Create a class called Carrier that has the following attributes: Carrier() Carrier(numberOfSpaceFighter Docks, numberOfDrill Docks, cargoholdSize) Carrier spaceFighterDocks[] SpaceFighter drillDocks[] - Drill cargohold[] - String deployFighter() SpaceFighter dockFighter(SpaceFighter) - Boolean deployDrill() Drill dockDrill(Drill) - Boolean addItemToCargohold(String) - Boolean removeltemFromCargohold(String) - Boolean toString() - String Note: The deploy and dock method just add or remove things from the corresponding arrays of SpaceFighter and Drill. When you dock something, if the array full, return false; otherwise true. Similarly for the Cargo methods.
Create a class called Carrier that has the following attributes: Carrier() Carrier(numberOfSpaceFighter Docks, numberOfDrill Docks, cargoholdSize) Carrier spaceFighterDocks[] SpaceFighter drillDocks[] - Drill cargohold[] - String deployFighter() SpaceFighter dockFighter(SpaceFighter) - Boolean deployDrill() Drill dockDrill(Drill) - Boolean addItemToCargohold(String) - Boolean removeltemFromCargohold(String) - Boolean toString() - String Note: The deploy and dock method just add or remove things from the corresponding arrays of SpaceFighter and Drill. When you dock something, if the array full, return false; otherwise true. Similarly for the Cargo methods.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
26
![### Class: Carrier
The `Carrier` class in this educational module encompasses several attributes and methods related to maintaining and manipulating space fighters, drills, and a cargo hold.
#### Attributes:
1. **spaceFighterDocks[]**: `SpaceFighter`
2. **drillDocks[]**: `Drill`
3. **cargohold[]**: `String`
#### Constructors:
- **Carrier()**: Default constructor.
- **Carrier(numberOfSpaceFighterDocks, numberOfDrillDocks, cargoholdSize)**: Parameterized constructor to initialize the Carrier with specified sizes for the docks and cargo hold.
#### Methods:
1. **deployFighter()**: `SpaceFighter`
- Deploys a space fighter from the carrier.
2. **dockFighter(SpaceFighter)**: `Boolean`
- Docks a space fighter into the carrier. Returns `true` if successful, `false` if the dock is full.
3. **deployDrill()**: `Drill`
- Deploys a drill from the carrier.
4. **dockDrill(Drill)**: `Boolean`
- Docks a drill into the carrier. Returns `true` if successful, `false` if the dock is full.
5. **addItemToCargohold(String)**: `Boolean`
- Adds an item to the cargohold. Returns `true` if successful, `false` if the cargo hold is full.
6. **removeItemFromCargohold(String)**: `Boolean`
- Removes an item from the cargohold. Returns `true` if successful, `false` if the item does not exist.
7. **toString()**: `String`
- Converts the carrier's current state to a string representation.
#### Note:
- The `deploy` and `dock` methods manage the respective arrays for `SpaceFighter` and `Drill`. When docking, if the respective array is full, the method returns `false`; otherwise, it returns `true`. Similarly, this logic is applied to adding and removing items from the cargo hold.
#### Explanation:
The depiction in the image includes fields and methods within a tabular structure that delineates the components of the `Carrier` class. The constructors and methods offer a comprehensive way to handle docking and deploying space assets and managing cargo in the system.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F676c8c41-ef82-4331-a0d2-fcd67f369270%2Ff92003a9-998f-40ce-b132-34ff1955c564%2F4qz9d9_processed.png&w=3840&q=75)
Transcribed Image Text:### Class: Carrier
The `Carrier` class in this educational module encompasses several attributes and methods related to maintaining and manipulating space fighters, drills, and a cargo hold.
#### Attributes:
1. **spaceFighterDocks[]**: `SpaceFighter`
2. **drillDocks[]**: `Drill`
3. **cargohold[]**: `String`
#### Constructors:
- **Carrier()**: Default constructor.
- **Carrier(numberOfSpaceFighterDocks, numberOfDrillDocks, cargoholdSize)**: Parameterized constructor to initialize the Carrier with specified sizes for the docks and cargo hold.
#### Methods:
1. **deployFighter()**: `SpaceFighter`
- Deploys a space fighter from the carrier.
2. **dockFighter(SpaceFighter)**: `Boolean`
- Docks a space fighter into the carrier. Returns `true` if successful, `false` if the dock is full.
3. **deployDrill()**: `Drill`
- Deploys a drill from the carrier.
4. **dockDrill(Drill)**: `Boolean`
- Docks a drill into the carrier. Returns `true` if successful, `false` if the dock is full.
5. **addItemToCargohold(String)**: `Boolean`
- Adds an item to the cargohold. Returns `true` if successful, `false` if the cargo hold is full.
6. **removeItemFromCargohold(String)**: `Boolean`
- Removes an item from the cargohold. Returns `true` if successful, `false` if the item does not exist.
7. **toString()**: `String`
- Converts the carrier's current state to a string representation.
#### Note:
- The `deploy` and `dock` methods manage the respective arrays for `SpaceFighter` and `Drill`. When docking, if the respective array is full, the method returns `false`; otherwise, it returns `true`. Similarly, this logic is applied to adding and removing items from the cargo hold.
#### Explanation:
The depiction in the image includes fields and methods within a tabular structure that delineates the components of the `Carrier` class. The constructors and methods offer a comprehensive way to handle docking and deploying space assets and managing cargo in the system.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
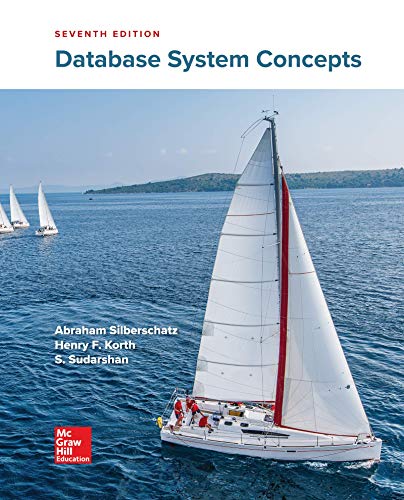
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
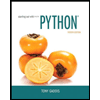
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
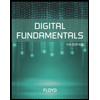
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
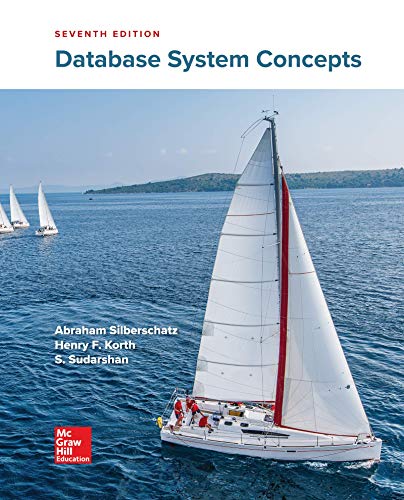
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
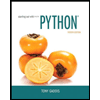
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
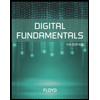
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
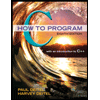
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
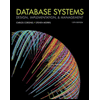
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
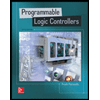
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education