
else i++;
if (!simulQ.isEmpty()) {
thereIsLine++;
cout << " wait = "
<< currWait/60.0;
if (maxWait < currWait)
maxWait = currWait;
}
else cout << " wait = 0;";
}
<« numofClerks << " clerks, there was a line "
<< thereIsLine/numofMinutes*100.0 << "% of the time;\n"
<< "maximum wait time was " << maxWait/60.0 << " min.";
cout << "\nFor "
return 0;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F35b1a49f-318f-4190-9cbc-933b3a48d3d2%2Fafd795c7-ca9a-4d04-8ee6-bffad3af93dd%2Fg2poyam_processed.png&w=3840&q=75)
Transcribed Image Text:#include <iostream>
#include <cstdlib>
using namespace std;
#include "genQueue.h"
int option (int percents []) {
register int i = 0, choice = rand () %100+1, perc;
for (perc = percents[0]; perc < choice; perc += percents[i+l], i++);
return i;
int main() {
int arrivals [] = {15,20,25,10,30};
int service []
= {0,0,0,10,5,10,10,0,15,25,10,15};
int clerks[] = {0,0,0,0}, numOfClerks = sizeof(clerks)/sizeof(int);
int customers, t, i, numofMinutes = 100, x;
double maxWait = 0.0, currWait = 0.0, thereIsLine = 0.0;
Queue<int> simulQ;
cout.precision (2);
for (t = 1; t <= numOfMinutes; t++) {
cout << "E = " << t;
for (i = 0; i < numOfClerks; i++)// after each minute subtract
if (clerks[i] < 60)
// at most 60 seconds from time
clerks (i] = 0;
// left to service the current
else clerks (i] -= 60;
// customer by clerk i;
customers
option (arr
als);
for (i = 0; i < customers; i++) {// enqueue all new customers
x = option (service) *10;
simulQ.enqueue (x);
// (or rather service time
// they require);
currWait += X;
}
// dequeue customers when clerks are available:
for (i = 0; i < numOfClerks && !simulQ.isEmpty (); )
if (clerks [i] < 60)
{
// assign more than one customer
x = simulQ.dequeue () ;
clerks (i] += X;
// to a clerk if service time
// is still below 60 sec;
currWait
-= x;
}
else i++;
if (!simulQ.isEmpty()) {
thereIsLine++;
cout << " wait = "
<< currWait/60.0;
if (maxWait < currWait)
maxWait = currWait;
}
else cout << " wait = 0;";
}
<« numofClerks << " clerks, there was a line "
<< thereIsLine/numofMinutes*100.0 << "% of the time;\n"
<< "maximum wait time was " << maxWait/60.0 << " min.";
cout << "\nFor "
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
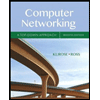
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
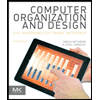
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
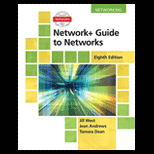
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
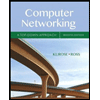
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
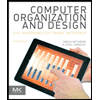
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
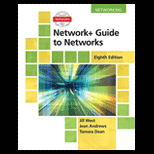
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
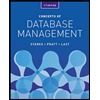
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
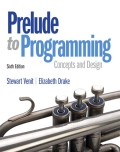
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
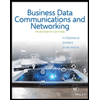
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY