Create a Flowchart:
Create a Flowchart:
# User defined function for logic OR
# The function takes two parameters and returns a single int
def OR(a: int, b: int)->int:
# If a is equal to 1 return 1
if a == 1 :
return 1
# If b is equal to 1 return 1
elif b == 1 :
return 1
# If a and b is equal to 0 return 0
else :
return 0
# User defined function for logic NOR
# The function takes two parameters and returns a single int
def NOR(a: int, b: int)->int:
# If a is equal to 0 and b is also equal to 0 return 1
if a == 0 and b == 0 :
return 1
# If a is equal to 0 and b is equal to 1 return 0
elif a == 0 and b == 1 :
return 0
# If a is equal to 1 and b is also equal to 0 return 0
elif a == 1 and b == 0 :
return 0
# If a is equal to 1 and b is also equal to 1 return 0
elif a == 1 and b == 1 :
return 0
# User defined function for logic AND
# The function takes two parameters and returns a single int
def AND(a: int, b: int)->int:
# Return 1 if both a and b are equal to 1
if a == 1 and b == 1 :
return 1
# Return 0 otherwise
else :
return 0
# User defined function for defining a logical circuit
# The function takes three parameters and returns a single int
def logic_circuit(A: int, B: int, C: int)->int:
# Calling above defined functions with
# parameters passed to them
# The function calls simulate a logic circuit
# The result is stored in a variable Q
# The value in Q is returned
Q = OR(NOR(A, B), AND(B, C))
return Q
# User inputs for bits A, B and C
while(True): #infinte loop
A = int(input("Enter binary input For A: ")); #ask for input
if(A==0 or A==1): #if input is 0 or 1
break; #exit from while loop
while(True): #infinte loop
B = int(input("Enter binary input For B: ")); #ask For input
if(B==0 or B==1): #if input is 0 or 1
break; #exit from while loop
while(True): #infinte loop
C = int(input("Enter binary input For C: ")); #ask for input
if(C==0 or C==1): #if input is 0 or 1
break; #exit from while loop
# Calling function logic_circuit with A, B and C
# passed as parameters
# The result is stored in a variable Q
# The value of Q is returned
Q = logic_circuit(A, B, C)
print("Output: ", Q)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

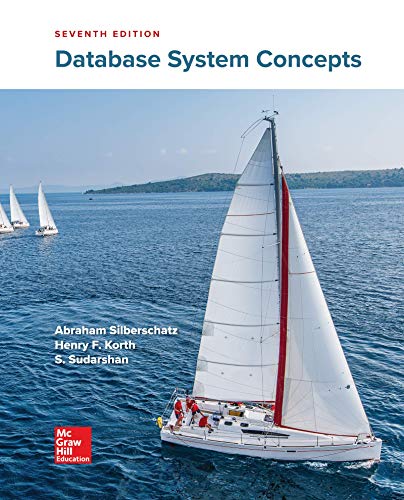
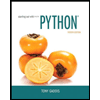
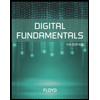
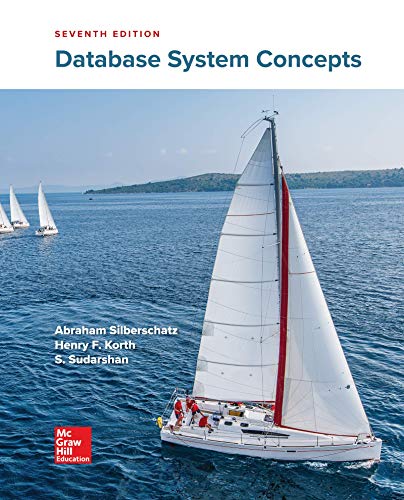
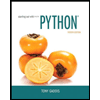
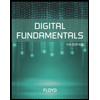
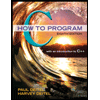
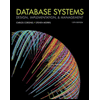
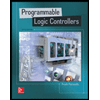