Create a class ArrayQueue ● default constructor isEmpty() count() peek() ● ● ● ● Instructions - do an array-based implementation of a queue dequeue() enqueue() Create a class MCCAirport ● ● final double LANDING_TIME = 3; final double TAKE_OFF_TIME = 2; final double LANDING_RATE = 10; final double TAKE_OFF_RATE= 10; final int ITERATIONS = 1440; . //use a random generator import java.util.Random; Random generator = new Random(System.current TimeMillis( )); Print out the following: values should be 6 places to the right of the decimal Average landing queue length: Average take off queue length: Average landing queue time: Average take off queue time:
Create a class ArrayQueue ● default constructor isEmpty() count() peek() ● ● ● ● Instructions - do an array-based implementation of a queue dequeue() enqueue() Create a class MCCAirport ● ● final double LANDING_TIME = 3; final double TAKE_OFF_TIME = 2; final double LANDING_RATE = 10; final double TAKE_OFF_RATE= 10; final int ITERATIONS = 1440; . //use a random generator import java.util.Random; Random generator = new Random(System.current TimeMillis( )); Print out the following: values should be 6 places to the right of the decimal Average landing queue length: Average take off queue length: Average landing queue time: Average take off queue time:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
The first image is the main question, the second image is the instructions for the

Transcribed Image Text:---
## Instructions: Implementing an Array-Based Queue
### Task 1: Create a class `ArrayQueue<T>`
The `ArrayQueue<T>` class should include the following methods:
- **default constructor**: Initializes the queue.
- **isEmpty()**: Checks if the queue is empty.
- **count()**: Returns the number of elements in the queue.
- **peek()**: Returns the element at the front of the queue without removing it.
- **dequeue()**: Removes and returns the element at the front of the queue.
- **enqueue(T item)**: Adds an item to the back of the queue.
### Task 2: Create a class `MCCAirport`
The `MCCAirport` class should have the following constants:
- **final double LANDING_TIME = 3**: Average time for a landing (in some time unit).
- **final double TAKE_OFF_TIME = 2**: Average time for a take-off.
- **final double LANDING_RATE = 10**: Rate of landings (landings per time unit).
- **final double TAKE_OFF_RATE = 10**: Rate of take-offs.
A final integer should be defined:
- **final int ITERATIONS = 1440**: Total number of iterations for the simulation.
You should also import and use a random number generator for simulation purposes:
```java
import java.util.Random;
Random generator = new Random(System.currentTimeMillis());
```
### Output Requirements
At the end of the simulation, print out the following statistics:
- **Average landing queue length:**
- **Average take-off queue length:**
- **Average landing queue time:**
- **Average take-off queue time:**
These values should be displayed with 6 decimal places of precision.
---

Transcribed Image Text:### Simulating Airport Runway Operations at Middlesex County College Airport
Suppose that Middlesex County College Airport has one runway, which each airplane takes LandingTime minutes to land and TakeOffTime minutes to take off, and that on average, TakeOffRate planes take off and LandingRate planes land each hour.
Assume that the planes arrive at random instants of time. Delays make the assumption of randomness quite reasonable.
---
There are two types of queues:
1. A queue of airplanes waiting to land.
2. A queue of airplanes waiting to take off.
Since it is more expensive to keep a plane airborne than to have one waiting on the ground, we assume that airplanes in the landing queue have priority over those in the takeoff queue.
### Program Simulation
Write a Java program to simulate MCC Airport's operation. You might assume a simulated clock that advances in one-minute intervals. For each minute, generate two random numbers. If the first is less than LandingRate/60.0, a "landing arrival" has occurred and is added to the landing queue. If the second is less than TakeOffRate/60.0, a "takeoff arrival" has occurred and is added to the takeoff queue.
Next, check whether the runway is free. If it is free, first check whether the landing queue is nonempty, and if so, allow the first airplane to land. Otherwise, consider the takeoff queue.
### Considerations:
- Have the program calculate the average queue length and the average time that an airplane spends in a queue.
- You should also consider the effect of varying arrival and departure rates to simulate the prime and slack times of the day, or what happens if the amount of time to land or take off is increased or decreased.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
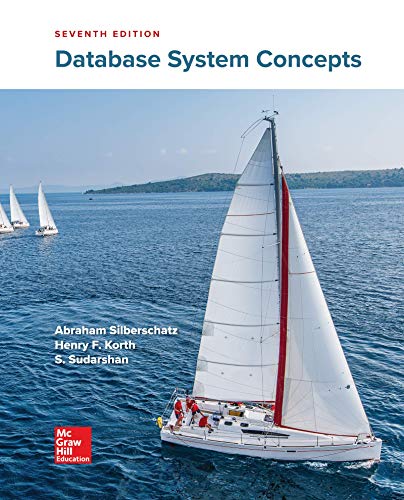
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
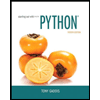
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
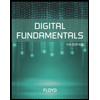
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
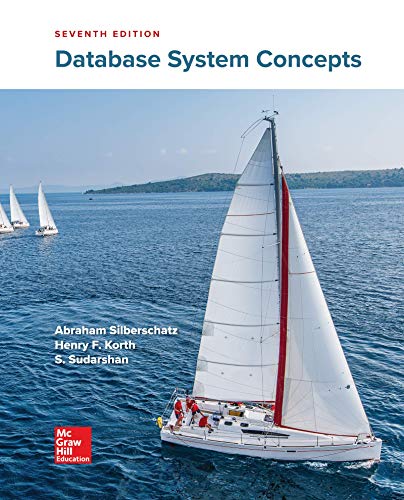
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
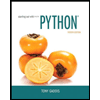
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
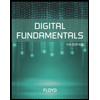
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
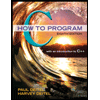
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
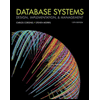
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
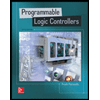
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education