Create a file named inventory.py. a. Define a class named Inventory. a. Define a member function named_init_ to meet the following requirements: a. Take a self object as a positional parameter. b. Take a filename string as a keyword parameter. c. Define a self.data instance variable to hold the dictionary. d. Open the filename JSON file, if it exists and contains data, assign the JSON deserialized data (a dictionary) to self.data, otherwise cleanly handle the error. b. Define a member function named add_item to meet the following requirements: a. Take a self object as a positional parameter. b. Take a barcode string as a keyword parameter. Return an integer value of 109 if the value of the barcode does not meet the required format identified in the dictionary structure above. Return an integer value of 101 if the barcode already exists in the dictionary. c. Take a description string as a keyword parameter. d. Add the item to the dictionary by setting the dictionary key to barcode and the value to the list made up of the description and the quantity of zero. e. Write the updated dictionary to the filename provided in the __init___ function. f. Return an integer value of 100 for a successful add. c. Define a member function named modify_description to meet the following requirements: a. Take a self object as a positional parameter. b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the barcode does not exist in the dictionary. c. Take a description string as a keyword parameter. d. Update the item in the dictionary by setting the dictionary key to barcode and the value to the list made up of the description and the existing quantity (i.e the quantity should not change). e. Write the updated dictionary to the filename provided in the __init___ function. f. Return an integer value of 100 for a successful add. d. Define a member function named add_qty to meet the following requirements: a. Take a self object as a positional parameter. b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the barcode does not exist in the dictionary. c. Take a qty integer as a keyword parameter. Return an integer value of 108 if the value of the qty does not meet the required format identified in the dictionary structure above (i.e. an integer). d. Update the item in the dictionary by setting the dictionary key to barcode and the value to the list made up of the existing description and the existing quantity plus qty. e. Write the updated dictionary to the filename provided in the __init___ function. f. Return an integer value of 100 for a successful add. e. Define a member function named remove_qty to meet the following requirements: a. Take a self object as a positional parameter. b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the barcode does not exist in the dictionary. c. Take a qty integer as a keyword parameter. Return an integer value of 108 if the value of the qty does not meet the required format identified in the dictionary structure above (i.e. an integer). d. Return an integer value of 103 if the value of the qty is greater than the existing quantity in inventory. e. Update the item in the dictionary by setting the dictionary key to barcode and the value to the list made up of the existing description and the existing quantity minus qty. f. Write the updated dictionary to the filename provided in the __init____ function. g. Return an integer value of 100 for a successful add. f. Define a member function named get_inventory to meet the following requirements: a. Take a self object as a positional parameter. b. Return a formatted string that contains all the items in the dictionary, each on a separate line. The format should allow 8 characters for the barcode, 20 characters for the description, and 5 characters for the quantity, indicated as follows (note: the top line is for illustrative purposes only and should not be included in the return string): |--8--||----20▬▬▬▬▬▬▬▬▬▬▬▬||-5-| BC9001 Paper BC9034 Notebook. BC9026 Computer BC9072 Pencil 173 84 7 21
Create a file named inventory.py. a. Define a class named Inventory. a. Define a member function named_init_ to meet the following requirements: a. Take a self object as a positional parameter. b. Take a filename string as a keyword parameter. c. Define a self.data instance variable to hold the dictionary. d. Open the filename JSON file, if it exists and contains data, assign the JSON deserialized data (a dictionary) to self.data, otherwise cleanly handle the error. b. Define a member function named add_item to meet the following requirements: a. Take a self object as a positional parameter. b. Take a barcode string as a keyword parameter. Return an integer value of 109 if the value of the barcode does not meet the required format identified in the dictionary structure above. Return an integer value of 101 if the barcode already exists in the dictionary. c. Take a description string as a keyword parameter. d. Add the item to the dictionary by setting the dictionary key to barcode and the value to the list made up of the description and the quantity of zero. e. Write the updated dictionary to the filename provided in the __init___ function. f. Return an integer value of 100 for a successful add. c. Define a member function named modify_description to meet the following requirements: a. Take a self object as a positional parameter. b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the barcode does not exist in the dictionary. c. Take a description string as a keyword parameter. d. Update the item in the dictionary by setting the dictionary key to barcode and the value to the list made up of the description and the existing quantity (i.e the quantity should not change). e. Write the updated dictionary to the filename provided in the __init___ function. f. Return an integer value of 100 for a successful add. d. Define a member function named add_qty to meet the following requirements: a. Take a self object as a positional parameter. b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the barcode does not exist in the dictionary. c. Take a qty integer as a keyword parameter. Return an integer value of 108 if the value of the qty does not meet the required format identified in the dictionary structure above (i.e. an integer). d. Update the item in the dictionary by setting the dictionary key to barcode and the value to the list made up of the existing description and the existing quantity plus qty. e. Write the updated dictionary to the filename provided in the __init___ function. f. Return an integer value of 100 for a successful add. e. Define a member function named remove_qty to meet the following requirements: a. Take a self object as a positional parameter. b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the barcode does not exist in the dictionary. c. Take a qty integer as a keyword parameter. Return an integer value of 108 if the value of the qty does not meet the required format identified in the dictionary structure above (i.e. an integer). d. Return an integer value of 103 if the value of the qty is greater than the existing quantity in inventory. e. Update the item in the dictionary by setting the dictionary key to barcode and the value to the list made up of the existing description and the existing quantity minus qty. f. Write the updated dictionary to the filename provided in the __init____ function. g. Return an integer value of 100 for a successful add. f. Define a member function named get_inventory to meet the following requirements: a. Take a self object as a positional parameter. b. Return a formatted string that contains all the items in the dictionary, each on a separate line. The format should allow 8 characters for the barcode, 20 characters for the description, and 5 characters for the quantity, indicated as follows (note: the top line is for illustrative purposes only and should not be included in the return string): |--8--||----20▬▬▬▬▬▬▬▬▬▬▬▬||-5-| BC9001 Paper BC9034 Notebook. BC9026 Computer BC9072 Pencil 173 84 7 21
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help writing this is python please

Transcribed Image Text:Create a file named inventory.py.
