Create a Python Code Since an electronic circuit is the interconnection of several components, it might not be surprising that they can be represented as graphs which allow our computers to simulate the behavior of the circuit. A sample resistor network has its corresponding netlist representation used in "ngspice" (see the image). Description: This problem focuses on properly parsing the input. To test this, your code should be able to properly store each input resistor and store them in a data structure and representation of your choice. To verify this, your code should be able to identify which resistors are in series and which resistors are in parallel. In the context of a graph, two resistors can be considered in series if there are no branches in the path formed between the resistors. On the other hand, two resistors can be considered in parallel if they are connected to the same two nodes. Input Format: The first line of the input consists of 2 integers N and Q which is the total number of resistors and total number of queries, respectively. The next N lines will consist of 3 strings and an integer R, each separated by a space. The first string is the resistor name, the second and third strings are the node names where the resistor terminals are connected, and R is its resistance value. Finally, there will be Q lines with two strings each which will each be the name of a resistor. Output Format: The output is expected to have Q lines which correspond to the answer to each query. If the resistors in the first query are in series, the first line of the output should be SERIES. If they are parallel, the expected output is PARALLEL. If they are neither in series or parallel, the expected output is NEITHER. Constraints: ● Resistors in the queries are guaranteed to exist. ● 1 ≤ N, Q ≤ 1000 and 0 < R ≤ (109) Testcases: Sample Input 0 (see the image) 5 4 R1 Vdd a 1000 R2 a GND 1000 R3 Vdd b 1000 R4 b GND 1000 R5 b GND 1000 R1 R2 R2 R3 R3 R4 R4 R5 Sample Output 0 SERIES NEITHER NEITHER PARALLEL Sample Input 1 3 6 R1 Vdd GND 1000 R2 Vdd GND 500 R3 Vdd GND 200 R1 R2 R1 R3 R2 R3 R2 R1 R3 R1 R3 R2 Sample Output 1 PARALLEL PARALLEL PARALLEL PARALLEL PARALLEL PARALLEL Sample Input 2 5 10 R1 Vdd a 10000 R2 a b 2000 R3 b c 300 R4 c d 40 R5 d GND 5 R1 R2 R1 R3 R1 R4 R1 R5 R2 R3 R2 R4 R2 R5 R3 R4 R3 R5 R4 R5 Sample Output 2 SERIES SERIES SERIES SERIES SERIES SERIES SERIES SERIES SERIES SERIES
Create a Python Code
Since an electronic circuit is the interconnection of several components, it might not be surprising that they can be represented as graphs which allow our computers to simulate the behavior of the circuit. A sample resistor network has its corresponding netlist representation used in "ngspice" (see the image).
Description:
This problem focuses on properly parsing the input. To test this, your code should be able to properly store each input resistor and store them in a data structure and representation of your choice. To verify this, your code should be able to identify which resistors are in series and which resistors are in parallel. In the context of a graph, two resistors can be considered in series if there are no branches in the path formed between the resistors. On the other hand, two resistors can be considered in parallel if they are connected to the same two nodes.
Input Format:
The first line of the input consists of 2 integers N and Q which is the total number of resistors and total number of queries, respectively. The next N lines will consist of 3 strings and an integer R, each separated by a space. The first string is the resistor name, the second and third strings are the node names where the resistor terminals are connected, and R is its resistance value. Finally, there will be Q lines with two strings each which will each be the name of a resistor.
Output Format:
The output is expected to have Q lines which correspond to the answer to each query. If the resistors in the first query are in series, the first line of the output should be SERIES. If they are parallel, the expected output is PARALLEL. If they are neither in series or parallel, the expected output is NEITHER.
Constraints:
● Resistors in the queries are guaranteed to exist.
● 1 ≤ N, Q ≤ 1000 and 0 < R ≤ (109)
Testcases:
Sample Input 0 (see the image) 5 4 Sample Output 0 SERIES |
Sample Input 1 3 6 Sample Output 1 PARALLEL |
Sample Input 2 5 10 Sample Output 2 SERIES |


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

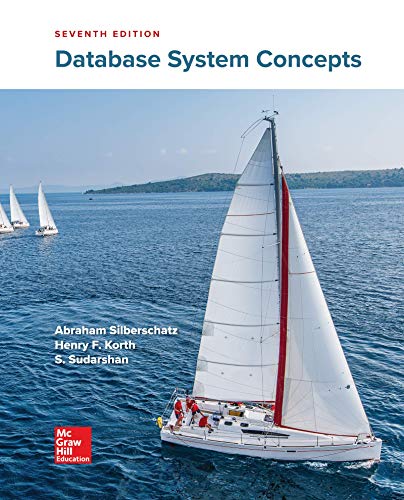
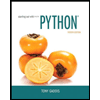
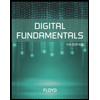
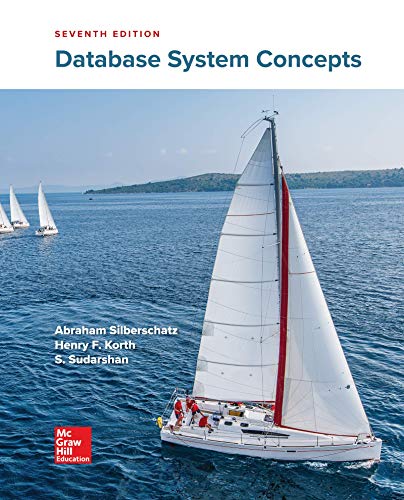
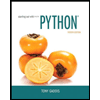
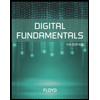
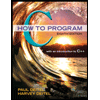
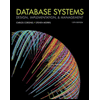
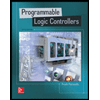