Create a class named Caesar Cipher that has the following. • Write a public static method named encrypt that takes a String s and an int das parameters and returns a String. The Caesar cipher is one of the earliest known and simplest ciphers. It is a type of substitution cipher in which each letter in the text is "shifted" a certain number of places down the alphabet. For example, with a shift of 1, a would become b, b would become c, and so on. The method is named after Julius Caesar, who used it to communicate with his generals. • The encrypt method should take the String parameters and encrypt it using the Caesar cipher with a shift of d. Note that there is no upper limit (other than the max value of the int primitive type) on the value for d. You can assume that there is no punctuation and that the String parameter will always contain lower case letters. • Additional rules: You may use one conditional (if) statements, and it can have only one (else) statement. Create a test class named CaesarCipherTest that contains the main method and prints the following method outputs in the following order, with a blank line between each encryption. Method Output Method Call CaesarCipher.encrypt("abc", 1) CaesarCipher.encrypt("abc", 25) CaesarCipher.encrypt("abc", 50) CaesarCipher.encrypt("attack at dawn", 17) Shift: 1 abc encrypted is bcd Shift: 25 abc encrypted is zab Shift: 50 abc encrypted is yza Shift: 17 attack at dawn encrypted is rkkrtb rk urne • Write a public static method named decrypt that takes a String s and an int d as parameters and returns a String. • The decrypt method should take the String parameters and decrypt it using the Caesar cipher with a shift back of d. Note that there is no upper limit (other than the max value of the int primitive type) on the value for d. You can assume that there is no punctuation and that
Create a class named Caesar Cipher that has the following. • Write a public static method named encrypt that takes a String s and an int das parameters and returns a String. The Caesar cipher is one of the earliest known and simplest ciphers. It is a type of substitution cipher in which each letter in the text is "shifted" a certain number of places down the alphabet. For example, with a shift of 1, a would become b, b would become c, and so on. The method is named after Julius Caesar, who used it to communicate with his generals. • The encrypt method should take the String parameters and encrypt it using the Caesar cipher with a shift of d. Note that there is no upper limit (other than the max value of the int primitive type) on the value for d. You can assume that there is no punctuation and that the String parameter will always contain lower case letters. • Additional rules: You may use one conditional (if) statements, and it can have only one (else) statement. Create a test class named CaesarCipherTest that contains the main method and prints the following method outputs in the following order, with a blank line between each encryption. Method Output Method Call CaesarCipher.encrypt("abc", 1) CaesarCipher.encrypt("abc", 25) CaesarCipher.encrypt("abc", 50) CaesarCipher.encrypt("attack at dawn", 17) Shift: 1 abc encrypted is bcd Shift: 25 abc encrypted is zab Shift: 50 abc encrypted is yza Shift: 17 attack at dawn encrypted is rkkrtb rk urne • Write a public static method named decrypt that takes a String s and an int d as parameters and returns a String. • The decrypt method should take the String parameters and decrypt it using the Caesar cipher with a shift back of d. Note that there is no upper limit (other than the max value of the int primitive type) on the value for d. You can assume that there is no punctuation and that
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:●
Additional rules: You may use one conditional (if) statements, and it can have only one (else)
statement.
In your CaesarCipherTest class, print out the following method outputs in the following order
with a blank space in between each decryption as well. Check the following usage examples to
check your code.
Method Call
CaesarCipher.decrypt("bcd", 1)
CaesarCipher.decrypt("zab", 25)
CaesarCipher.decrypt("yza", 50)
CaesarCipher.decrypt("rkkrtb rk urne", 17)
Method Output
Shift: 1
bcd decrypted is abc
Shift: 25
zab decrypted is abc
Shift: 50
yza decrypted is abc.
Shift: 17
rkkrtb rk urne decrypted is attack at dawn

Transcribed Image Text:Practice Problem #2
Create a class named CaesarCipher that has the following.
Write a public static method named encrypt that takes a String s and an int das
parameters and returns a String.
• The Caesar cipher is one of the earliest known and simplest ciphers. It is a type of substitution
cipher in which each letter in the text is "shifted" a certain number of places down the
alphabet. For example, with a shift of 1, a would become b, b would become c, and so on. The
method is named after Julius Caesar, who used it to communicate with his generals.
• The encrypt method should take the String parameters and encrypt it using the Caesar
cipher with a shift of d. Note that there is no upper limit (other than the max value of the int
primitive type) on the value for d. You can assume that there is no punctuation and that the
String parameter will always contain lower case letters.
Additional rules: You may use one conditional (if) statements, and it can have only one (else)
statement.
• Create a test class named Caesar CipherTest that contains the main method and prints the
following method outputs in the following order, with a blank line between each encryption.
Method Output
Method Call
CaesarCipher.encrypt("abc", 1)
CaesarCipher.encrypt("abc", 25)
CaesarCipher.encrypt("abc", 50)
CaesarCipher.encrypt("attack at dawn", 17)
Shift: 1
abc encrypted is bcd
Shift: 25
abc encrypted is zab
Shift: 50
abc encrypted is yza
Shift: 17
attack at dawn encrypted is rkkrtb rk urne
Write a public static method named decrypt that takes a Strings and an int d as
parameters and returns a String.
• The decrypt method should take the String parameters and decrypt it using the Caesar
cipher with a shift back of d. Note that there is no upper limit (other than the max value of the
int primitive type) on the value for d. You can assume that there is no punctuation and that
the String parameter will always contain lower case letters.
Expert Solution

Step 1
To implement Caesar cipher code in which each letter in the text is "shifted" to a certain number of places down the alphabet.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
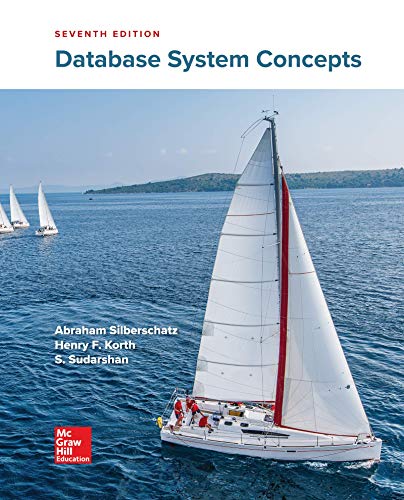
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
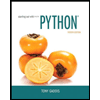
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
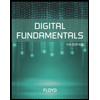
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
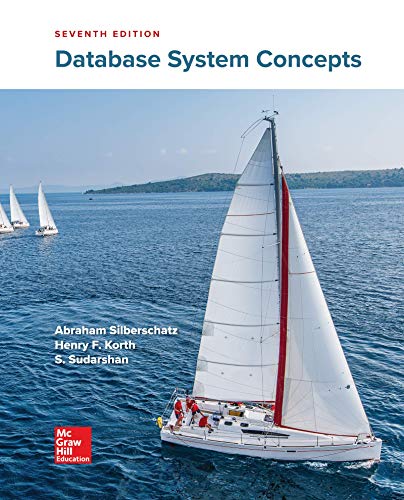
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
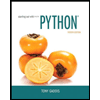
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
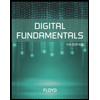
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
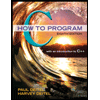
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
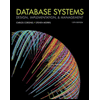
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
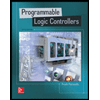
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education