a. Define a class named Inventory.
a. Define a member function named_init_ to meet the following requirements:
a. Take a self object as a positional parameter.
b. Take a filename string as a keyword parameter.
c. Define a self.data instance variable to hold the dictionary.
d. Open the filename JSON file, if it exists and contains data, assign the JSON deserialized
data (a dictionary) to self.data, otherwise cleanly handle the error.
b. Define a member function named add_item to meet the following requirements:
a. Take a self object as a positional parameter.
b. Take a barcode string as a keyword parameter. Return an integer value of 109 if the value
of the barcode does not meet the required format identified in the dictionary structure
above. Return an integer value of 101 if the barcode already exists in the dictionary.
c. Take a description string as a keyword parameter.
d. Add the item to the dictionary by setting the dictionary key to barcode and the value to the
list made up of the description and the quantity of zero.
e. Write the updated dictionary to the filename provided in the __init_ function.
f. Return an integer value of 100 for a successful add.
c. Define a member function named modify_description to meet the following requirements:
a. Take a self object as a positional parameter.
b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the
barcode does not exist in the dictionary.
c. Take a description string as a keyword parameter.
d. Update the item in the dictionary by setting the dictionary key to barcode and the value to
the list made up of the description and the existing quantity (i.e the quantity should not
change).
e. Write the updated dictionary to the filename provided in the __init___ function.
f. Return an integer value of 100 for a successful add.
d. Define a member function named add_qty to meet the following requirements:
a. Take a self object as a positional parameter.
b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the
barcode does not exist in the dictionary.
c. Take a qty integer as a keyword parameter. Return an integer value of 108 if the value of
the qty does not meet the required format identified in the dictionary structure above (i.e. an
integer).
d. Update the item in the dictionary by setting the dictionary key to barcode and the value to
the list made up of the existing description and the existing quantity plus qty.
e. Write the updated dictionary to the filename provided in the __init___ function.
f. Return an integer value of 100 for a successful add.
e. Define a member function named remove_qty to meet the following requirements:
a. Take a self object as a positional parameter.
b. Take a barcode string as a keyword parameter. Return an integer value of 102 if the
barcode does not exist in the dictionary.
c. Take a qty integer as a keyword parameter. Return an integer value of 108 if the value of
the qty does not meet the required format identified in the dictionary structure above (i.e. an
integer).
d. Return an integer value of 103 if the value of the qty is greater than the existing quantity in
inventory.
e. Update the item in the dictionary by setting the dictionary key to barcode and the value to
the list made up of the existing description and the existing quantity minus qty .
f. Write the updated dictionary to the filename provided in the __init___ function.
g. Return an integer value of 100 for a successful add.
f. Define a member function named get_inventory to meet the following requirements:
a. Take a self object as a positional parameter.
b. Return a formatted string that contains all the items in the dictionary, each on a separate
line. The format should allow 8 characters for the barcode, 20 characters for the
description, and 5 characters for the quantity, indicated as follows (note: the top line is for
illustrative purposes only and should not be included in the return string):
|--8--||---- 20-
-20---- -||-5-1
173
84
7
21
BC9001 Paper
BC9034 Notebook
BC9026 Computer
BC9072 Pencil
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
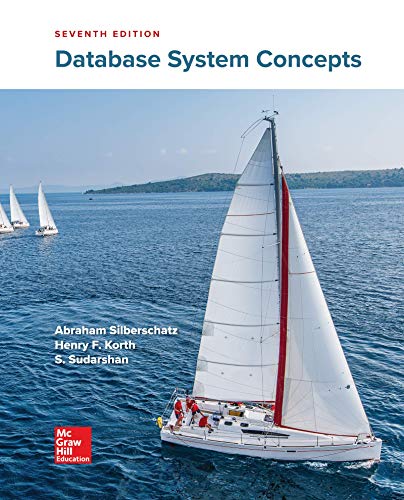
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
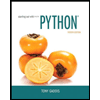
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
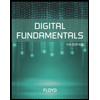
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
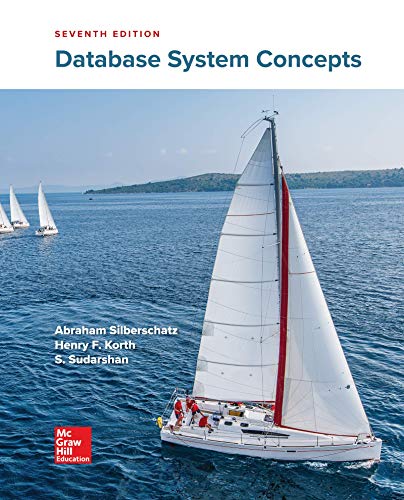
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
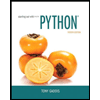
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
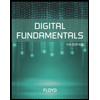
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
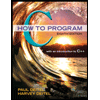
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
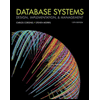
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
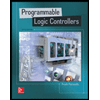
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education